How do I create an empty array and then append to it in NumPy?
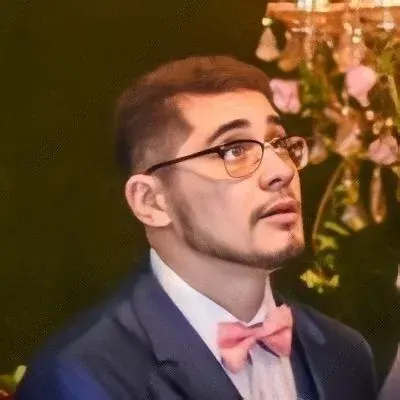
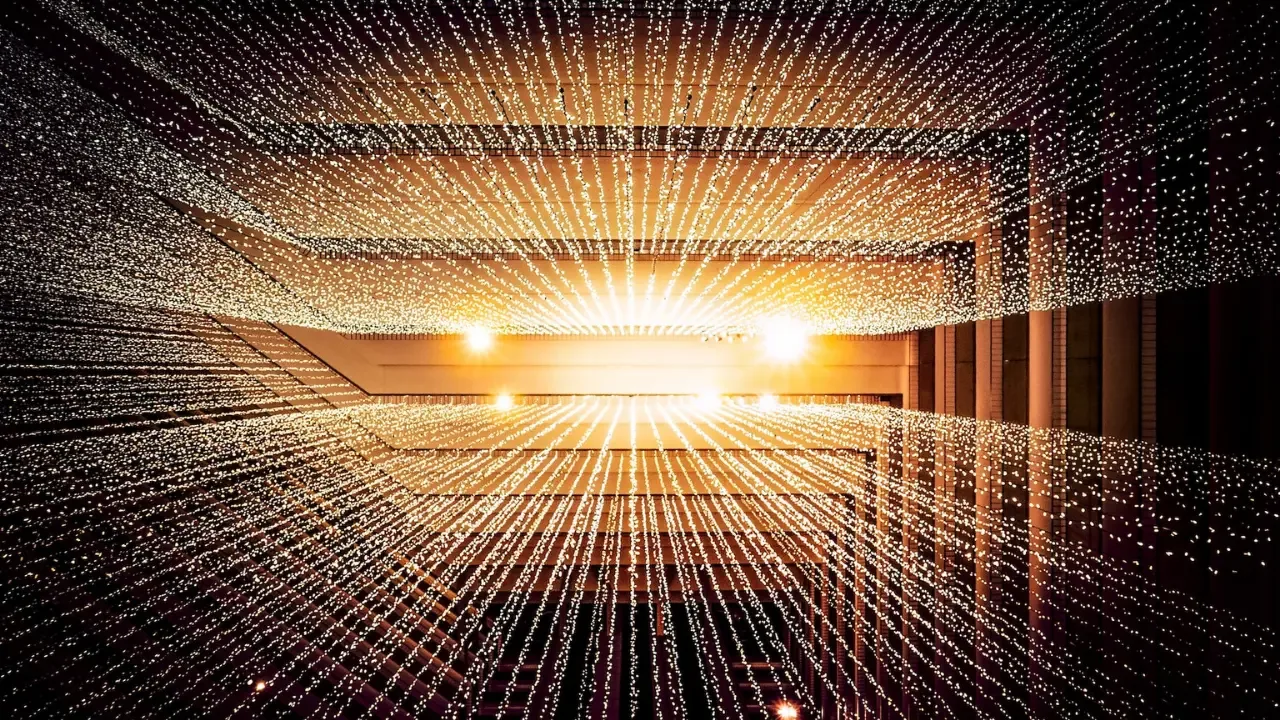
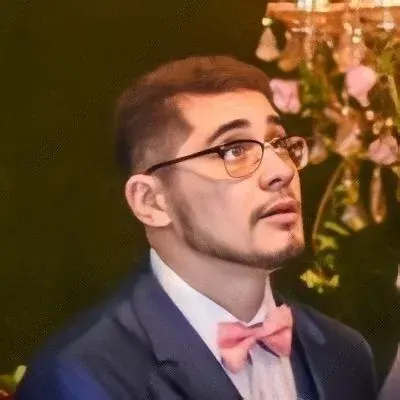
ππ₯πTech Blog: Creating an Empty Array and Appending to it in NumPy! π§
Are you stuck on how to create an empty array in NumPy and then append items to it? π€ Don't worry, we've got you covered! In this blog post, we'll address this common issue and provide easy solutions to save your coding day. Let's dive right in! πͺ
So, you want to create an empty array and add items to it one at a time? The initial approach might be to use a Python list and the append
method. Like this:
xs = []
for item in data:
xs.append(item)
But the question arises, can we use this list-style notation with NumPy arrays? π€·ββοΈ The answer is both yes and no. Let's explore the reasons behind this.
NumPy arrays have a fixed size, which makes appending items to them more complex than with regular Python lists. However, there are a couple of workarounds to achieve what you want. Let's explore them, shall we? π
1οΈβ£ Method 1: Using the np.append
function
The
np.append
function in NumPy allows you to add elements to an existing array. However, keep in mind that it creates a new array every time you call it, so it may not be the most efficient solution. Here's an example:
import numpy as np
xs = np.array([])
for item in data:
xs = np.append(xs, item)
2οΈβ£ Method 2: Creating an empty array with a specified size
Instead of dynamically appending, you can create an empty array with a predefined size using the
np.empty
function. Then, you can assign values to specific indices within the array. Here's an example:
import numpy as np
size = len(data) # Determine the size based on your data
xs = np.empty(size)
for i, item in enumerate(data):
xs[i] = item
3οΈβ£ Method 3: Preallocating an array with zeros and updating values
If you know the final size of your array in advance, you can use
np.zeros
to preallocate an array filled with zeros. Then, you can update the values as you iterate through your data. Here's an example:
import numpy as np
size = len(data) # Determine the size based on your data
xs = np.zeros(size)
for i, item in enumerate(data):
xs[i] = item
Now that you have three methods to create an empty array and append items to it using NumPy, try them out and see which one suits your needs best! π©βπ»π‘
But wait, there's more! If you want to learn even more about advanced array operations in NumPy and how it can level up your data manipulation game, check out our in-depth guide [link to your guide]. It's an incredible resource packed with tips, tricks, and examples to master NumPy like a pro! ππ₯
Happy coding! ππ¨βπ» And don't forget to share this post with your tech-savvy friends who might find it helpful. Keep exploring and never stop learning! πͺπ‘
Feel free to leave your thoughts and questions in the comments section below. We can't wait to hear from you! ππβ¨