How do I create a constant in Python?
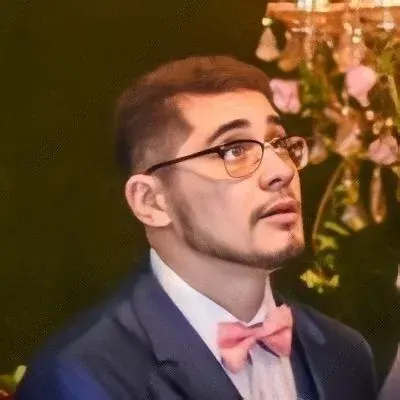
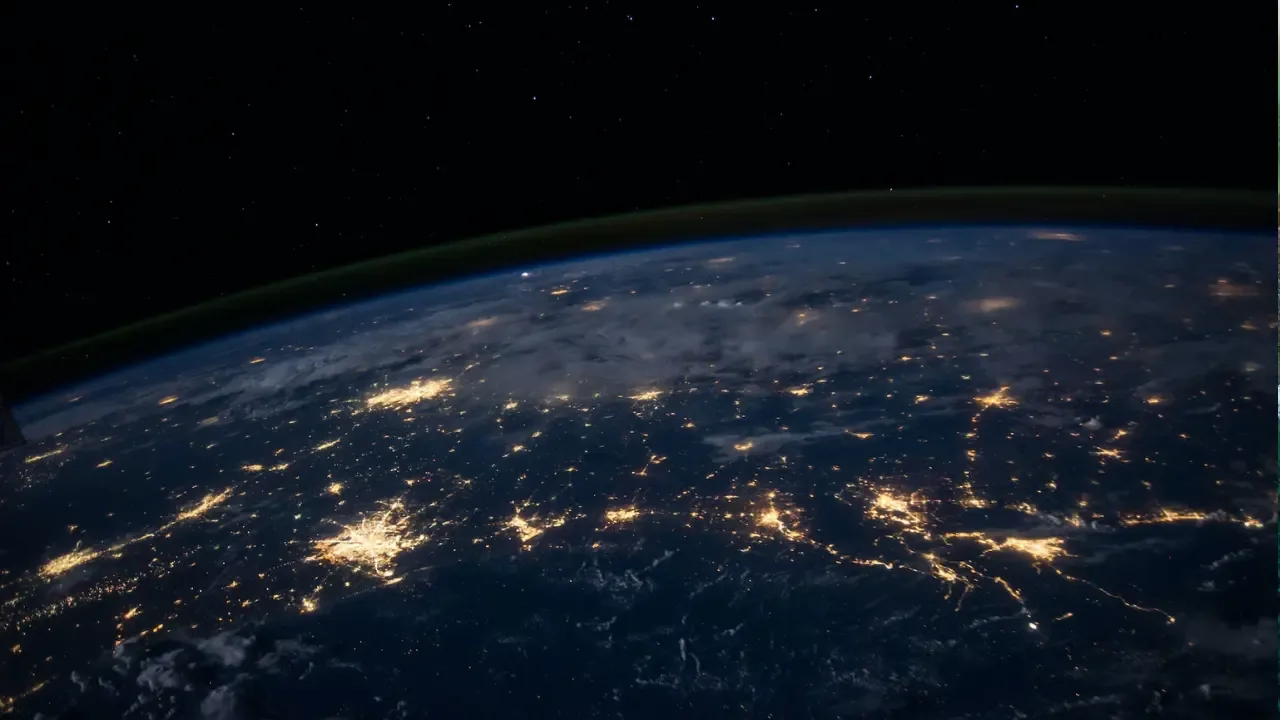
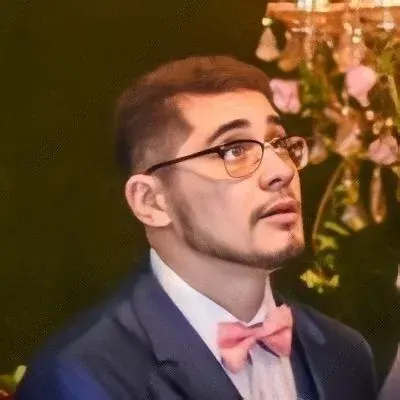
Creating Constants in Python: A Guide for Beginners 👨💻🔒
Creating constants in Python may not be as straightforward as it is in other programming languages like Java. However, fear not! In this guide, we will explore different approaches to declare a constant in Python and provide easy solutions to common issues you may encounter along the way. Let's dive in! 🏊♂️💨
The Challenge: Declaring Constants in Python
As mentioned, Python doesn't have built-in support for constants like Java does. In Python, variables are typically mutable, meaning their values can be changed. However, there are conventions and techniques you can follow to create constants that effectively achieve the same objective. 💡🐍
Approach 1: Using Uppercase Convention
One common convention in Python is to declare constants using uppercase letters. Although this doesn't technically prevent the value from being modified, it serves as a visual indicator to developers that the variable should not be changed. Let's see an example: 📝🆙
CONST_NAME = "Name"
By convention, using uppercase letters informs other developers that CONST_NAME
should be treated as a constant, and its value should remain constant throughout the code.
However, it's important to note that this approach does not enforce immutability. Developers can still modify the value of CONST_NAME
since Python doesn't provide a way to enforce constant values by default.
Approach 2: Using the constants
Module
If you want a more robust way to declare constants in Python, you can utilize the constants
module available from the enum
module. This approach offers more explicit and enforceable constant values. Let's see how it's done: 🔏🖇️
from enum import Enum
class Constants(Enum):
CONST_NAME = "Name"
In this approach, we define a new class using the Enum
base class from the enum
module. By creating a class that extends Enum
, we can add constant values as class attributes. The beauty of using this approach is that it prevents accidental modification of the constant values.
To access the constant value defined in the Constants
class, you would use:
name = Constants.CONST_NAME.value
Recap and Next Steps 📚🛠️
In this guide, we explored two different approaches to creating constants in Python. Although Python doesn't have built-in support for constants, we can use conventions like uppercase naming or the enum
module to achieve similar functionality.
Remember that the effectiveness of these approaches lies in following conventions and communicating the purpose of constants to other developers.
Now that you have a grasp of creating constants in Python, it's time to put your skills to the test! Try implementing constants in your next Python project and see how it improves code readability and maintainability.
If you found this guide helpful, share it with your developer friends and let them conquer the challenge of creating constants in Python too! 🚀💪
Got questions or alternative approaches? Leave a comment below and let's engage in a coding conversation! 😉💬