How do I convert a Pandas series or index to a NumPy array?
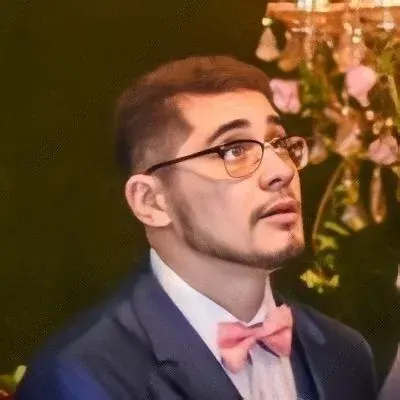
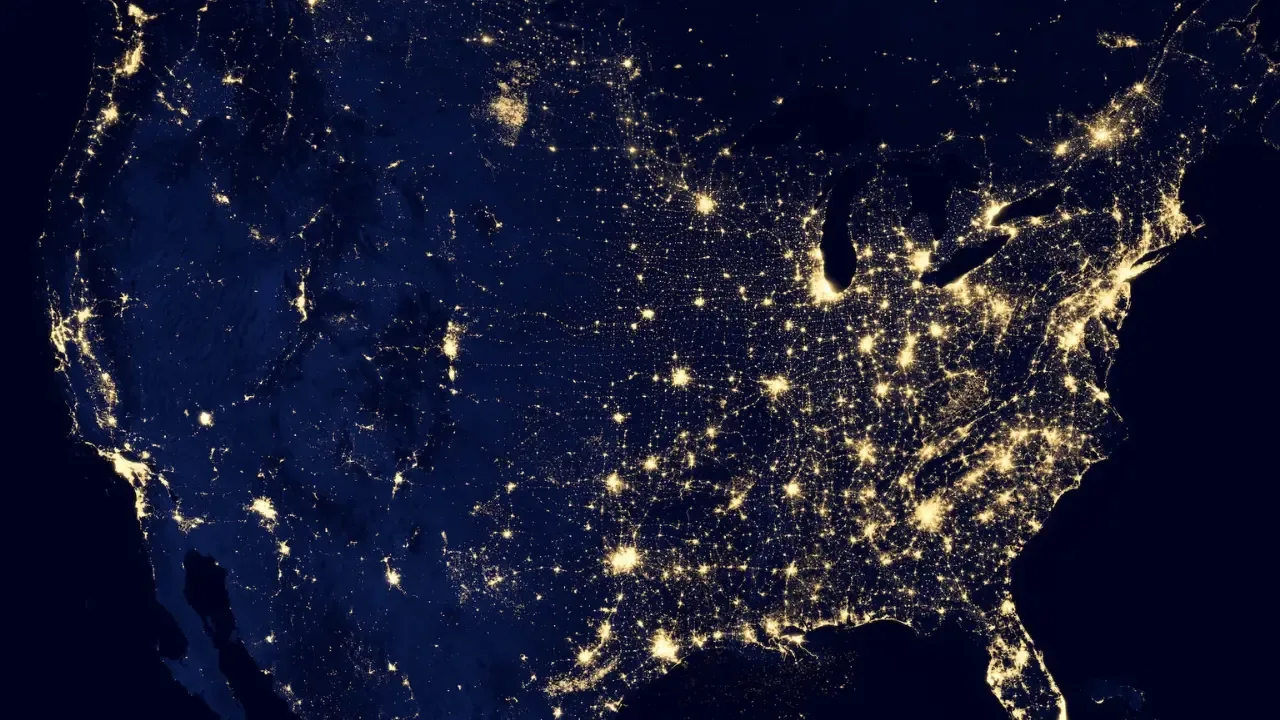
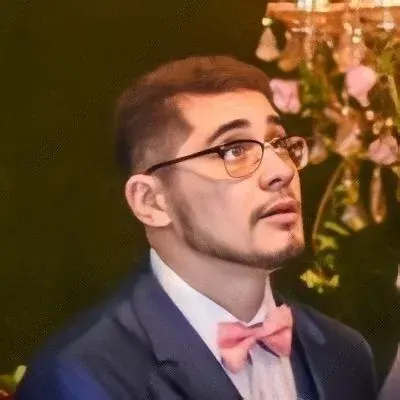
🎉 The Easy Guide to Convert Pandas Series or Index to NumPy Array! 🚀
Are you struggling to convert your Pandas series or index to a NumPy array? 🤔 Don't worry, we’ve got you covered! In this super helpful guide, we'll address common issues, provide easy solutions, and make you a pro at converting in no time! ✨
So, let's dive right in! 💪
The Problem: Getting the Index or Column of a DataFrame
The challenge is to convert the index or column of a DataFrame into a NumPy array or Python list. Sometimes, we need to perform mathematical operations or machine learning tasks that require NumPy arrays rather than Pandas objects.
The Solution: Converting Pandas Series to NumPy Array
To convert a Pandas series to a NumPy array, you can use the values
attribute of the series. It returns a NumPy array with the same data as the series. Let's see how it works with an example:
import pandas as pd
import numpy as np
# Create a sample series
series = pd.Series([1, 2, 3, 4, 5])
# Convert series to NumPy array
numpy_array = series.values
print(numpy_array)
Output:
array([1, 2, 3, 4, 5])
By accessing the values
attribute, you can effortlessly convert your Pandas series into a NumPy array! 🎉
The Solution: Converting Pandas Index to NumPy Array or Python List
Converting a Pandas index to a NumPy array or Python list is quite similar to converting a series. You can use the to_numpy()
method or the tolist()
method of the index object. Let's see both methods in action:
import pandas as pd
import numpy as np
# Create a sample index
index = pd.Index(['apple', 'banana', 'cherry', 'date', 'elderberry'])
# Convert index to NumPy array
numpy_array = index.to_numpy()
# Convert index to Python list
python_list = index.tolist()
print(numpy_array)
print(python_list)
Output:
array(['apple', 'banana', 'cherry', 'date', 'elderberry'])
['apple', 'banana', 'cherry', 'date', 'elderberry']
Voilà! With the to_numpy()
method and the tolist()
method, you can convert your Pandas index to either a NumPy array or a Python list with ease! 🙌
Your Turn to Convert with Confidence! 🎓
Now that you've learned how to convert a Pandas series or index to a NumPy array, you're ready to rock it on your own! 💥 Whether you're performing complex mathematical operations or feeding data to machine learning models, you can handle it all!
Go ahead and put your newfound knowledge into practice. Believe in yourself, and remember, you're just a few lines of code away from transforming your data like a pro! 🚀
If you have any questions or face any challenges during the conversion process, feel free to ask in the comments section below. Together, we'll conquer it! 👊
Happy converting, and may your data transformations be epic! ✨🔢
Stay curious and keep exploring! 🌐