How do I convert a Django QuerySet into list of dicts?
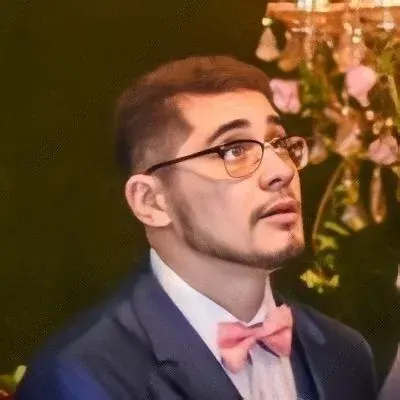
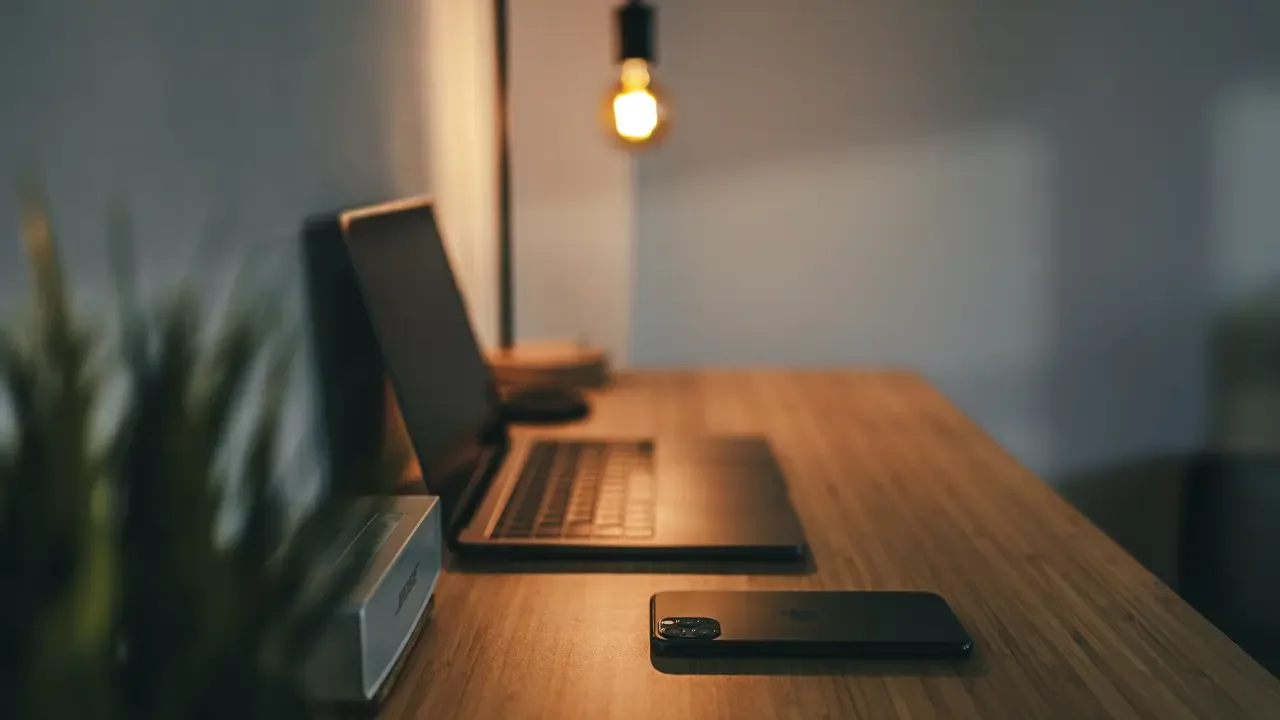
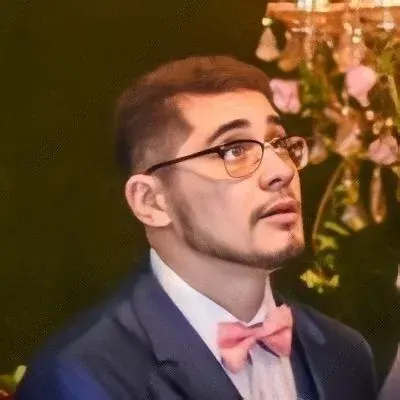
Converting a Django QuerySet into a List of Dicts: It's Easier Than You Think! 💪🔎
So, you want to convert a Django QuerySet
into a list
of dict
s? You're not alone! Many Django developers have encountered this challenge and wondered if there's an easy solution out there. Well, fear not! In this blog post, we're going to dive into this common issue and provide you with simple, yet powerful solutions. Let's get started! 😃
The Challenge: Converting a QuerySet into a List of Dicts 🤔
Let's first understand the problem at hand. By default, when you perform a Django query, you receive a QuerySet. This QuerySet is a powerful object that allows you to chain multiple filtering and sorting operations before executing the actual database query. However, sometimes you need to convert this QuerySet into a conventional Python data structure, like a list
of dict
s.
Solution 1: Using Python's values()
Method 🐍🔑
One of the simplest ways to achieve this conversion is by using the values()
method provided by Django QuerySets. This method returns a QuerySet that represents the data as a list of dictionaries, where each dictionary represents a single model instance. Here's an example:
queryset = YourModel.objects.all()
dict_list = list(queryset.values())
And voila! 🎉 By calling values()
on your QuerySet and then converting it into a list, you obtain a list of dictionaries that match your original QuerySet.
Solution 2: Utilizing Django's values()
Method with Specific Fields 🔍🔍
The previous solution might give you all the fields of your model, but what if you only need a specific subset of fields? Django's values()
method allows you to pass in the specific fields you want to retrieve. Here's an example:
queryset = YourModel.objects.all()
dict_list = list(queryset.values('field1', 'field2', 'field3'))
In this example, field1
, field2
, and field3
represent the fields you want to include in your resulting dictionary list. Feel free to customize it according to your needs! 🛠️
Solution 3: Leveraging Django's values_list()
Method 💡📋
Now, what if you don't need dictionaries but prefer a list of values for each instance of your QuerySet? Fear not! Django has got your back with the values_list()
method. This method returns a QuerySet that represents the data as a list of tuples, where each tuple contains the field values for a single model instance. Here's how you can use it:
queryset = YourModel.objects.all()
list_of_lists = list(queryset.values_list('field1', 'field2', 'field3'))
And just like that, you have your QuerySet converted into a list of tuples containing the desired fields. 🌟
Engage with Our Community! 🌐🙌
Did you find these solutions helpful? If you have any additional questions or other interesting approaches, we'd love to hear from you! Share your thoughts and engage with our tech-savvy community by commenting below.
Remember, sharing is caring! If you found this blog post useful, don't forget to share it with your fellow Django developers, so they can also benefit from these handy solutions! 🚀❤️
Happy coding! 👩💻👨💻