How do I connect to a MySQL Database in Python?
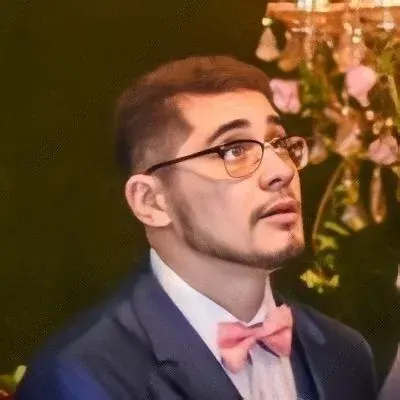
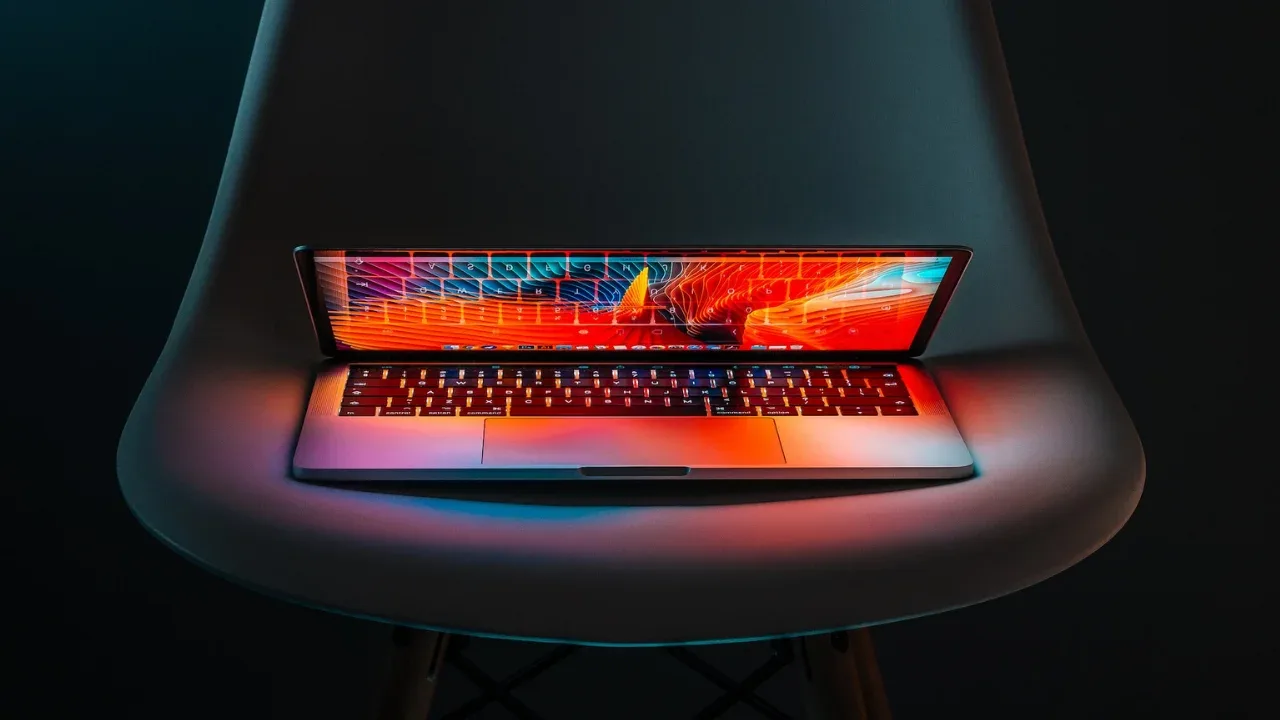
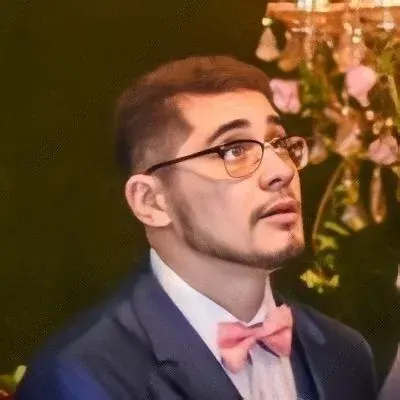
How to Connect to a MySQL Database in Python: A Complete Guide ππ§©πΎ
So you want to connect to a MySQL database using Python, huh? Well, you're in luck because I'm here to make it as easy as π°!
Why Connect to a MySQL Database in Python? π€
Python is a powerful and popular programming language for various tasks, including data manipulation and analysis. MySQL, on the other hand, is a widely used open-source relational database management system. Combining the two can unlock endless possibilities for data-driven applications.
Step 1: Install the Required Libraries ππ
Before we dive into the code, we first need to install the necessary libraries. The two key libraries you'll need for this task are:
mysql-connector-python: This library allows Python programs to connect to MySQL databases.
pandas (optional): If you want to work with data in a more convenient and efficient manner, pandas is a fantastic library to have.
You can install these libraries using pip, the Python package installer, by running the following commands in your terminal:
pip install mysql-connector-python
pip install pandas
Make sure you have an active internet connection for these installations to succeed.
Step 2: Import the Required Libraries π₯π
Now that we have the libraries installed, it's time to import them into our Python script. Open up your favorite code editor and create a new Python file (e.g., connect_to_mysql.py
).
Here's how you can import the necessary libraries:
import mysql.connector
import pandas as pd # (optional)
Step 3: Establish a Connection π€π
To connect to a MySQL database, you'll need a few key pieces of information:
host: The hostname or IP address where your MySQL server is located.
user: The username to authenticate your connection.
password: The password corresponding to the provided username.
database: The specific database within the MySQL server to which you want to connect.
Let's assume your MySQL server is running locally on your machine, and you want to connect to the "mydatabase" database with the username "myuser" and password "mypassword". Here's how you can establish a connection:
host = "localhost"
user = "myuser"
password = "mypassword"
database = "mydatabase"
connection = mysql.connector.connect(
host=host,
user=user,
password=password,
database=database
)
If the connection is successful, you're now ready to interact with your MySQL database using Python!
Step 4: Perform Database Operations πͺπΌπ
With a successful connection established, you can now perform various database operations like querying data, inserting records, or updating existing entries. Here's an example of retrieving data from a table:
# Assuming you have a table called "users"
query = "SELECT * FROM users"
# Execute the query
cursor = connection.cursor()
cursor.execute(query)
# Fetch all rows from the result
result = cursor.fetchall()
# (optional) Use pandas to work with the data more easily
df = pd.DataFrame(result, columns=cursor.column_names)
# Print the result
print(df)
Feel free to modify the query or perform other operations based on your specific needs.
Step 5: Close the Connection πͺβ
Once you're done with your database operations, it's good practice to close the connection to free up resources. You can simply add the following line of code at the end of your script:
connection.close()
Wrapping Up ππ
Congratulations! You now know how to connect to a MySQL database in Python like a pro! π
Remember, connecting to a database is just the beginning. With your newfound knowledge, you can explore more complex operations, build robust applications, and unlock the true potential of data-driven Python programming.
So go ahead and start connecting, querying, and building remarkable things with Python and MySQL! And don't forget to share your experiences and any questions you have in the comments section below. Let's keep the conversation going! ππ
Happy coding! ππ»