How do I concatenate two lists in Python?
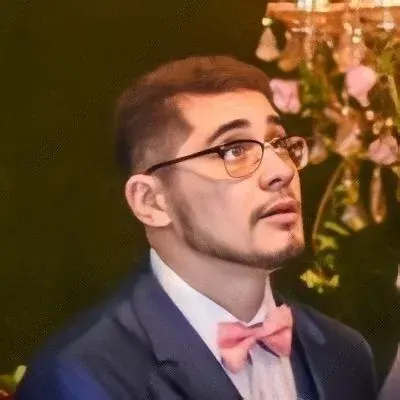
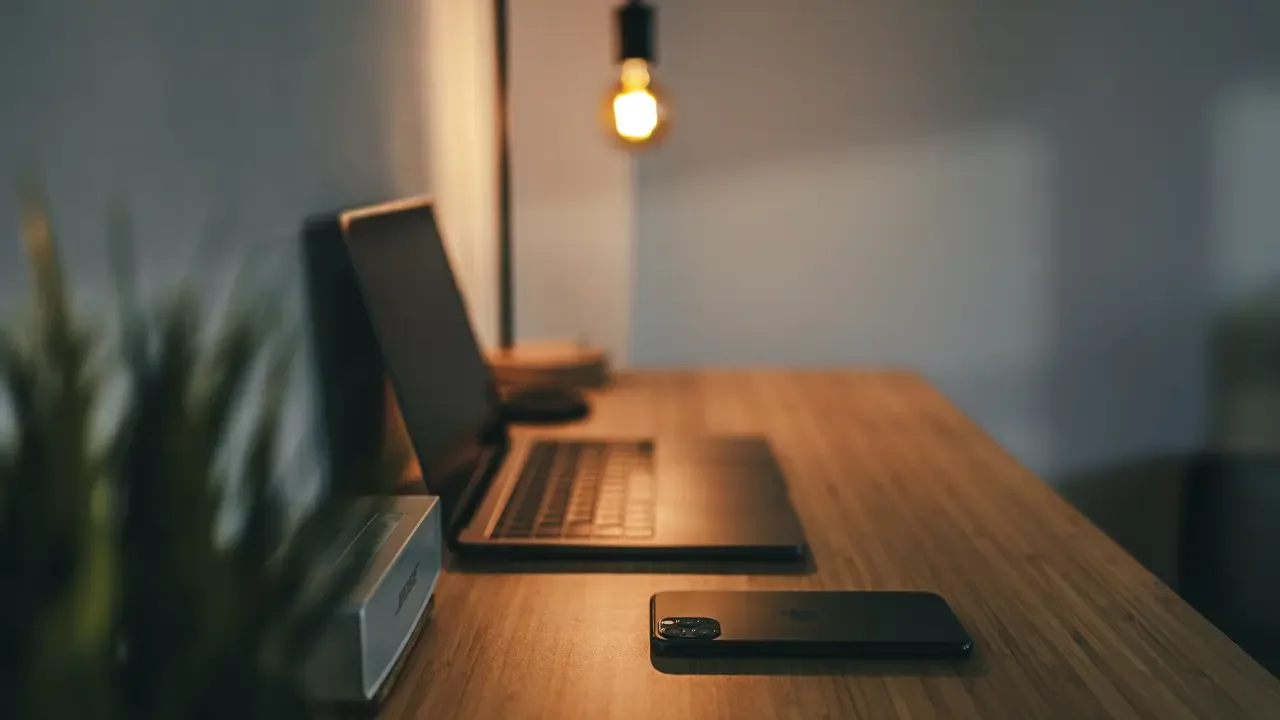
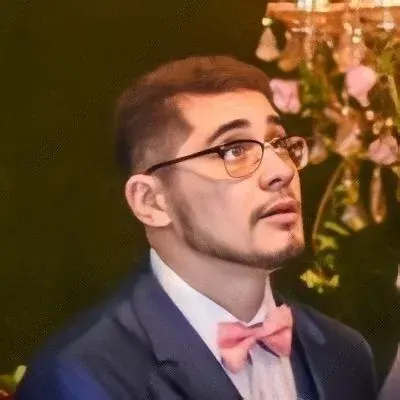
How to Concatenate Two Lists in Python: A Quick and Easy Guide! 🐍😎
So you want to concatenate two lists in Python? No worries, it's simpler than you think! In this guide, we'll cover everything you need to know about combining lists effortlessly. Whether you're a beginner or an experienced Pythonista, this post has got you covered. Let's dive right in! 💪🚀
Understanding the Problem ✨
To concatenate two lists means to join them together and create a single list containing all the elements from both lists. In our example, we have listone
and listtwo
:
listone = [1, 2, 3]
listtwo = [4, 5, 6]
And our expected outcome is to have a new list called joinedlist
that contains all the elements from both listone
and listtwo
, like this:
joinedlist = [1, 2, 3, 4, 5, 6]
The Solution: Using the +
Operator 🔗
Python provides a simple and elegant way to concatenate lists using the +
operator. Here's how you can do it:
joinedlist = listone + listtwo
That's it! 🎉 By using the +
operator, you can easily concatenate two lists and store the result in a new variable (joinedlist
in our case).
Let's See It in Action! 💡
Take a look at the following Python code that demonstrates the concatenation process:
listone = [1, 2, 3]
listtwo = [4, 5, 6]
joinedlist = listone + listtwo
print(joinedlist)
When you run this code, you'll see the following output:
[1, 2, 3, 4, 5, 6]
Isn't that awesome? You've successfully concatenated two lists using Python! 🎊
Common Pitfalls and Tips ⚠️💡
Pitfall: Mutability of Lists
It's important to note that when you concatenate two lists using the +
operator, a brand new list is created. This means that the original lists, listone
and listtwo
, remain unchanged. If you modify the concatenated list, it won't affect the original lists.
Tip: Concatenate Multiple Lists
You can concatenate more than two lists by extending the same technique. Simply use the +
operator to join as many lists as you need!
joinedlist = listone + listtwo + listthree + listfour
Tip: Preserve the Original Lists
If you want to keep the original lists intact while creating the concatenated list, make sure to create a copy of the lists and concatenate them separately:
listone_copy = list(listone)
listtwo_copy = list(listtwo)
joinedlist = listone_copy + listtwo_copy
Time to Get Concatenating! ⚙️
Now that you know how to concatenate lists in Python, it's time to put your knowledge into action! Feel free to experiment with different lists and explore the endless possibilities of manipulating data using Python's versatile list operations.
If you found this guide helpful, don't forget to share it with your fellow Python enthusiasts! 👍✨
Got any questions or suggestions? Let me know in the comments below. Happy coding! 🖥️💻
#Python #Lists #Concatenation #CodingTips