How do I clone a Django model instance object and save it to the database?
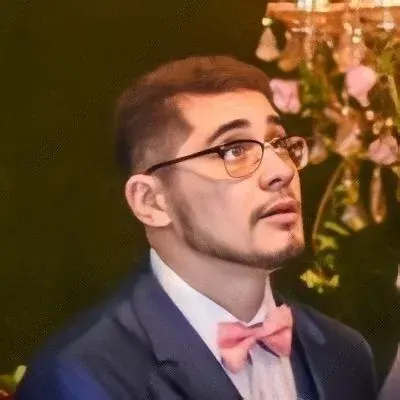
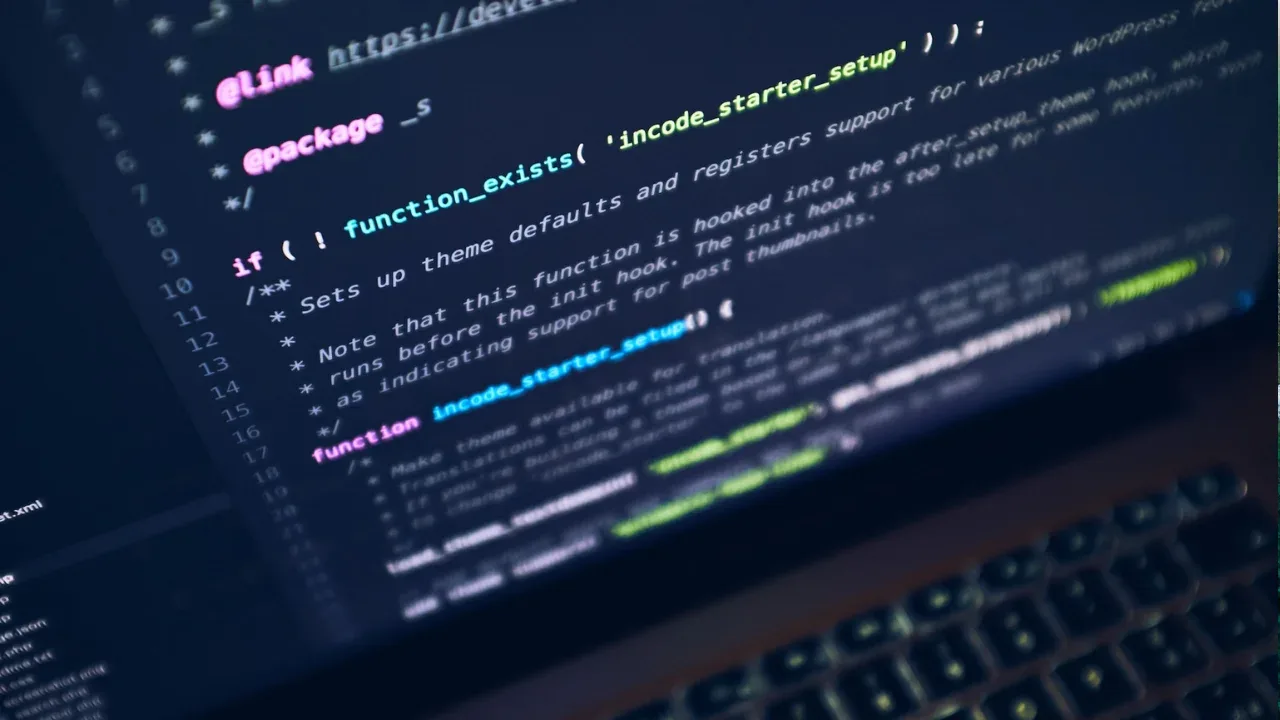
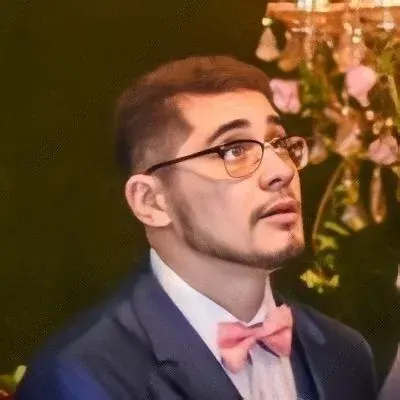
🧬 Cloning a Django Model Instance: A Simple Guide
So, you want to clone a Django model instance object and save it to the database? You've come to the right place! Cloning a model instance can be quite handy when you want to duplicate an existing object, but with some slight modifications. Let's dive in and unravel the steps involved in this process.
🎯 The Problem
Let's start by understanding the specific problem at hand. Imagine you have a Django model called Foo
, and you already have an existing object with the following details:
Foo.objects.get(pk="foo")
# Output: <Foo: test>
Now, the challenge is to add another object to the database that is an exact copy of the above object, but with a different primary key.
🔍 Common Issues
Before we explore the solution, it's essential to address some common hurdles people might face when attempting to clone a model instance:
Data Integrity: Cloning an object may have implications for data integrity, especially if relationships or dependencies exist between various model instances. It's crucial to consider and appropriately handle such relationships during the cloning process.
Unique Constraints: If your model has unique constraints on any fields, duplicating an object using the same field values might result in conflicts. We'll explore how to handle this scenario as part of the solution.
🛠️ The Solution
To clone a Django model instance, follow these straightforward steps:
Retrieve the object you want to clone from the database using appropriate query methods like
get()
orfilter()
. In our case, we already have the object with a primary key (pk
) of "foo".Create a new instance of the model, but do not save it to the database yet. You can use the
copy()
method provided by Django'sModel
class to achieve this:original_object = Foo.objects.get(pk="foo") cloned_object = original_object.copy()
If your model has any fields other than the primary key that need to be modified or set differently, you can do so on the cloned object:
cloned_object.pk = "new_key" cloned_object.title = "New Title"
Handle any unique constraints on your model's fields. If you encounter a conflict with unique fields while saving, catch the
IntegrityError
exception and modify the field values uniquely:from django.db import IntegrityError try: cloned_object.save() except IntegrityError: # Handle unique constraint conflicts here cloned_object.pk = "another_new_key" cloned_object.save()
Voilà! Your cloned object is now saved to the database with the desired changes.
🚀 The Call-to-Action
Cloning a Django model instance and saving it to the database can open up a world of possibilities for your application. It enables you to efficiently create duplicates with ease. So why not give it a try and see how it simplifies your development process?
If you found this guide helpful, make sure to share it with your fellow Django developers. And if you have any more questions or suggestions, feel free to leave a comment below. Happy cloning! 👯♂️