How do I check whether a file exists without exceptions?
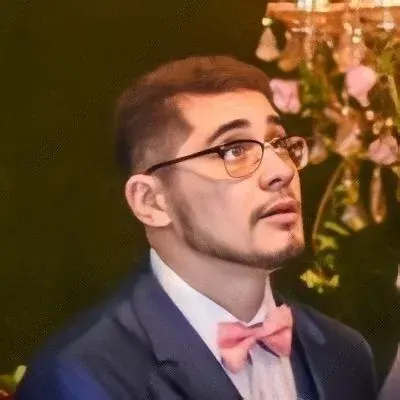
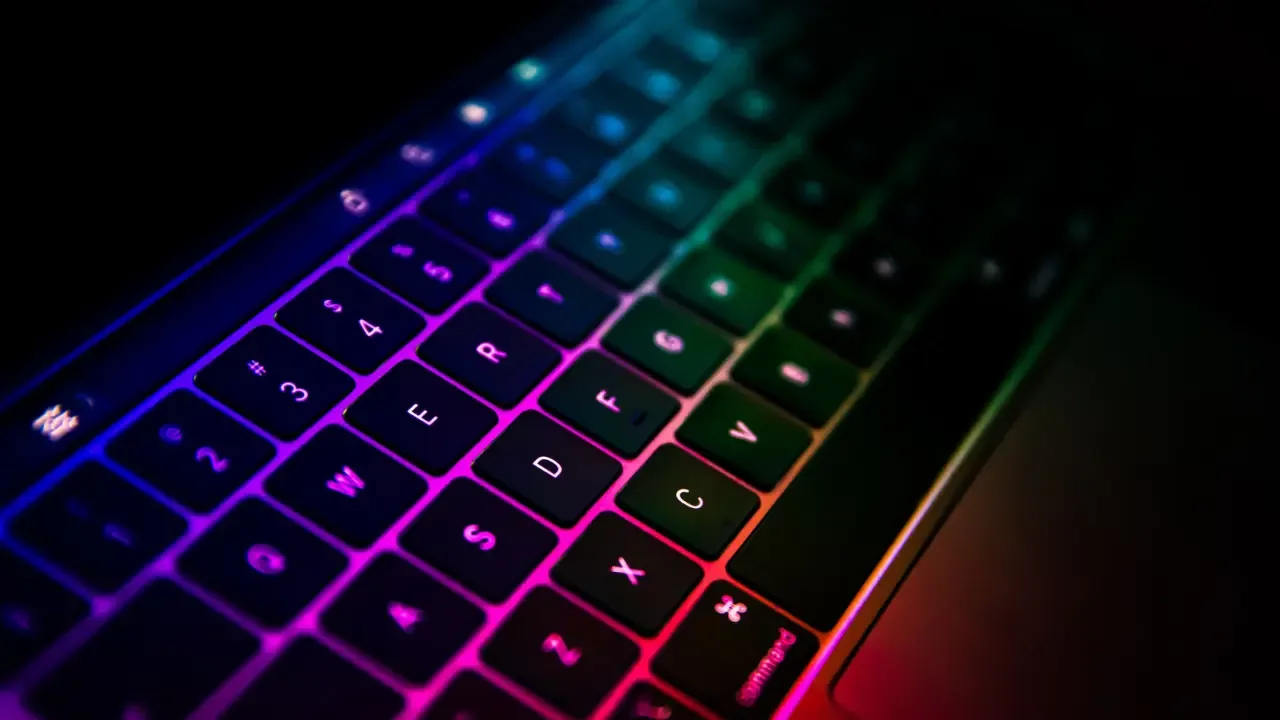
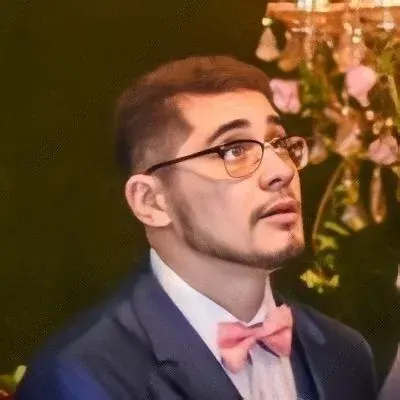
📂 How to Check Whether a File Exists Without Exceptions
Have you ever wanted to check if a file exists in your Python program, without having to deal with those pesky exceptions? Well, you're in luck! In this blog post, we'll explore different ways to determine if a file exists and avoid exceptions.
📝 The Common Issue
Most developers would agree that using exceptions for flow control is not the best practice. When it comes to checking file existence, the most common solution is to use the try-except
statement. However, this can lead to code that is harder to read and maintain.
💡 The Easy Solutions
Fortunately, Python provides alternative methods that allow us to check for file existence without relying on exceptions. Let's explore two commonly used approaches:
1. Using the os.path
module
The os.path
module in Python provides several functions that can be used to manipulate file and directory paths. One of these functions is os.path.exists()
, which returns True
if a file or directory exists, and False
otherwise.
Here's an example:
import os
file_path = '/path/to/file.txt'
if os.path.exists(file_path):
print("File exists!")
else:
print("File does not exist.")
By using os.path.exists()
, we can easily check if a file exists without having to catch any exceptions.
2. Using the pathlib
module
The pathlib
module, introduced in Python 3.4, provides an object-oriented approach to handle file paths. This module offers the Path.exists()
method, which works similarly to os.path.exists()
.
Take a look at this example:
from pathlib import Path
file_path = Path('/path/to/file.txt')
if file_path.exists():
print("File exists!")
else:
print("File does not exist.")
Using pathlib.Path.exists()
, we can achieve the same result as before, but in a more object-oriented manner.
📣 The Compelling Call-to-Action
Now that you know two convenient ways to check if a file exists without exceptions, give them a try in your next Python project! Don't let exceptions hinder your code's readability and maintainability.
Share this blog post with your fellow Python developers, and let's build better, cleaner code together! 🚀
👉 What are your favorite techniques for handling file existence? Let us know in the comments below!