How do I check if a variable exists?
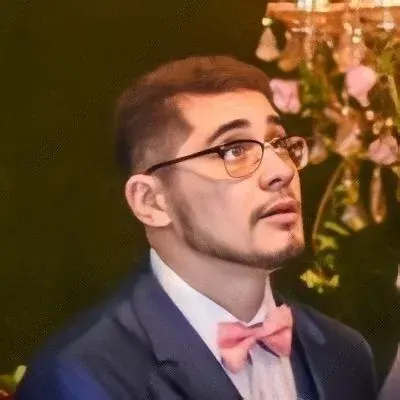
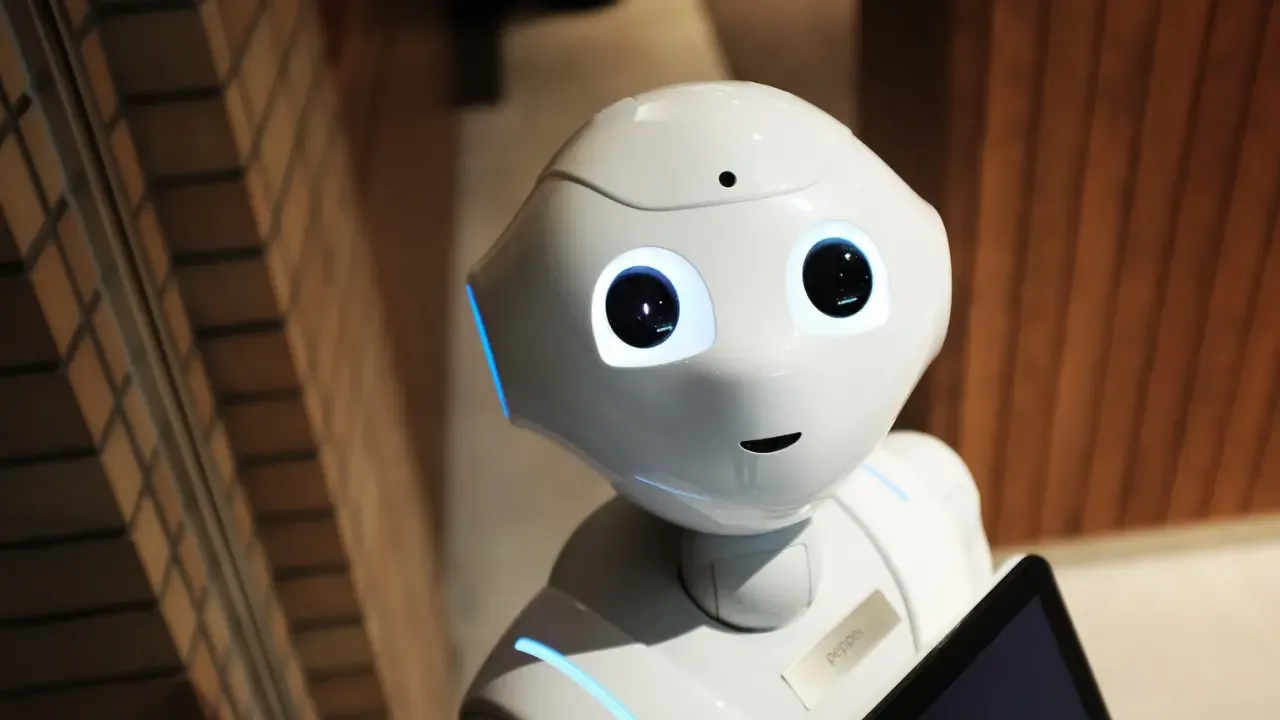
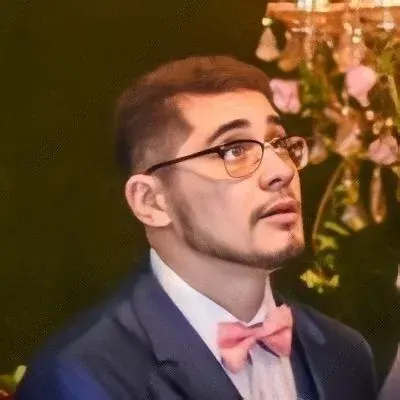
How to Check if a Variable Exists in Python 🐍
If you've ever wondered how to check if a variable exists in Python, you're not alone. This is a common issue that many developers encounter, especially when dealing with larger codebases or complex algorithms. In this article, we'll explore different techniques to tackle this problem and discuss how to do it effectively. Let's dive in! 💻
The Traditional Approach
The traditional way of checking if a variable exists in Python involves using a try-except
block, just like the code snippet you provided. This approach works fine, but let's explore other alternatives to handle this situation without relying on exceptions. 😉
Method 1: Using the globals()
Function
One simple approach is to use the globals()
function, which returns a dictionary of all global variables in the current scope. You can then check if a specific variable is present in this dictionary. Here's an example:
if 'myVar' in globals():
# Do something.
By using the in
keyword, this code will check if the variable 'myVar'
exists in the global scope. If it does, the code inside the if
statement will be executed. Otherwise, it will be skipped.
Method 2: Using the locals()
Function
Similar to globals()
, you can also use the locals()
function to check for the existence of a variable within the local scope. Here's how you can do it:
if 'myVar' in locals():
# Do something.
In this case, Python will search for the variable 'myVar'
specifically within the local scope, which is the current function or method you're in. If the variable is found, the code will be executed.
Method 3: Using the dir()
Function
The dir()
function returns a list of all defined symbols (variables, functions, classes, etc.) in the current module. You can check if a variable exists using this approach:
if 'myVar' in dir():
# Do something.
By calling dir()
without any arguments, you get a list of all defined symbols in your current scope. By checking if 'myVar'
is present in this list, you can determine if the variable exists.
🚀 It's Time to Take Action!
Now that you've learned different methods to check if a variable exists in Python, it's your turn to put this knowledge into practice. Experiment with these approaches, and choose the one that best fits your needs and coding style. Remember, there's often more than one way to solve a problem! 💪
If you found this article helpful, don't forget to share it with fellow developers who might encounter the same issue. Let's spread the knowledge!
Leave a comment below and let me know which method you prefer or if you have any additional tips to share. Happy coding! 😄👨💻