How do I check if a string represents a number (float or int)?
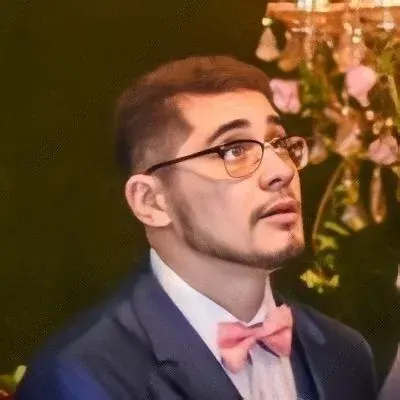
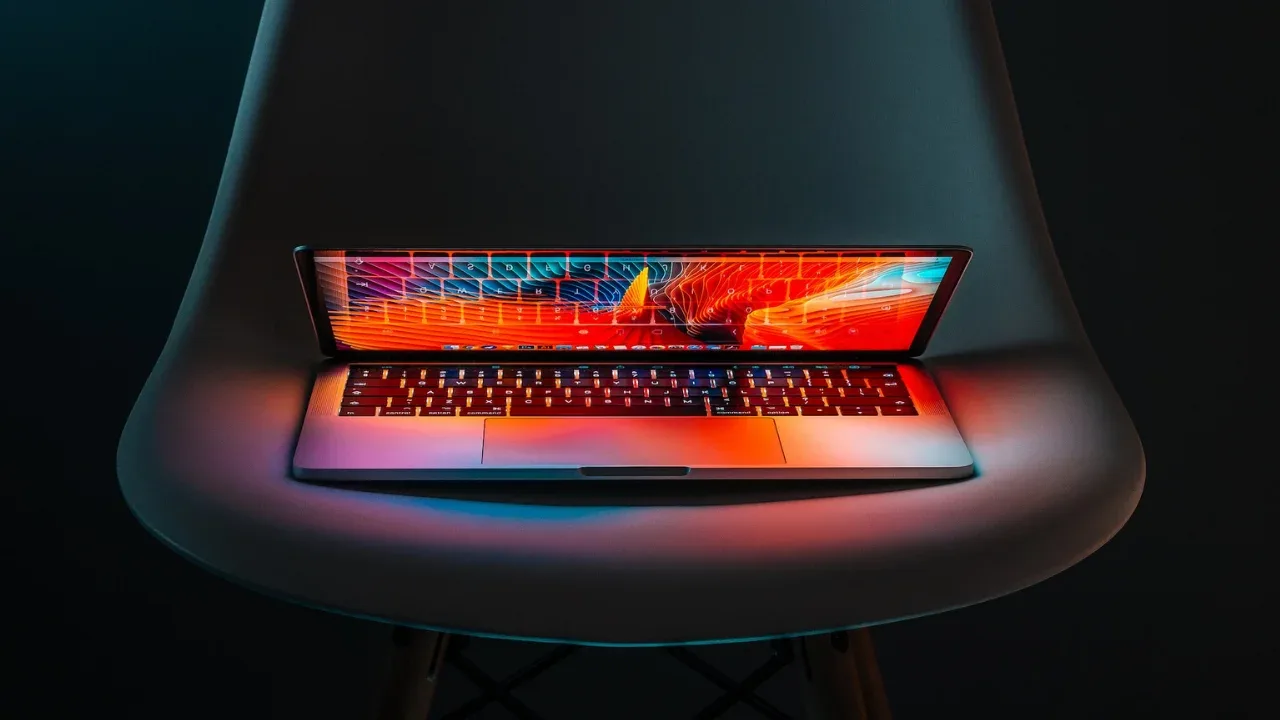
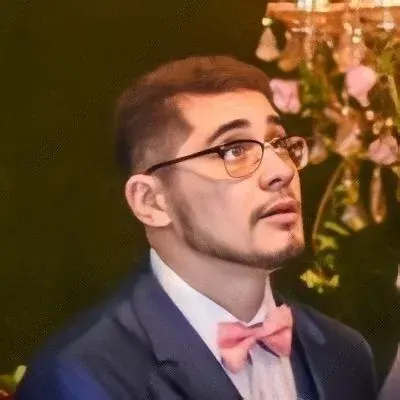
๐ How to Check if a String Represents a Number (Float or Int) in Python
Have you ever come across a situation where you need to check if a string in your Python program represents a valid number, either a float or an integer? You're not alone! This is a common problem that many developers face. In this blog post, we'll discuss different ways to tackle this issue and provide you with easy solutions.
The Clunky Approach ๐ค
Before we dive into the easy solutions, let's take a look at the "clunky" approach mentioned in the context you provided. Here's the code snippet:
def is_number(s):
try:
float(s)
return True
except ValueError:
return False
This approach works, but it might not be the most elegant solution. However, don't worry, there are better ways to achieve the same result!
Solution 1: Regular Expression ๐งต
One way to check if a string represents a number is by using regular expressions. Python provides the re
module for regular expression operations. Let's see how we can use it:
import re
def is_number(s):
return bool(re.match(r'^[-+]?[0-9]*\.?[0-9]+$', s))
In this solution, we use the re.match()
function to match the string against a regular expression pattern. The pattern ^[-+]?[0-9]*\.?[0-9]+$
ensures that the string matches the criteria of a valid number, allowing an optional sign (-
or +
), any number of digits before and after the decimal point, and at least one digit.
Solution 2: Built-in Functions ๐งฐ
Python also provides a couple of built-in functions to check if a string represents a number. Let's explore these options:
Solution 2.1: isdigit()
and isdecimal()
Functions
def is_number(s):
return s.isdigit() or s.isdecimal()
The isdigit()
function checks if the string contains only digits (0-9), while the isdecimal()
function checks if the string contains only decimal characters (0-9), such as Unicode numeric characters.
Solution 2.2: isnumeric()
Function
def is_number(s):
return s.isnumeric()
The isnumeric()
function checks if the string contains only numeric characters, including digits from various scripts, such as ASCII digits, Arabic-Indic digits, or full-width digits.
Conclusion and Call-to-Action โ
Checking if a string represents a number can be tricky, but with these easy solutions, you can handle it like a pro! Whether you choose the regular expression approach or the built-in functions approach, you have the tools to solve this problem efficiently.
Now, it's your turn to give it a try! Implement these solutions in your code and see the magic happen. If you have any questions or other tips to share, feel free to leave a comment below. Happy coding! ๐ป๐ก