How do I check if a list is empty?
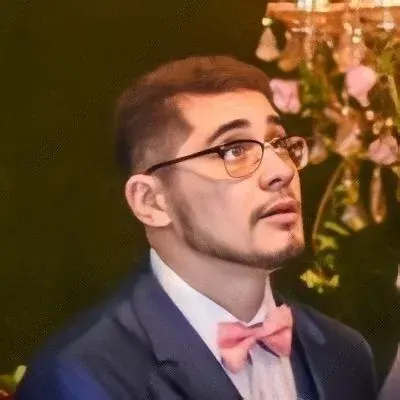
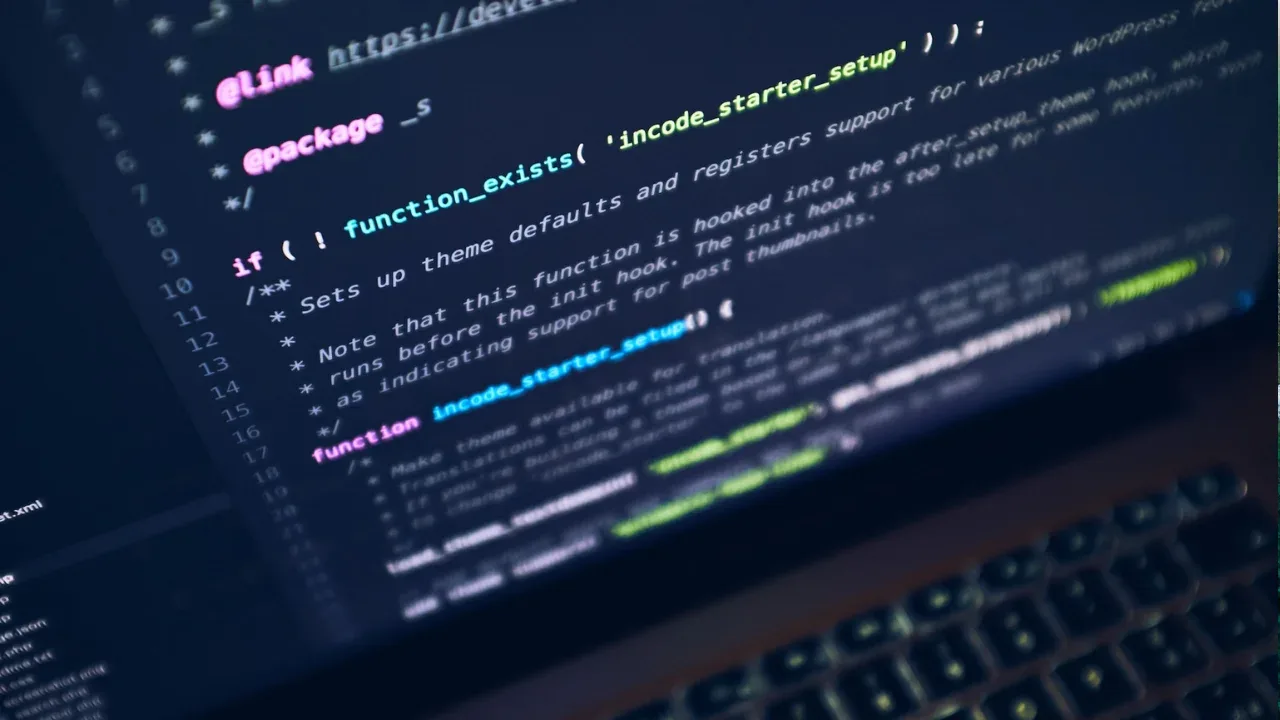
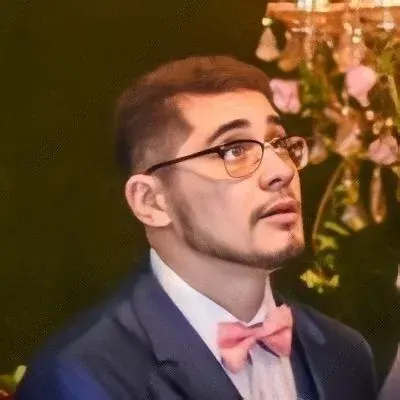
<h2>🔍 How to Check if a List is Empty? 🔍</h2>
<p>Do you often find yourself struggling to determine whether a list in your code is empty or not? Don't worry, you're not alone! In this blog post, we'll explore the common issues surrounding this question and provide you with easy solutions to check if a list is empty. Let's dive in! 🚀</p>
<h3>⚠️ The Common Misconception ⚠️</h3>
<p>Before we discuss the solutions, let's address a common misconception. Many beginners tend to assume that an empty list has a value of <code>None</code> or <code>0</code>. However, that's not the case! An empty list is simply a list with no elements. 🙅♂️</p>
<h3>🔑 Solution #1: Using the <code>len()</code> Function 🔑</h3>
<p>One easy way to check if a list is empty is by using the <code>len()</code> function. This function returns the number of elements in a list. So, if the length of the list is <code>0</code>, that means the list is empty. Let's take a look at an example:</p>
a = []
if len(a) == 0:
print("The list is empty!")
else:
print("The list is not empty.")
<p>The output of this code will be:</p>
The list is empty!
<h3>🔑 Solution #2: Using the Truthiness of Lists 🔑</h3>
<p>In Python, empty lists have a "truthiness" value of <code>False</code>. So, you can directly use an empty list in an <code>if</code> statement to check if it's empty or not. Let's see it in action:</p>
a = []
if not a:
print("The list is empty!")
else:
print("The list is not empty.")
<p>Again, the output of this code will be:</p>
The list is empty!
<h3>🔑 Solution #3: Checking for Emptiness in an Index-Based Approach 🔑</h3>
<p>Another approach is to check if the list is empty by accessing its first element. If the list is empty, an attempt to access the first element will result in an <code>IndexError</code>. Let's take a look at the code:</p>
a = []
try:
first_element = a[0]
print("The list is not empty.")
except IndexError:
print("The list is empty!")
<p>Yet again, the output of this code will be:</p>
The list is empty!
<h3>👉 Wrap Up and Take Action! 👈</h3>
<p>Now you're equipped with three easy solutions to check if a list is empty. Remember, you can use the <code>len()</code> function, the truthiness of lists, or an index-based approach.</p>
<p>So, next time you encounter an empty list, don't panic! Try out one of the solutions mentioned above and maintain your code's elegance and efficiency. 🧐</p>
<p>If you found this blog post helpful, feel free to <strong>share it with your fellow coders</strong> who might also struggle with this question. And don't forget to leave a comment below to share your thoughts or ask any related questions. Let's continue to learn and grow together! 🌱</p>
<p>Happy coding! 😄👩💻👨💻</p>