How do I check if a directory exists in Python?
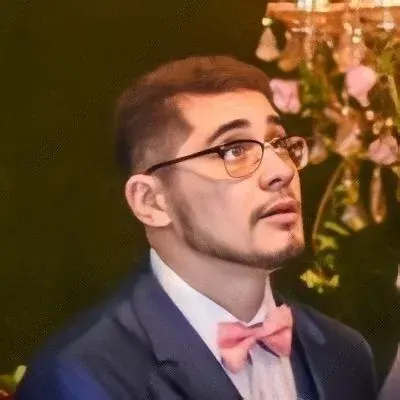
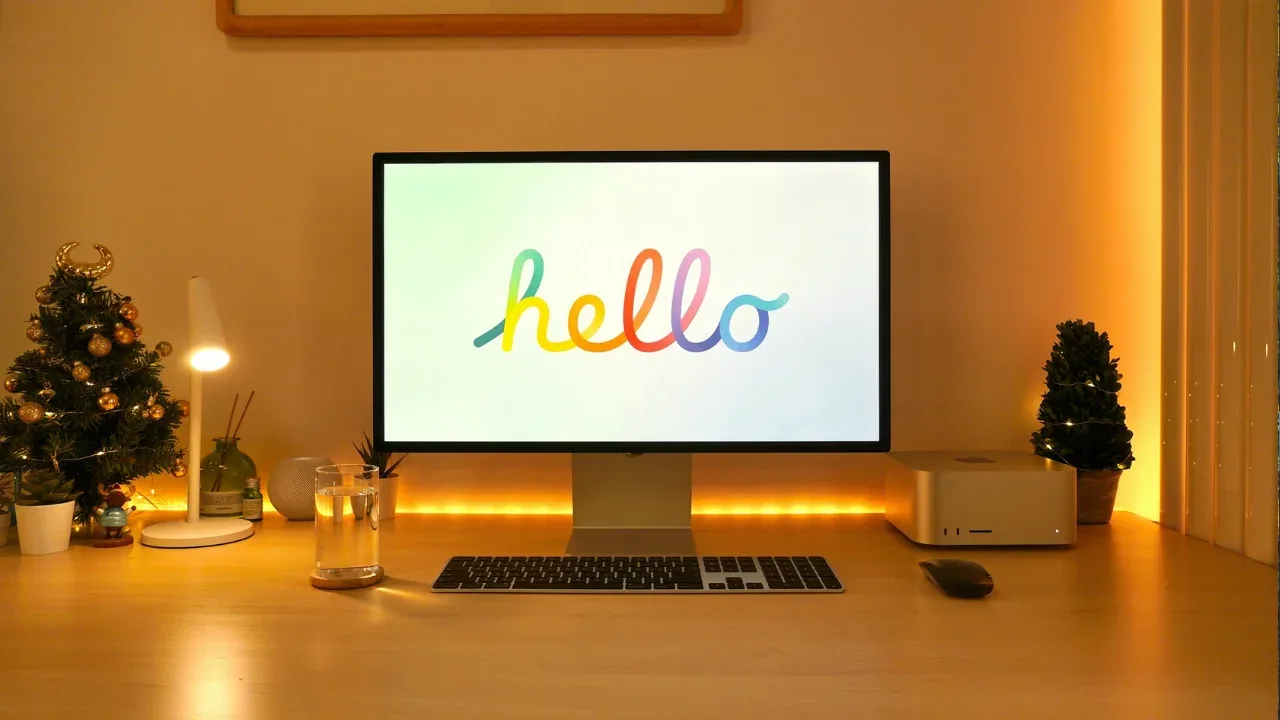
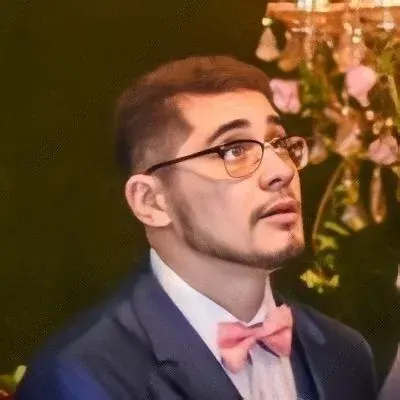
📝🤔 How do I check if a directory exists in Python?
Have you ever found yourself in the depths of Python programming and needed a way to check if a directory exists? It's a common problem that can be quite frustrating. But fear not! In this post, I'll show you some easy ways to tackle this issue and ensure your code runs smoothly. 🐍💻
The Problem: Checking if a directory exists in Python can be a challenge, especially if you're new to the language. But don't worry, I've got you covered! 🛡️
The Solution: There are multiple ways to check for the existence of a directory in Python, and I'll walk you through some of the most popular methods. Let's dive in! 🏊♂️
Using the
os.path
module 📂:
import os.path
path = '/path/to/directory'
if os.path.isdir(path):
print("Directory exists!")
else:
print("Directory doesn't exist.")
In this method, we use the os.path.isdir()
function to check if the given path represents a directory. If it does exist, we'll see the friendly message "Directory exists!".
Using the
pathlib
module 💡:
from pathlib import Path
path = Path('/path/to/directory')
if path.is_dir():
print("Directory exists!")
else:
print("Directory doesn't exist.")
With the pathlib
module, we can use the .is_dir()
method on a Path
object to check for directory existence. It's as simple as that!
Pro Tips:
Instead of hard-coding the path, use variables to make your code more flexible and reusable.
Combine these methods with exception handling to gracefully handle errors, such as permission issues or filesystem problems.
➡️ Takeaways: Checking if a directory exists in Python doesn't have to be a daunting task. By using either the os.path
module or the pathlib
module, you can easily determine the existence of a directory and proceed with your code accordingly.
So, next time you find yourself in need of checking a directory's existence, remember these straightforward methods! Happy coding! 🚀💻
Have you encountered any other challenges in Python development? Let me know in the comments below! 👇😊