How do I change the string representation of a Python class?
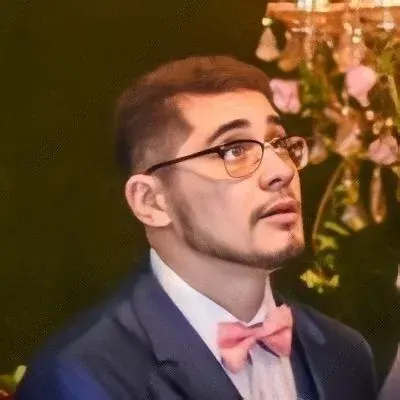
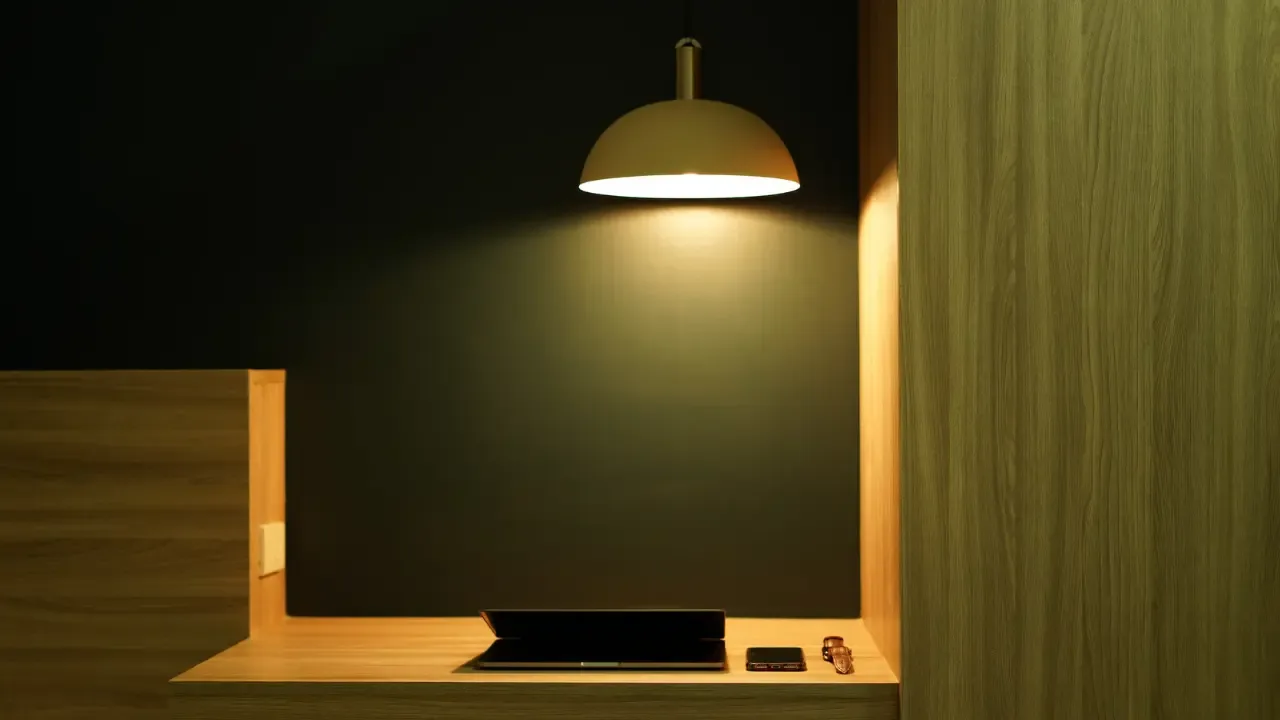
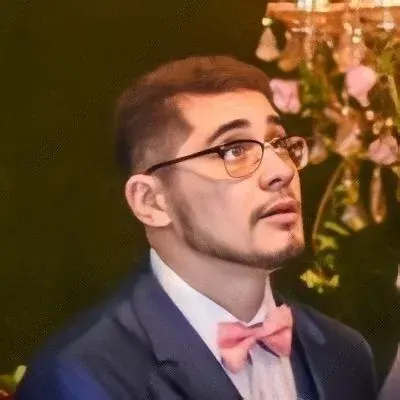
How to Customize the String Representation of a Python Class ππ
So you want to change the way your Python class is displayed as a string? Just like in Java where you can override the toString()
method, Python also provides a way to customize the string representation of your class instances. Let's see how you can do that! π
The Problem π
By default, when you try to print an instance of a Python class, like our example PlayCard
class, you get a string containing the class name and a memory address, which is not very helpful:
>>> print(c)
<__main__.Card object at 0x01FD5D30>
But what we really want is something more meaningful, like the actual value of the card, such as "Aβ£". So how can we achieve that?
The Solution π‘
To customize the string representation of a class in Python, you need to define a special method called __str__()
in your class. This method should return the desired string representation of the instance. Let's update our PlayCard
class to include the __str__()
method:
class PlayCard:
def __init__(self, value, suit):
self.value = value
self.suit = suit
def __str__(self):
return f"{self.value}{self.suit}"
Now, when you print an instance of PlayCard
, it will use the string returned by the __str__()
method:
>>> c = PlayCard("A", "β£")
>>> print(c)
Aβ£
Much better! We've customized the string representation of our class instance. You can now use any logic you want within the __str__()
method to format the string representation according to your needs.
Conclusion π
Changing the string representation of a Python class is as easy as defining the __str__()
method within your class. This allows you to display a more meaningful representation of your objects when using the print()
function or any other string-related operations.
So go ahead and start customizing the string representation of your classes. Impress your friends and colleagues with your Python skills! π
Have you ever customized the string representation of a Python class? Share your experiences and any cool tips you have in the comments below! Let's make our Python code look even cooler! π€π₯