How do I add a placeholder on a CharField in Django?
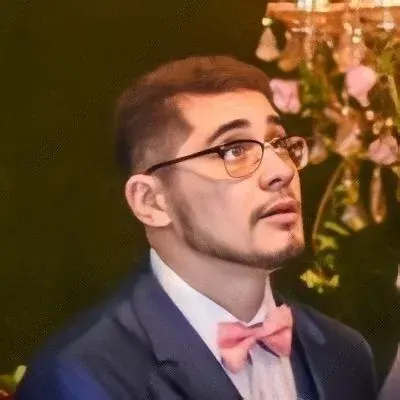
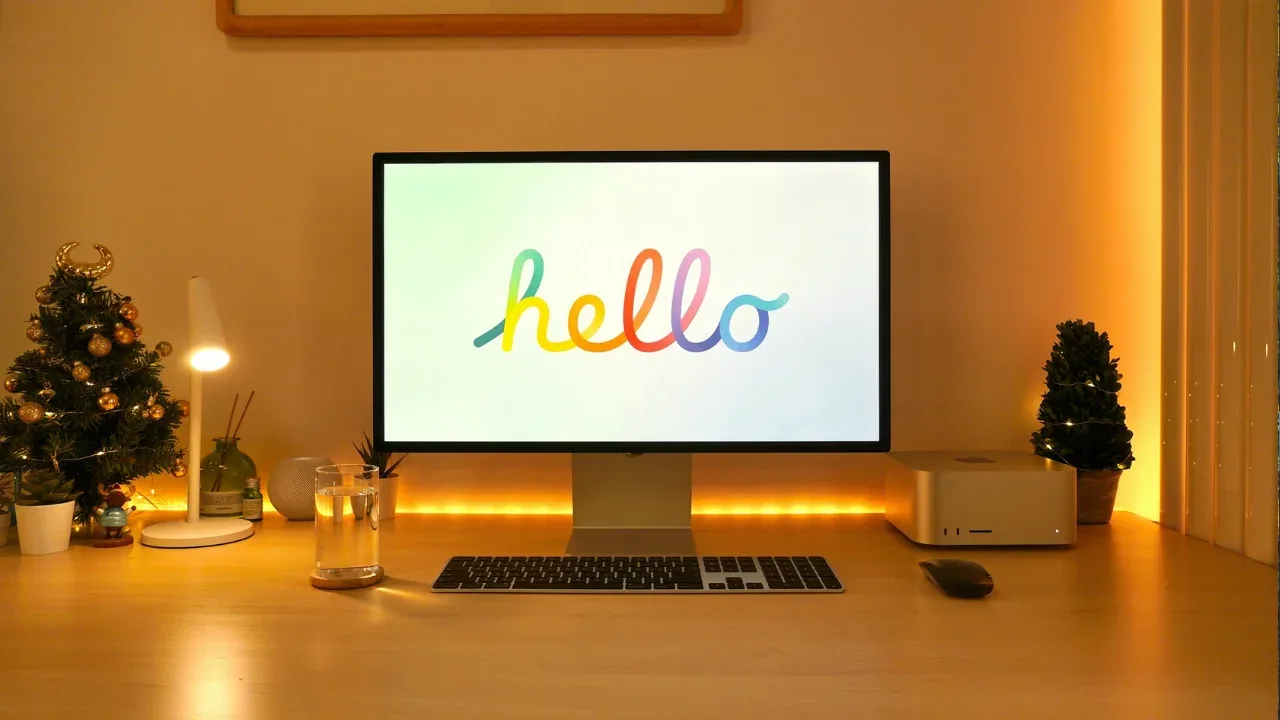
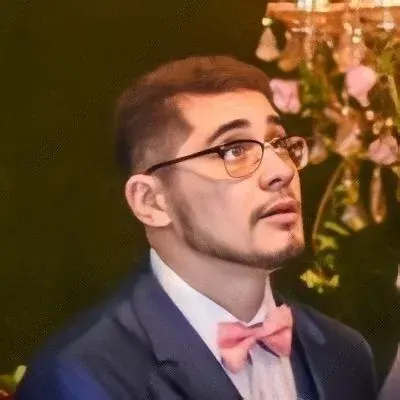
Adding a Placeholder on a CharField in Django: A Simple Guide
So, you've got a Django form, and you want to add a placeholder to one of the CharFields? No problemo! 🙌 In this guide, I'll walk you through the common issue of adding a placeholder and provide you with not just one, but two easy solutions. Let's dive in! 💻
The Problem Statement
Let's start with a simple example. Imagine you have a Django form called SearchForm
with a q
CharField. When this form is rendered in the template, you notice that the placeholder
attribute is missing. You want to add a placeholder to the q
field, with the value "Search". How can you accomplish this? 🤔
Solution 1: Modifying the Template
One way to address this issue is by modifying the template where you render the form. In your template file, you can manually add the placeholder
attribute to the input
tag. Here's how:
<input type="text" name="q" id="id_q" placeholder="Search" />
By directly adding the placeholder
attribute in the template, you can achieve the desired result. However, this approach may not be ideal if you have multiple forms in different templates and want to have consistent placeholder values. Fear not! We've got another solution for you. 😉
Solution 2: Using Widgets in Django Forms
Django provides a handy feature called widgets, which allow you to customize the way form fields are rendered. In our case, we can use a widget to add the desired placeholder. Here's how you can do it:
from django import forms
class SearchForm(forms.Form):
q = forms.CharField(label='search', widget=forms.TextInput(attrs={'placeholder': 'Search'}))
In this solution, we utilize the widget
parameter of the CharField
. We create a TextInput
widget and pass it a dictionary of attributes, in this case, the placeholder
attribute with the value "Search". Django takes care of rendering the field with the specified widget, including the placeholder attribute. 🎉
Which Solution to Choose?
Both solutions achieve the same result, so which one should you choose? Here are a few considerations for you to ponder:
If you want a consistent placeholder across multiple forms, modifying the template may require additional effort and consistency checks. In this case, using widgets would be a more systematic approach.
On the other hand, if you have only one form or a specific scenario where you don't require consistency, modifying the template might be the quicker and easier option.
Ultimately, the choice is yours! 😄
Conclusion
Adding a placeholder on a CharField in Django is now a piece of cake! 🍰 Whether you opt for modifying the template or utilizing widgets, you have the power to customize your form fields and enhance the user experience.
So, what are you waiting for? Go ahead and start beautifying your Django forms with eye-catching placeholders! And don't forget to share your cool implementations and thoughts in the comments below. Let's engage in a lively conversation! 🎉💬