How can I represent an "Enum" in Python?
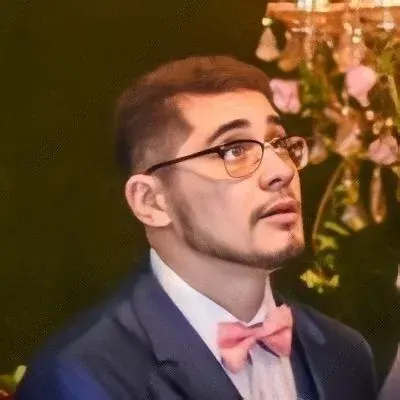
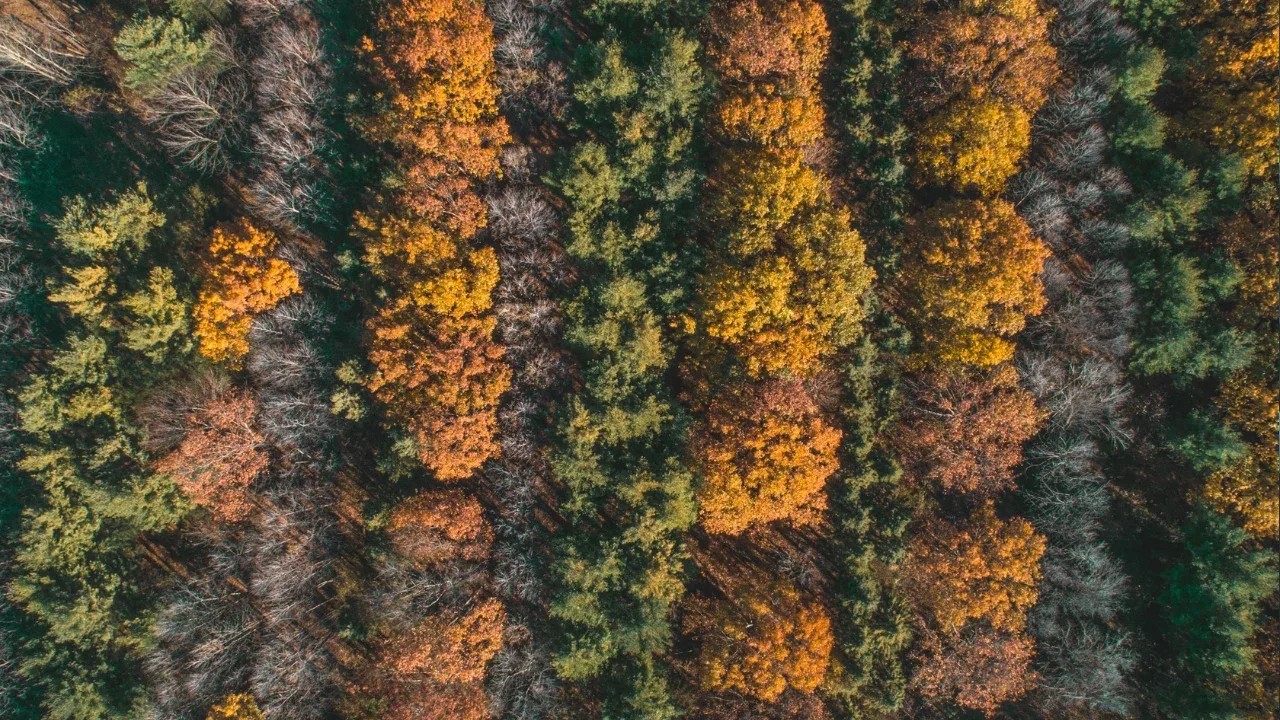
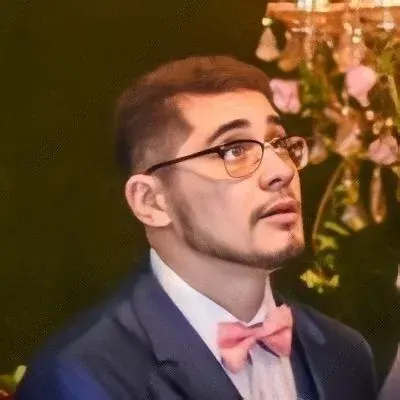
Representing an 'Enum' in Python 🐍
Are you a C# developer who has recently started working on a Python project? If so, you might be familiar with the concept of an Enum
in C# but wondering how to achieve a similar functionality in Python. Well, worry not! In this blog post, we will dive into the world of Python enums and explore how you can effectively represent them in your Python code. 🤓🚀
Understanding Enums in Python 🤔
In Python, an Enum
is a way to define a set of named values, much like in C#. They provide a concise and expressive way to represent a fixed number of possible values. Enums can be used for a variety of purposes, such as defining choices for a user, representing days of the week, or even representing different states in a game.
The enum
module in Python 📚
To define and work with enums in Python, we have the built-in enum
module. This module provides a class called Enum
that we can inherit from to define our own custom enums. Let's take a look at an example:
from enum import Enum
class Color(Enum):
RED = 1
GREEN = 2
BLUE = 3
In this example, we've defined a simple Color
enum with three values: RED
, GREEN
, and BLUE
. Each of these values is assigned an integer value, but you can also use strings or other types if desired. The values in an enum are unique, and you can access them using dot notation, just like you would in C#. For example:
print(Color.RED)
Output:
Color.RED
Enums also support iteration, membership testing, and comparing values. You can even convert an enum value to its corresponding name or value. 🔄
Enum-related challenges and easy solutions 💪
Challenge 1: Converting between Enum names and values
Sometimes, you might need to convert an enum name to its corresponding value, or vice versa. Fortunately, Python enums make this task straightforward:
# Converting enum names to values
print(Color['GREEN'].value) # Output: 2
# Converting enum values to names
print(Color(3).name) # Output: 'BLUE'
Challenge 2: Enum comparison using string values
If you want to check if two enum values are equal, you can directly compare them using the ==
operator. However, you might encounter challenges when comparing an enum value with a string. To overcome this, you can access the enum value using dot notation, like this:
if Color.RED.value == '1':
print("Enum value is equal to '1'")
Challenge 3: Enum iteration and string representation
To iterate over all the values of an enum or display them as strings, you can use the following techniques:
# Iterating over enum values
for color in Color:
print(color)
# Displaying enum values as strings
print([color.name for color in Color])
Your turn to explore! 🚀
Now that you have a basic understanding of Python enums and how to use them effectively, it's time to apply your knowledge in practice! Consider implementing enums in your current Python project and see how they enhance your codebase. Don't hesitate to experiment and explore the different possibilities that enums offer. 💡
If you have any questions or face any challenges while working with enums, feel free to leave a comment below. I'm here to help and guide you through any obstacles you encounter. Let's embrace the power of Python enums together! 💪💻
Happy coding! 😄✨
PS: If you found this blog post helpful, don't forget to share it with your fellow Python developers. Let's spread the knowledge and help others level up their Python skills! 🌟🐍
Subscribe here to stay updated with the latest Python tips and tricks! 📩🚀