How can I remove a key from a Python dictionary?
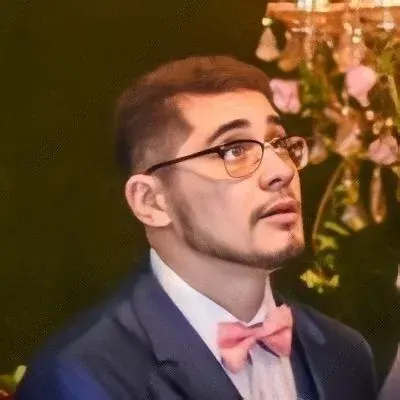
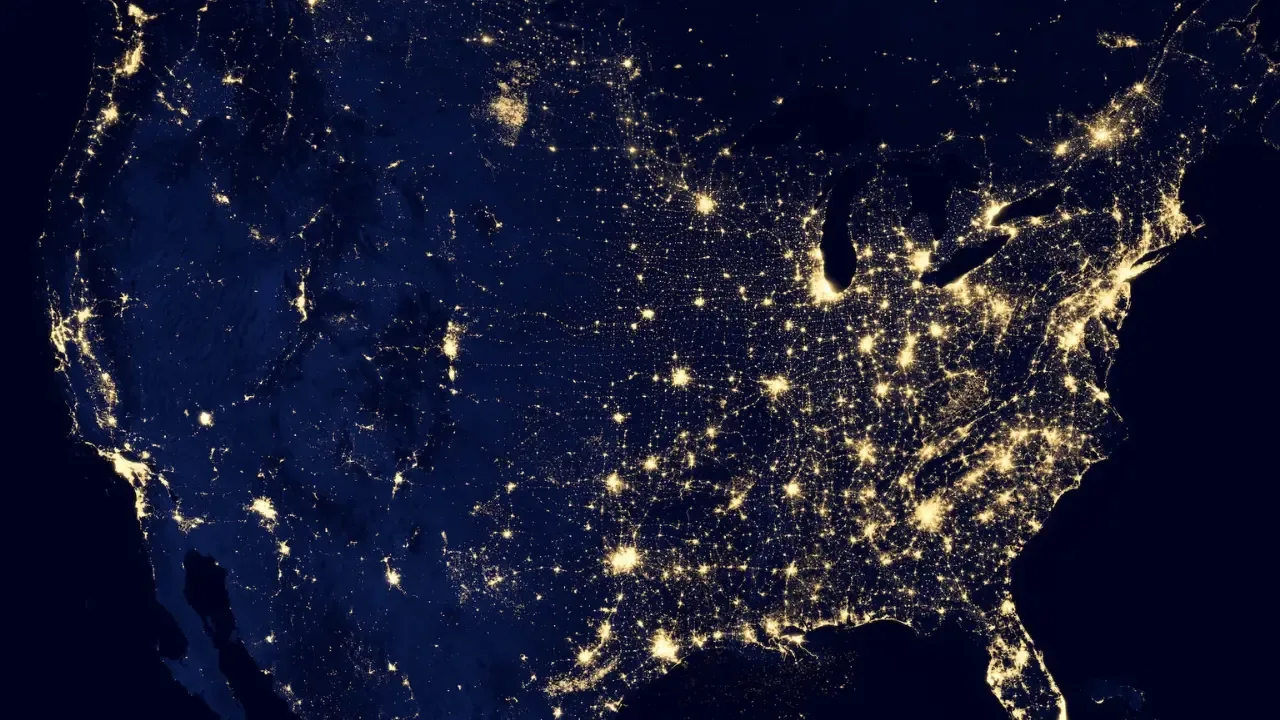
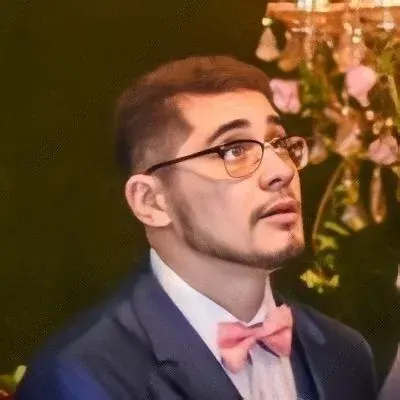
Removing a Key from a Python Dictionary: Simplified Solutions ✨💡
So, you want to gracefully remove a key from a Python dictionary, huh? That's a common challenge that many Python developers encounter. But fear not, my friend! I'm here to guide you through this process with simple and elegant solutions. Let's dive in! 🚀
The Initial Approach 🤔
Before we explore simpler alternatives, let's take a look at the initial code provided:
if key in my_dict:
del my_dict[key]
This code snippet checks if the key exists in the dictionary and then proceeds to delete it if it's present. While it gets the job done, it's not the cleanest or most concise solution. Plus, it requires an additional conditional check, increasing the code's complexity.
🎯 Simplified Solution: Using the pop()
method
Python's built-in pop()
method is a handy way to remove a key from a dictionary while also handling the case when the key is not present. Let's take a look at how it works:
my_dict.pop(key, None)
Voilà! With just this single line of code, you can elegantly remove the key from the dictionary, even if it doesn't exist! If the key is present, its corresponding value is returned and removed from the dictionary. If the key is not found, the pop()
method returns None
by default, ensuring that no KeyError
is raised.
🌟 A Real-World Example
To better illustrate the power of the pop()
method, let's consider a practical scenario. Imagine you have a dictionary called fruit_stock
, which keeps track of the available quantity for each fruit in your grocery store. And you want to remove the key-value pair for apples from the dictionary:
fruit_stock = {"bananas": 10, "apples": 5, "oranges": 8}
removed_quantity = fruit_stock.pop("apples", 0)
print(removed_quantity) # Output: 5
print(fruit_stock) # Output: {"bananas": 10, "oranges": 8}
In this example, removed_quantity
stores the value corresponding to the "apples" key, which is 5. After removing the key-value pair, the updated fruit_stock
dictionary contains only the remaining fruits and their quantities.
💬 Engage with the Tech Community
If you found these solutions helpful or have any further questions, let's keep the conversation going! Join our tech community on Stack Overflow and share your experiences with removing keys from dictionaries. We love hearing from fellow developers, and your insights might help someone else facing a similar challenge!
Feel free to follow this link to the dedicated Stack Overflow thread for more in-depth approaches: Delete an element from a dictionary.
Now that you have the power to gracefully remove keys from dictionaries, go forth and code with confidence! 💪💻
Remember, simplicity is key (pun intended!) in every aspect of coding. Happy coding, fellow Pythonistas! 🐍✨