How can I read inputs as numbers?
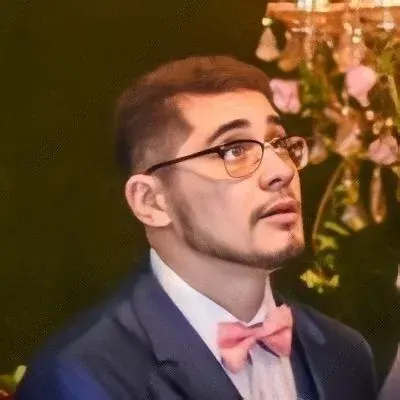
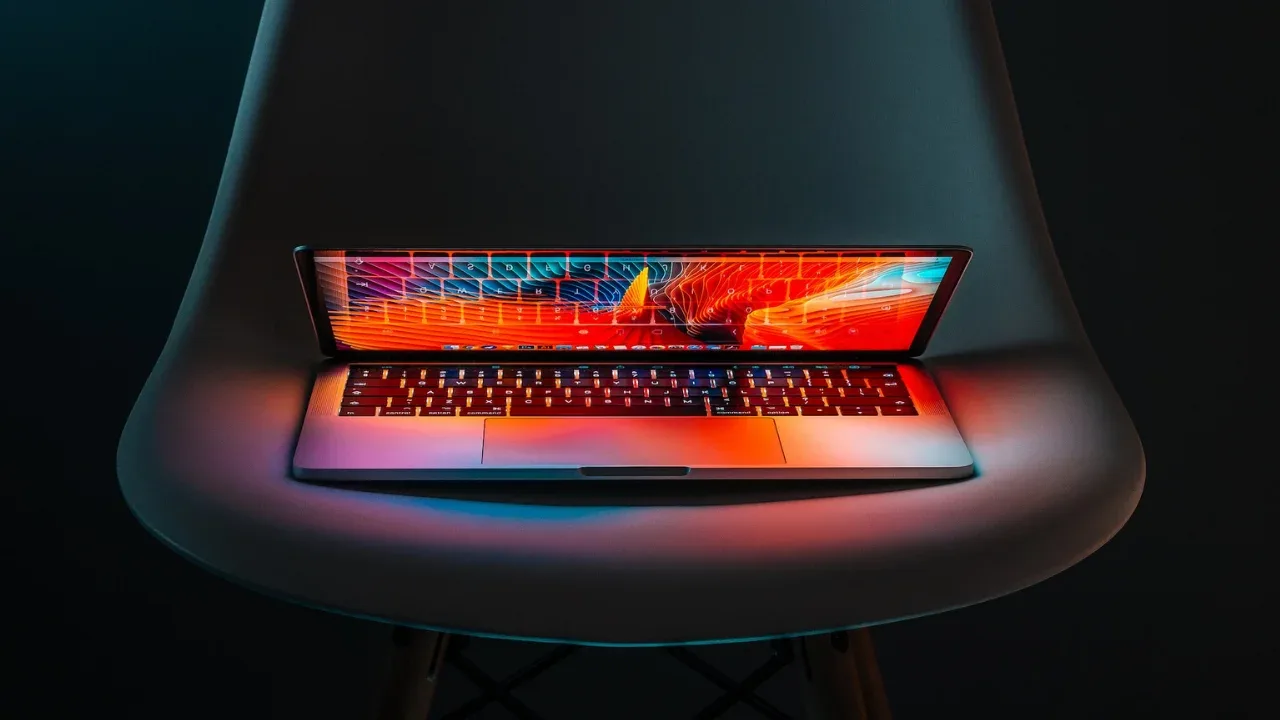
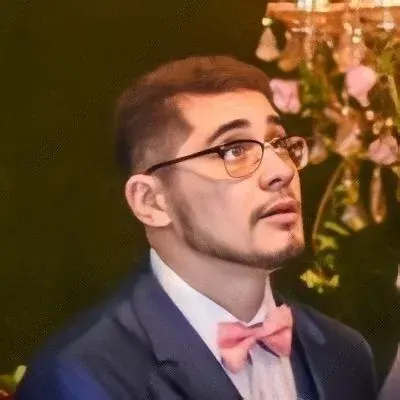
🔢 How can I read inputs as numbers? 🤔
Hey there tech enthusiasts! 👋 Are you scratching your head wondering why x
and y
are coming out as strings instead of ints in the code snippet above? 😖 Well, worry not, because I'm here to break it down for you and provide easy solutions! 🙌
So, let's dive into the problem... 🏊♂️
The reason why x
and y
are strings in this code is because the input()
function in Python always returns a string by default. 😮 In Python 2.x, there was a distinction between input()
and raw_input()
, where the former evaluated user input as code and the latter returned a string. However, in Python 3.x, input()
replaced the functionality of raw_input()
and now always returns a string. 🐍
Now that we understand why the inputs are strings, let's look at some easy solutions! 💡
Convert the inputs to integers with the
int()
function 🔄The simplest way to convert strings to integers is by using the built-in
int()
function. You can modify the code as follows:play = True while play: x = int(input("Enter a number: ")) y = int(input("Enter a number: ")) print(x + y) print(x - y) print(x * y) print(x / y) print(x % y) if input("Play again? ") == "no": play = False
By wrapping the
input()
function withint()
, the user inputs will be converted to integers. 🎉Validate user inputs to ensure they are numbers ✅
Another approach is to validate inputs to make sure they are numeric. You can use a
try-except
block to catch any potentialValueError
if a user enters a non-numeric input. Here's an example:play = True while play: try: x = int(input("Enter a number: ")) y = int(input("Enter a number: ")) print(x + y) print(x - y) print(x * y) print(x / y) print(x % y) except ValueError: print("Oops! Invalid input. Please enter a number.") if input("Play again? ") == "no": play = False
This way, if a user enters a non-numeric value, the code will handle the error gracefully and prompt them to enter a valid number. 🚫🔢
Alright, fellow tech enthusiasts! I hope these solutions have helped you understand how to read inputs as numbers. Now it's your turn to put these tips into practice and enhance your Python coding skills! 🚀
If you have any questions or other cool coding topics you'd like me to cover, feel free to drop a comment below! Let's keep the conversation going and encourage each other in our coding journeys. 💬😊