How can I read a text file into a string variable and strip newlines?
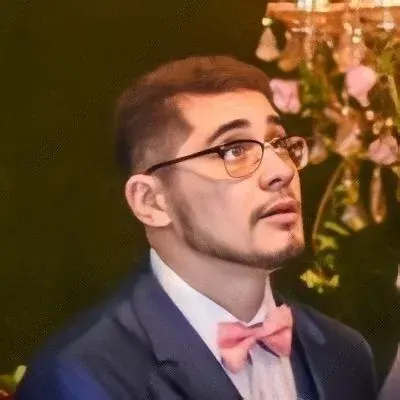
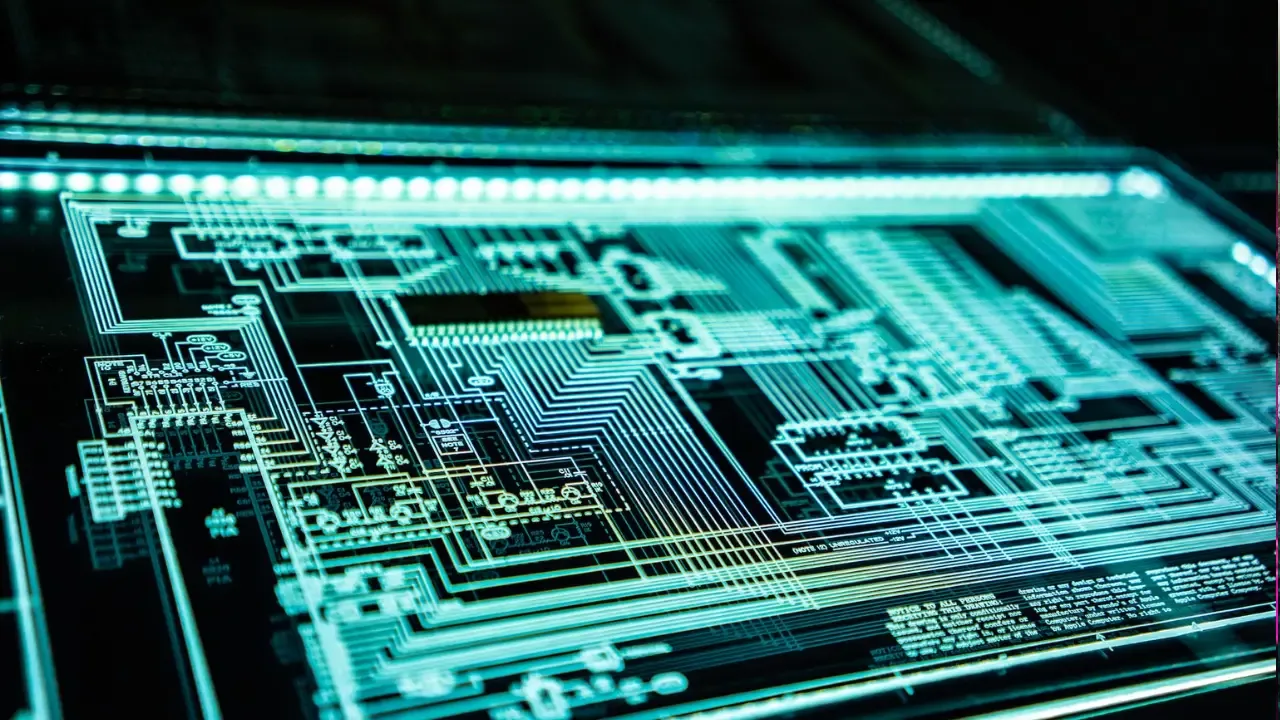
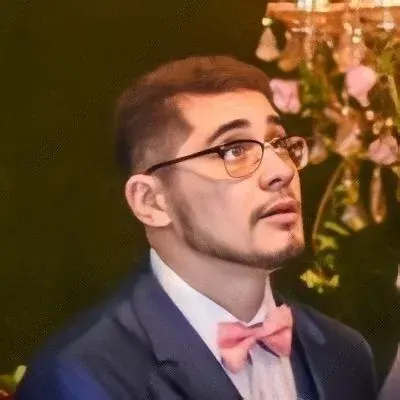
How to Read a Text File into a String Variable and Strip Newlines
Have you ever struggled with reading a text file into a string variable and removing those pesky newlines? 📄💔 Don't worry, I've got you covered! In this guide, I'll show you a couple of easy ways to solve this problem, so you can focus on the important stuff. Let's dive right in! 💪
The Challenge
Here's the scenario: you have a text file with multiple lines, and you want to read it into a single-line string, removing all the newlines. Let's say your file looks like this:
ABC
DEF
You want to transform it into a string like this: 'ABCDEF'
👍
Solution 1: Using file.read()
and str.replace()
One straightforward way to achieve this is by using Python's built-in file
object's read()
method and the str.replace()
method. Here's how you can do it:
with open('your_file.txt', 'r') as file:
text = file.read().replace('\n', '')
Let's break it down:
Open the file using the
open()
function and thewith
statement to ensure it's properly closed afterward.Use the
read()
method to read the entire contents of the file into a string.Apply the
replace()
method to replace all newline characters (\n
) with an empty string.
And voilà! You now have the text file loaded into the text
variable without any newlines. 🎉
Solution 2: Using file.readlines()
and str.join()
Another way to tackle this problem is by using the readlines()
method to read the file into a list of lines, and then using join()
to concatenate those lines into a single string. Here's the code:
with open('your_file.txt', 'r') as file:
lines = file.readlines()
text = ''.join(lines).replace('\n', '')
Here's how it works:
Open the file using
open()
and the trustywith
statement.Use
readlines()
to read the contents of the file into a list of lines.Use
join()
to concatenate those lines without any separator, effectively creating a single string.Finally, use
replace()
to remove any remaining newline characters.
Now you have the same desired result: the content of the text file stored in the text
variable without any newlines. 🎉
Wrapping Up
Dealing with newlines when reading a text file into a string variable can be a minor but annoying challenge. 😩 Fortunately, there are simple solutions available to save the day!
You can try the first solution, using file.read()
and str.replace()
, if you prefer a more concise and direct approach. Alternatively, the second solution, using file.readlines()
and str.join()
, gives you more flexibility if you need to perform further operations with the lines in the file.
So go ahead, try these solutions and save yourself some coding headaches! 😄👏
If you have any questions or want to share your own tips, feel free to leave a comment below. Happy coding! 💻🚀