How can I randomly select (choose) an item from a list (get a random element)?
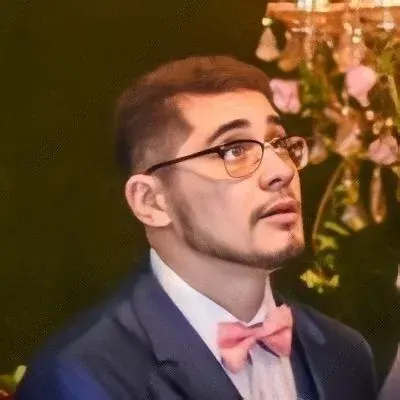
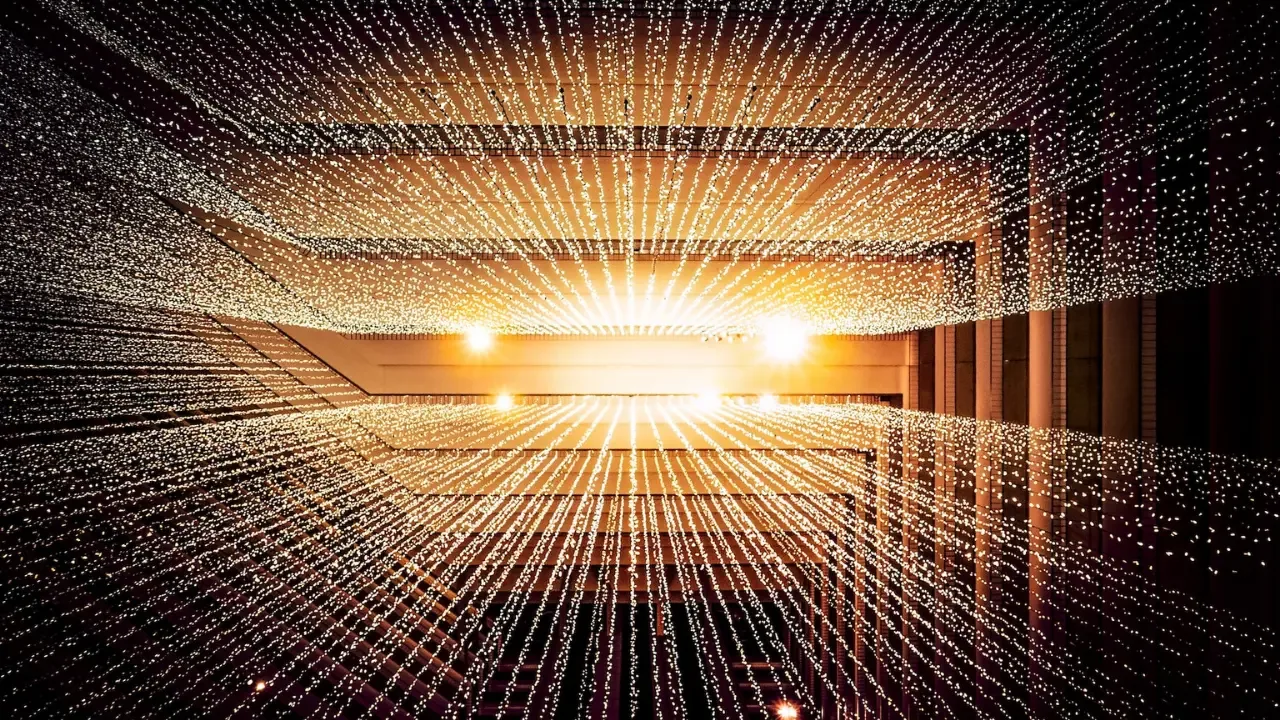
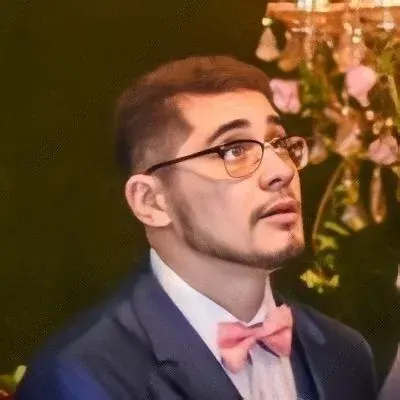
🎲📝💡
How to Randomly Select an Item from a List in Python 🐍🔀
Do you find yourself struggling to select a random item from a list in Python? 😕 Fear not! In this guide, we'll explore some common issues and provide easy solutions to help you choose a random element effortlessly. Let's get started! 🚀
The Problem: Selecting a Random Item from a List
The question you've stumbled upon is a common one: "How do I retrieve an item at random from the following list?" 🤔 The original code snippet looks like this:
foo = ['a', 'b', 'c', 'd', 'e']
Solution 1: Using Python's random
Module 🎯
Thankfully, Python provides a built-in module called random
that helps us tackle this problem seamlessly. Here's the code to achieve our goal of choosing a random element from the foo
list:
import random
random_element = random.choice(foo)
Voila! With just two lines of code, you can now retrieve a random item from your list. How cool is that? 😎
Solution 2: Using Numpy's random
Module 🌈
If you're comfortable with additional libraries, you can also leverage Numpy's random
module. Here's how you can modify your code to use it:
import numpy as np
random_element = np.random.choice(foo)
Using Numpy's random
module provides you with additional functionalities if you need advanced randomization techniques. It's worth exploring if you're feeling adventurous! 🌟
Solution 3: Manually Randomizing the Index 🎲
If you prefer a more hands-on approach, you can manually randomize the index of your list and retrieve the corresponding element. Here's how you can achieve this:
import random
random_index = random.randint(0, len(foo) - 1)
random_element = foo[random_index]
This method might be useful in certain scenarios where you need more control over the randomization process. Give it a try if it suits your requirements! 💪
Conclusion and Call-to-Action
Selecting a random item from a list doesn't have to be a daunting task anymore! 😄 In this guide, we explored three easy solutions using different modules in Python. Whether you choose to stick with Python's built-in random
module, explore Numpy's additional functionalities, or manually randomize the index of your list, the choice is yours! 🥳
Now that you know how to easily select a random element from a list, why not put your newfound knowledge to the test? Give it a try in your next project and let us know how it goes in the comments below! 🚀💬
Remember, happy coding and stay random! 🔀💻