How can I print multiple things (fixed text and/or variable values) on the same line, all at once?
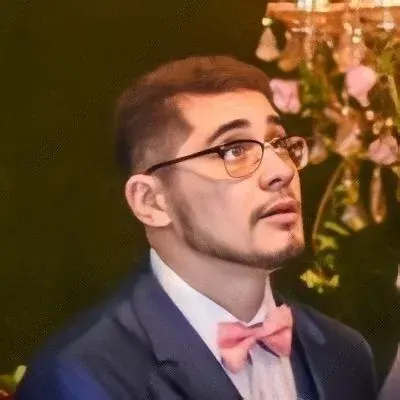
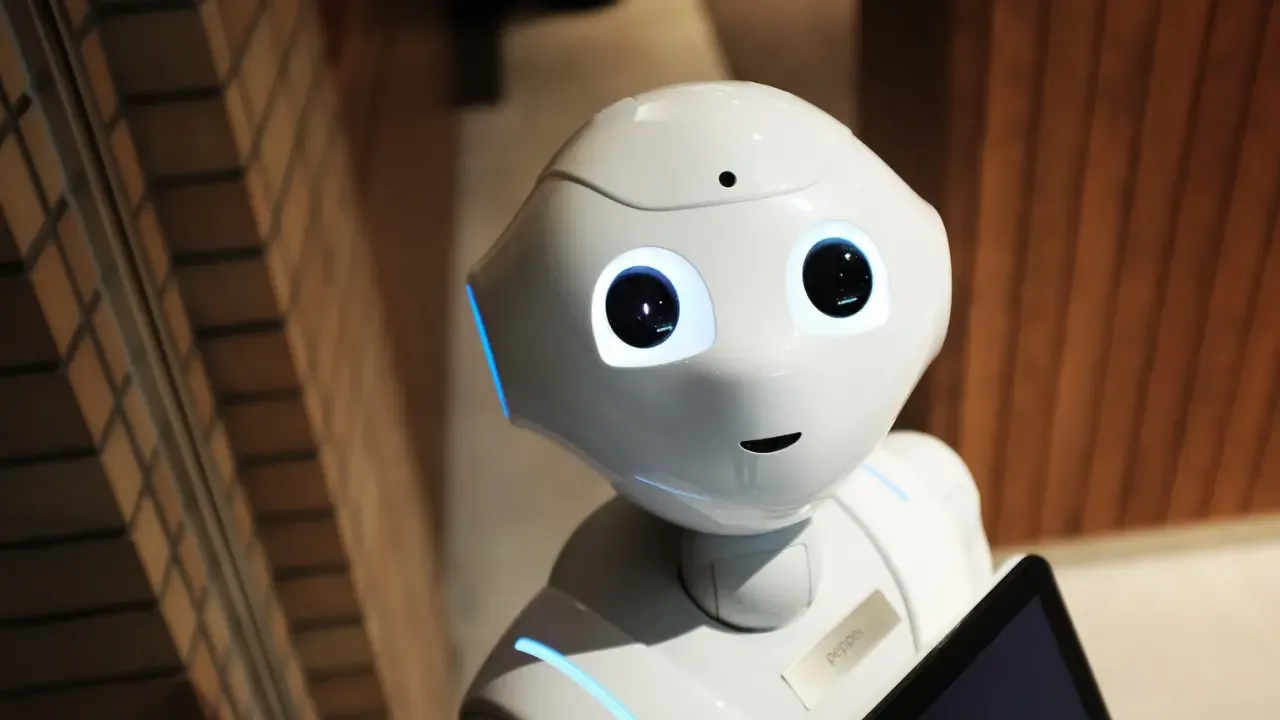
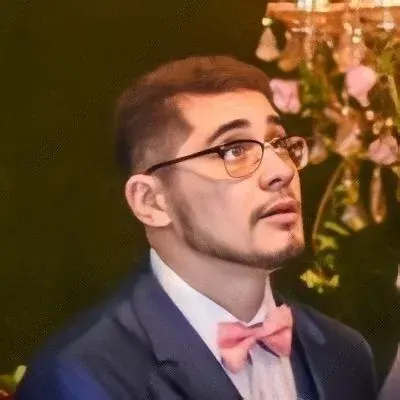
How to Print Multiple Things on the Same Line in Python 🖨️
Are you tired of getting your print statements all messed up? Do you want to print multiple things on the same line in Python, including fixed text and variable values, without ending up with a jumbled mess? Look no further! In this guide, we'll walk you through the common issues and easy solutions to get your print statements in order. Let's dive right in! 💪
The Problem 🤔
Let's consider the following code snippet:
score = 100
name = 'Alice'
print('Total score for %s is %s', name, score)
When you run this code, instead of getting the desired output of "Total score for Alice is 100"
, you end up with "Total score for %s is %s Alice 100"
. What went wrong? How can you get everything to print in the right order with the right formatting? Let's find out! 🧐
The Solution 💡
The issue here is that you are using the wrong syntax to format your string. In Python, you can use the string interpolation method .format()
or the more modern f-strings to achieve the desired result.
Option 1: Using .format()
print('Total score for {} is {}'.format(name, score))
By using {}
as placeholders in the string and using the .format()
method, you can pass the values of name
and score
in the right order.
Option 2: Using f-strings (Python 3.6+)
print(f'Total score for {name} is {score}')
With f-strings, you can directly embed the variables within the string using the {variable_name}
syntax. This makes the code more readable and easier to work with.
Try it Yourself! 👩💻👨💻
Now that you know the correct syntax, it's time to give it a try! Take the code snippet we discussed earlier, replace it with one of the solutions mentioned above, and run it again. You should now see the desired output: "Total score for Alice is 100"
🎉
But wait, we're not done yet! There's more to learn and explore. So, whether you're struggling with printing multiple things on the same line or you want to dive deeper into Python string formatting techniques, don't hesitate to reach out.
Let's Connect! 🌐
We hope this guide helped you solve the problem and improve your Python skills. If you found it useful, feel free to share it with your friends and colleagues. And while you're at it, why not subscribe to our newsletter? 📧 We regularly publish helpful content to level up your coding game and keep you up-to-date with the latest tech trends.
Got any more questions or need further assistance? Drop a comment below, and let's start a conversation! Happy coding! 🚀