How can I parse (read) and use JSON in Python?
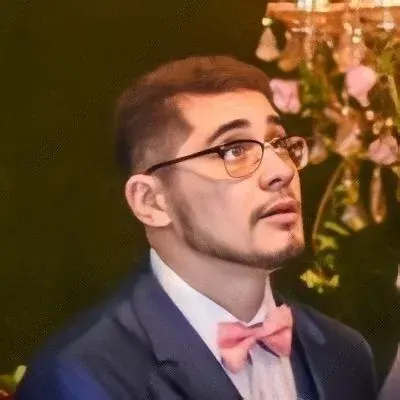
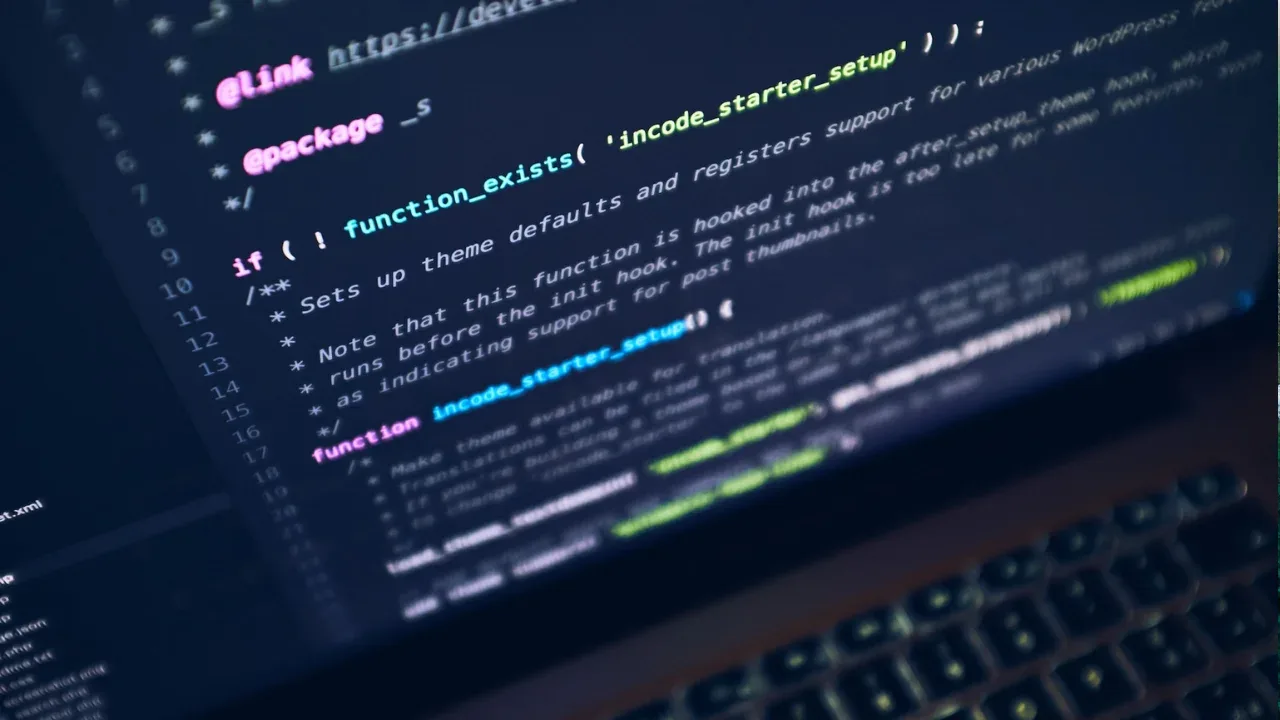
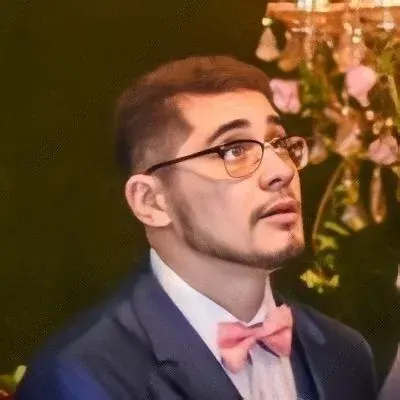
How to Parse and Use JSON in Python 🐍
So you've received some JSON data in your Python program, and now you want to extract specific information from it. 🤔 Don't worry, I got your back! Parsing and using JSON in Python is actually quite simple. Let me show you how! 😎
Step 1: Import the json
Module
Before we can start parsing JSON, we need to import the json
module in our Python script. This module provides us with the necessary tools and functions to work with JSON data. Here's how you can import it:
import json
Step 2: Use json.loads
to Parse JSON
To parse JSON, we'll use the json.loads
function. This function takes a JSON-formatted string as input and returns a Python object representing the JSON data. Let's say we have the following JSON string:
jsonStr = '{"one": "1", "two": "2", "three": "3"}'
To parse this JSON string, we can use the json.loads
function like this:
jsonData = json.loads(jsonStr)
Now, jsonData
will be a Python dictionary containing the parsed JSON data. Easy, right? 😉
Step 3: Accessing Specific Data
Now that we have parsed the JSON and stored it in a Python dictionary, we can easily access specific data using the keys. In your example, you want to retrieve the value corresponding to the key "two". Here's how you can do it:
value = jsonData["two"]
The variable value
will now contain the value "2". You can replace "two" with any other key to retrieve different values from the JSON data.
Common Issues and their Solutions
Issue 1: Confusing .load
and .loads
Sometimes, people get confused between the .load
and .loads
functions in the json
module. Remember, .load
is used for reading JSON data from a file, while .loads
is used to parse JSON data from a string. In your case, since you have a JSON string, make sure you use .loads
.
Issue 2: Non-JSON data
Not all data that looks like JSON is actually valid JSON. It might be the result of applying repr
to native Python data structures or have other formatting issues. In such cases, you'll need to use additional techniques to parse the data correctly. Check out the resources provided in the original question for more information on handling these scenarios.
Issue 3: JSONL - JSON in Each Line
If your JSON data is formatted with each JSON object on a separate line, you're dealing with a format called JSONL (JSON Lines). Since proper JSON cannot be parsed line by line, you'll need to handle this format differently. Again, refer to the resources mentioned in the original question for guidance on loading JSONL files as JSON objects.
Conclusion
Parsing and using JSON data in Python is a fundamental skill that will help you work with APIs, web services, and various data sources. By following these simple steps, you can easily extract the information you need from JSON data. 🚀
So go ahead, give it a try, and unlock the power of JSON in your Python projects! If you have any further questions or want to share your experiences, feel free to leave a comment below. Happy coding! 💻💪