How can I open an Excel file in Python?
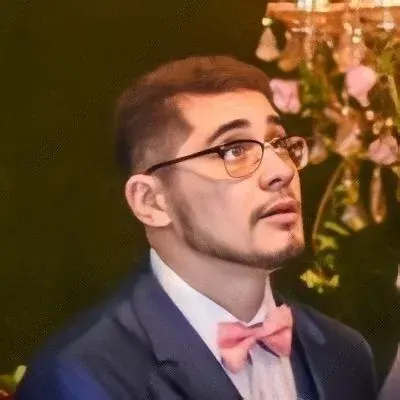
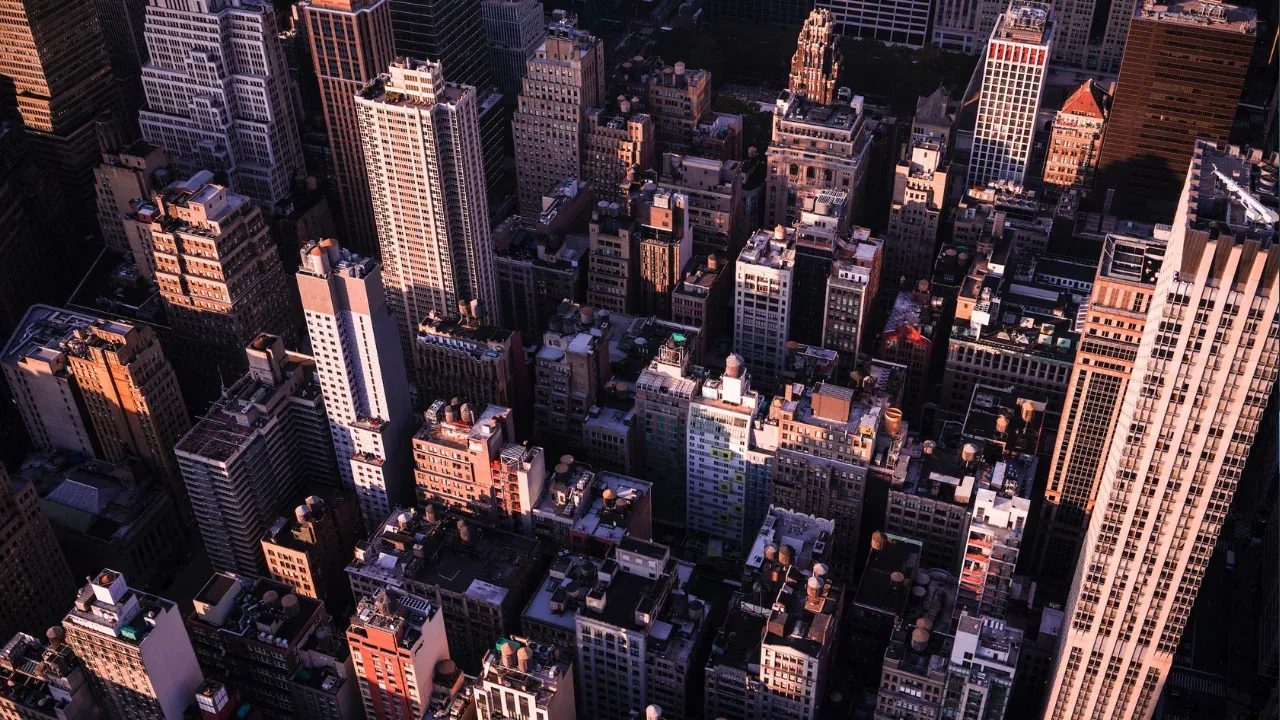
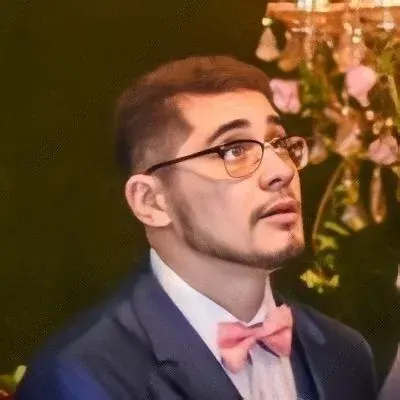
How to Open an Excel File in Python? πππ
So, you're an aspiring Python programmer who wants to open an Excel file? π€ No worries, I've got your back! In this blog post, I'll explain how you can easily read an Excel file using Python and provide some handy solutions to common issues along the way. Let's dive in! πͺ
The Challenge: Opening an Excel File in Python π
Opening simple text files in Python is a piece of cake. You just use the open()
function, specify the file name with its extension (e.g., sometextfile.txt
), and choose the file mode. However, when it comes to Excel files, things get a bit trickier. π€¨
The Excel Heroes: Pandas and Openpyxl π¦ΈββοΈπ¦ΈββοΈ
Fortunately, Python offers powerful libraries that make working with Excel files a breeze. The two most commonly used libraries for this task are:
Pandas πΌ: A versatile data manipulation library that provides a variety of functions and tools for working with data, including reading and writing Excel files.
Openpyxl π: A dedicated library specifically designed to handle Excel files. It allows you to read, write, and modify Excel worksheets with ease.
Solution 1: Using Pandas' read_excel() Function π
import pandas as pd
df = pd.read_excel('myexcelfile.xlsx')
print(df.head())
By using the read_excel()
function from the Pandas library, you can read the contents of an Excel file into a DataFrame. Easy peasy! Just make sure you have the Pandas library installed (pip install pandas
) before running the above code.
Solution 2: Harnessing the Power of Openpyxl π
If you prefer a more direct approach using the Openpyxl library, here's how you can achieve it:
from openpyxl import load_workbook
wb = load_workbook('myexcelfile.xlsx')
ws = wb.active
for row in ws.iter_rows():
for cell in row:
print(cell.value)
In this approach, we first load the Excel file using load_workbook()
, and then access the active worksheet (wb.active
). From there, you can loop through the rows and cells to access individual values.
Common Issues and Troubleshooting π οΈ
Issue 1: "ModuleNotFoundError: No module named 'pandas'" π«
If you encounter this issue, it means that the Pandas library is not installed. You can easily resolve it by running the following command:
pip install pandas
Issue 2: "FileNotFoundError: [Errno 2] No such file or directory: 'myexcelfile.xlsx'" ππ«
This error occurs when the provided file path is incorrect. Make sure to double-check the file name, extension, and location. If the file is in a different directory, you need to provide the full file path instead of just the file name.
Your Turn: Get Hands-on with Excel in Python! π»π’
Now that you have the tools and solutions at your disposal, it's time to unleash your creativity and explore the wonders of working with Excel in Python! π
Give it a try! Open an Excel file using either the Pandas or Openpyxl approach, and experiment with different operations like filtering, sorting, or analyzing the data. The possibilities are endless! π
Share your experience and any cool Excel manipulations you discover in the comments below. Let's inspire each other and learn together!
Happy Pythoning! πβ€οΈ
(Image Source: Unsplash.com)