How can I obtain the element-wise logical NOT of a pandas Series?
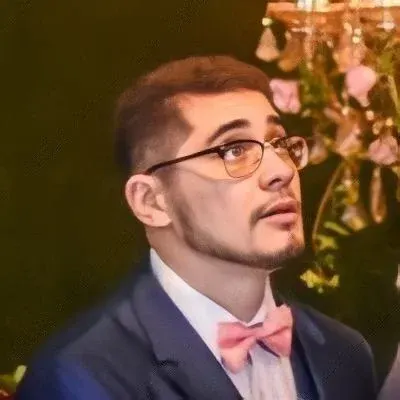
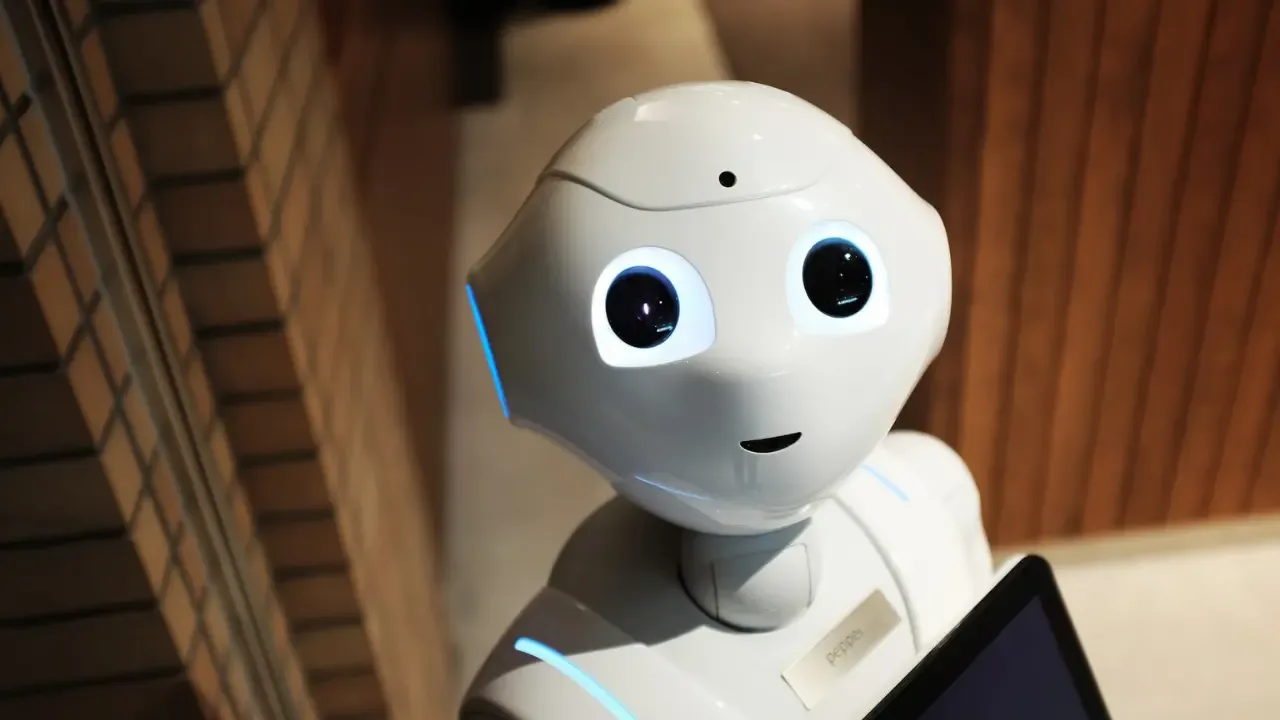
How to Obtain the Element-Wise Logical NOT of a Pandas Series 😕
So, you have a pandas Series
object consisting of boolean values, and you want to obtain another series that contains the logical NOT of each value? Fear not, my friend! I'm here to help you regain your mojo and solve this problem in a jiffy 🚀
The Challenge
Let's take a look at the series you have:
True
True
True
False
And the desired output:
False
False
False
True
Your goal is to convert each True
value to False
and vice versa, while keeping the series intact and preserving the order of elements.
The Solution
To achieve this, we can utilize the ~
operator in combination with the pandas Series
object. The ~
operator behaves as the logical NOT operator and negates each element of the series.
Here's the magical one-liner that will transform your series into what you desire:
inverted_series = ~original_series
Yes, it's really that simple! 😎
By applying the ~
operator to your original series, you obtain a new series where each True
value becomes False
, and each False
value becomes True
.
Let's Put It All Together
In our case, the solution would be:
import pandas as pd
original_series = pd.Series([True, True, True, False])
inverted_series = ~original_series
print(inverted_series)
Output:
0 False
1 False
2 False
3 True
dtype: bool
Voila! You now have your element-wise logical NOT series ready to go 🎉
Feel free to incorporate this solution into your pandas projects and let the magic unfold!
Challenges and Pitfalls
While obtaining the logical NOT of a pandas series is relatively straightforward, there are a couple of things to keep in mind:
Ensure that your series consists only of boolean values. If your series contains non-boolean elements, the logical NOT operation will throw an error. Make sure to convert or filter your series accordingly.
Pay attention to the data type of your series after applying the logical NOT operation. In our example, the resulting series has a data type of
bool
. Depending on your use case, you might need to convert the series to a different data type for further processing.
Your Turn to Shine ✨
Now that you know how to obtain the element-wise logical NOT of a pandas series, it's time for some action! Head over to your code editor and give it a try. If you face any challenges or have any exciting use cases to share, don't hesitate to reach out in the comments below.
Remember, the best way to learn is by doing, so don't be shy and unleash your newfound knowledge!
Happy coding! 🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
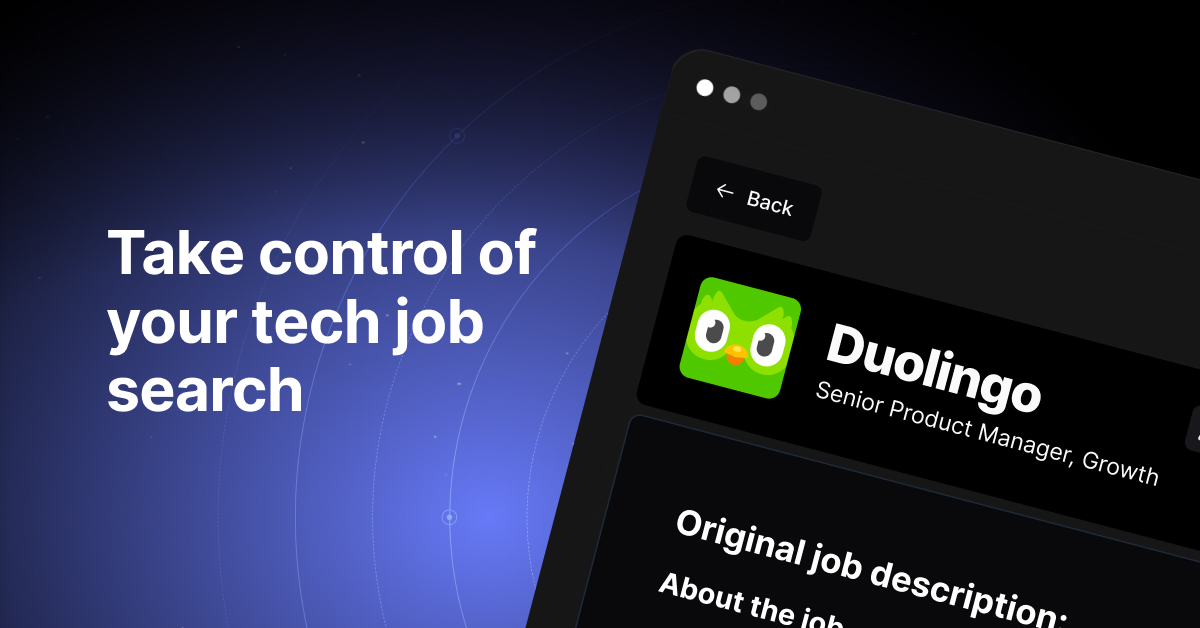