How can I map True/False to 1/0 in a Pandas DataFrame?
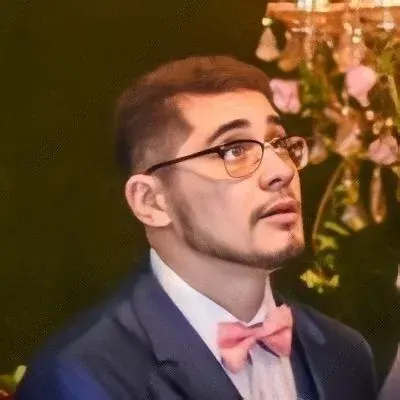
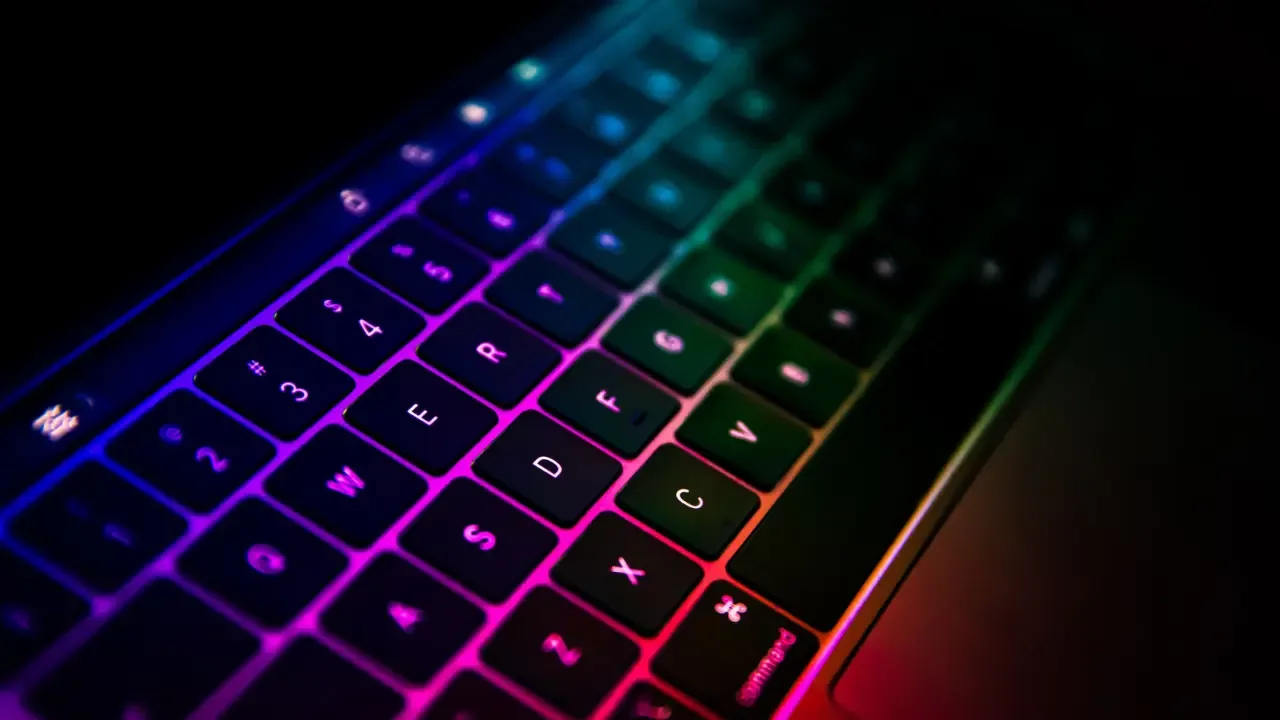
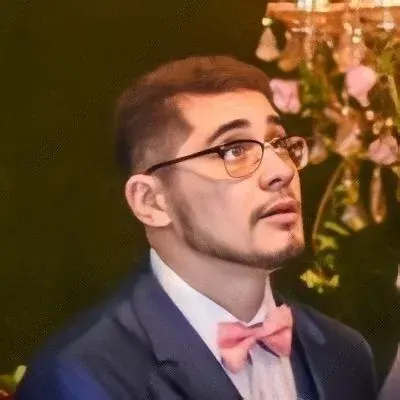
📝💡 How to Map True/False to 1/0 in a Pandas DataFrame: A Quick Guide
Are you working with a Pandas DataFrame that contains boolean values but need to convert them to 1s and 0s for further calculations? Look no further! In this blog post, we will explore a quick and efficient way to map True/False to 1/0 in a Pandas DataFrame using the power of pandas and numpy.
🔍 Understanding the Problem: You have a column in your Pandas DataFrame with boolean values (True/False). However, your analysis or calculations require them to be represented as 1s and 0s. Converting these boolean values is a common task, and luckily, there's an easy solution!
⏱️ Quick and Easy Solution: To convert True and False to 1 and 0, you can leverage the powerful and versatile pandas and numpy libraries. Here's how:
1️⃣ Import the necessary libraries:
import pandas as pd
import numpy as np
2️⃣ Let's assume your DataFrame is named df
and the column you want to convert is named boolean_column
. You can use the .astype()
method and pass int
as the argument to convert the boolean values to integers:
df['boolean_column'] = df['boolean_column'].astype(int)
That's it! 🎉 Now your True/False values in boolean_column
will be mapped to 1s and 0s, allowing you to perform further calculations or analysis effortlessly.
Here's an example:
import pandas as pd
import numpy as np
# Create a sample DataFrame
data = {'fruit': ['apple', 'banana', 'orange'],
'in_stock': [True, False, True]}
df = pd.DataFrame(data)
# Convert True/False to 1/0
df['in_stock'] = df['in_stock'].astype(int)
print(df)
Output:
fruit in_stock
0 apple 1
1 banana 0
2 orange 1
💡 Pro Tip:
If you have multiple boolean columns in your DataFrame or want to convert multiple columns, you can pass a list of column names to the .astype()
method. For example:
boolean_columns = ['column1', 'column2', 'column3']
df[boolean_columns] = df[boolean_columns].astype(int)
📣 Call-to-Action: Now that you know the quick and easy way to map True/False to 1/0 in a Pandas DataFrame, go ahead and try it out in your own analysis or calculations. Leave a comment below to share your experience or any other tips you might have. Happy coding! 😄💻