How can I make a dictionary (dict) from separate lists of keys and values?
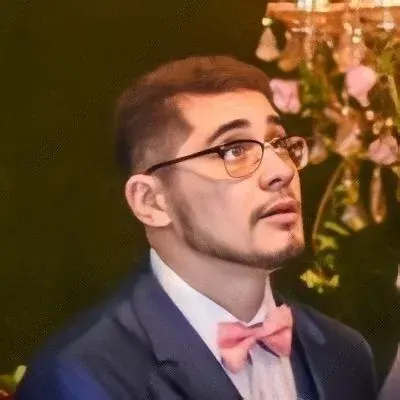
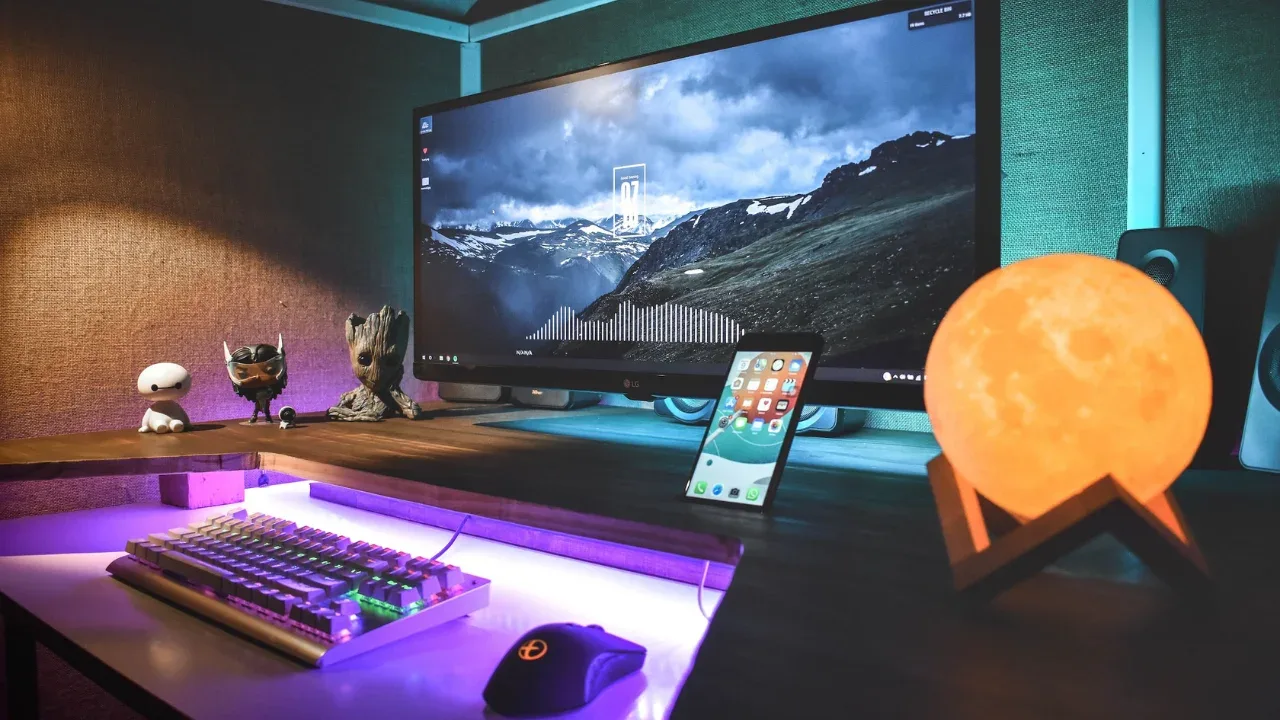
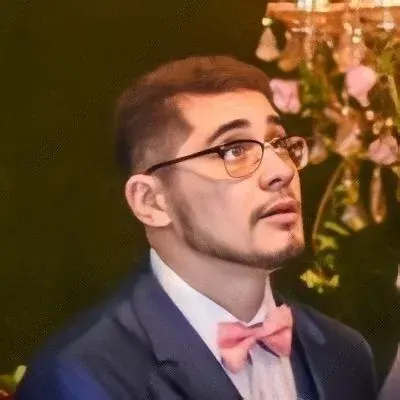
Title: 📚 Creating a Dictionary from Separate Lists in Python
Are you tired of manually assembling dictionaries from separate lists of keys and values? 😩 Well, fret no more! In this blog post, we'll show you how to effortlessly combine those lists into a single dictionary using Python. 🐍💡
The Challenge
Let's consider a scenario where we have two separate lists: keys
and values
. Our goal is to create a dictionary by pairing each element from the keys
list with its corresponding element from the values
list.
keys = ['name', 'age', 'food']
values = ['Monty', 42, 'spam']
The Solution 💡🔀
To achieve this, we can utilize Python's built-in zip()
function and a dictionary comprehension. The zip()
function takes multiple iterables as arguments and aggregates them into tuples. By passing the keys
and values
lists to zip()
, we can create a list of tuples:
combined = zip(keys, values)
Now, we can utilize a dictionary comprehension to construct our final dictionary by iterating over the combined
list and assigning each tuple's first element as the key and the second element as the value:
dictionary = {k: v for k, v in combined}
🎉 And voila! We have successfully created our desired dictionary:
{'name': 'Monty', 'age': 42, 'food': 'spam'}
Handling Unequal Lengths ⚖️
It's important to note that if the keys
and values
lists have different lengths, the resulting dictionary will only contain key-value pairs up to the length of the shortest list. This is because zip()
stops pairing elements once it reaches the end of the shortest list.
For example, if we have the following lists:
keys = ['name', 'age', 'food']
values = ['Monty', 42]
The resulting dictionary will be:
{'name': 'Monty', 'age': 42}
📢 Your Turn!
Now, it's time for you to put your newfound knowledge into action! 🚀 Try combining different lists and create dictionaries using the zip()
function and dictionary comprehension. Experiment with lists of varying lengths and see how the resulting dictionaries are affected.
Don't be shy to share your creative solutions, insights, or any questions you might have in the comments section below! Let's dive into the exciting world of dictionary creation and sharing our learnings. 📝💬
So go forth and conquer the challenge of combining separate lists into a powerful dictionary! 😎🔑
Happy coding! 💻🎉