How can I import a module dynamically given the full path?
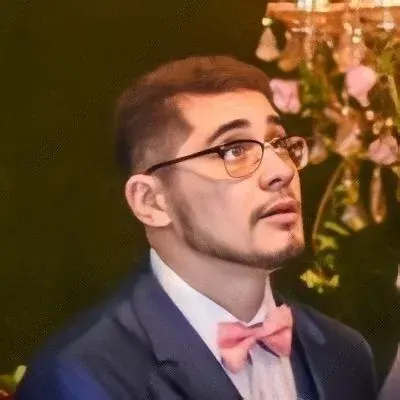
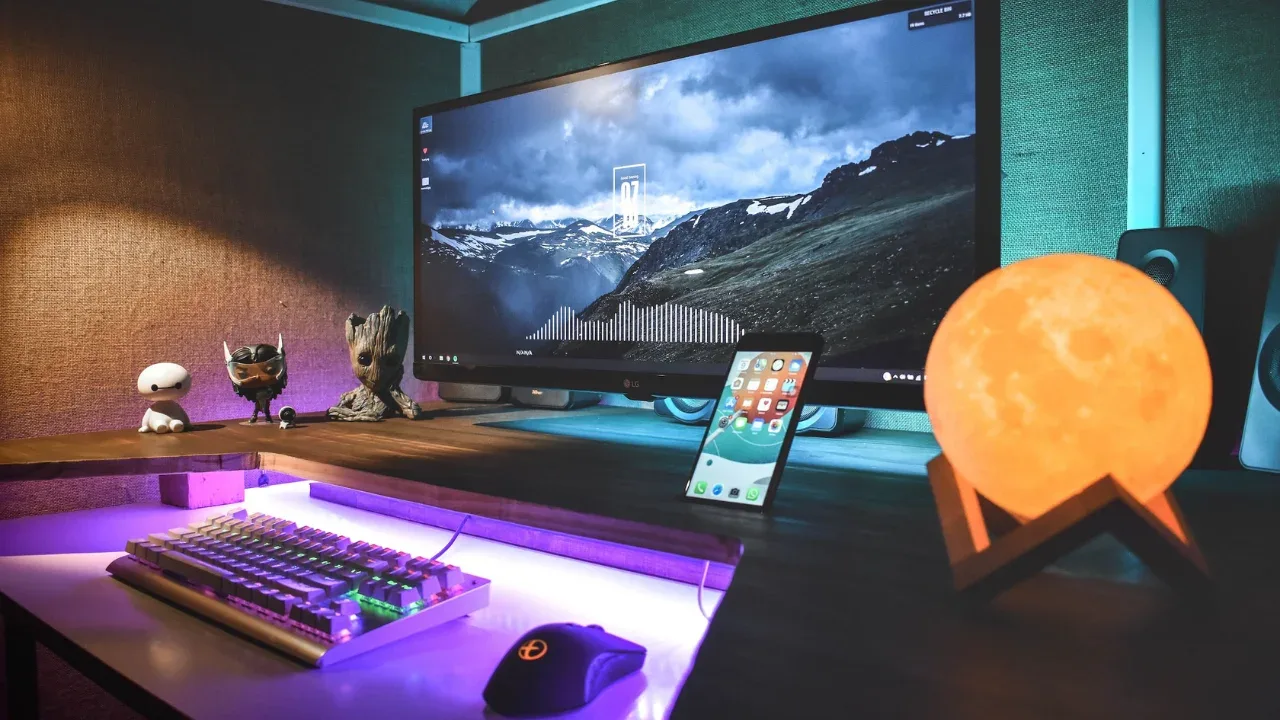
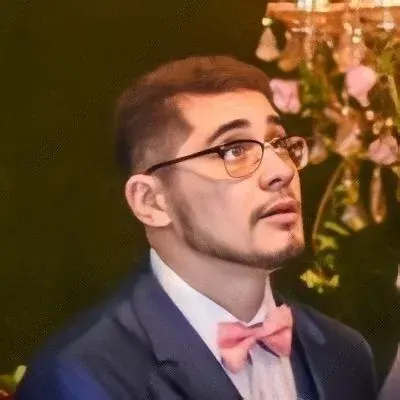
How to Import a Module Dynamically in Python ๐๐ป
Have you ever encountered a situation where you needed to import a Python module dynamically, given its full path? Maybe you're working on a project that requires loading modules from different locations on the file system, and you want to be able to do it flexibly. Well, you're in luck! In this blog post, we're going to explore the different approaches and easy solutions to dynamically import modules in Python. ๐
The Challenge: Importing a Module with a Full Path ๐
Let's start by addressing the main question: how do we load a Python module using its full path? The key here is to understand that the standard import
statement in Python works with module names, not file paths. So, importing a module using its full path requires a different approach. ๐ก
The Easy Solutions ๐กโ
Solution 1: Using importlib.util.spec_from_file_location
๐
Python's importlib
module provides a handy function called spec_from_file_location
. This function allows you to create a module specification from a file location. Here's an example:
import importlib.util
# Provide the full file path of the module
module_path = "/path/to/module.py"
# Create a module specification from the file location
spec = importlib.util.spec_from_file_location("module", module_path)
# Create the module from the specification
module = importlib.util.module_from_spec(spec)
# Load the module
spec.loader.exec_module(module)
By using spec_from_file_location
, we can create a module specification and then load the module using module_from_spec
and exec_module
. This solution provides a straightforward way to dynamically import a module using its full path. ๐
Solution 2: Using importlib.machinery.SourceFileLoader
๐
Another approach to dynamically import a module with a full path is by using importlib.machinery.SourceFileLoader
. This method directly loads the source file and creates the module. Here's an example:
import importlib.machinery
# Provide the full file path of the module
module_path = "/path/to/module.py"
# Use SourceFileLoader to load the module
loader = importlib.machinery.SourceFileLoader("module", module_path)
module = loader.load_module()
By utilizing SourceFileLoader
, we can directly load the source file and create the module without needing to create a module specification separately. This solution offers a concise method to achieve dynamic module imports. ๐
Conclusion and Call-to-Action ๐ฏ
Importing a module dynamically with a full path can be a challenging task, but with the right approach, it becomes much simpler. In this blog post, we explored two easy solutions using the importlib
module. You can choose between spec_from_file_location
or SourceFileLoader
depending on your specific needs.
Now that you've learned how to dynamically import modules in Python, it's time to put your knowledge into action! ๐ Try using these solutions in your own projects and let us know how it goes. If you have any questions or other interesting techniques to share, feel free to leave a comment below. Happy coding! ๐ป๐
<hr />
See also: How to Import a Module Given Its Name as a String? for more information on importing modules dynamically. ๐๐