How can I get a value from a cell of a dataframe?
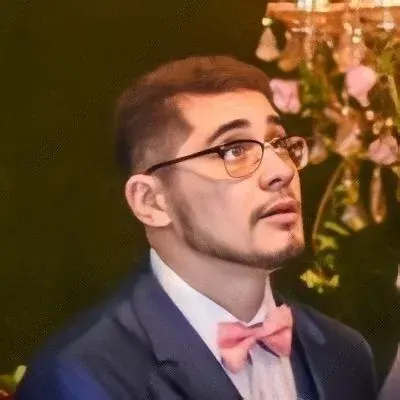
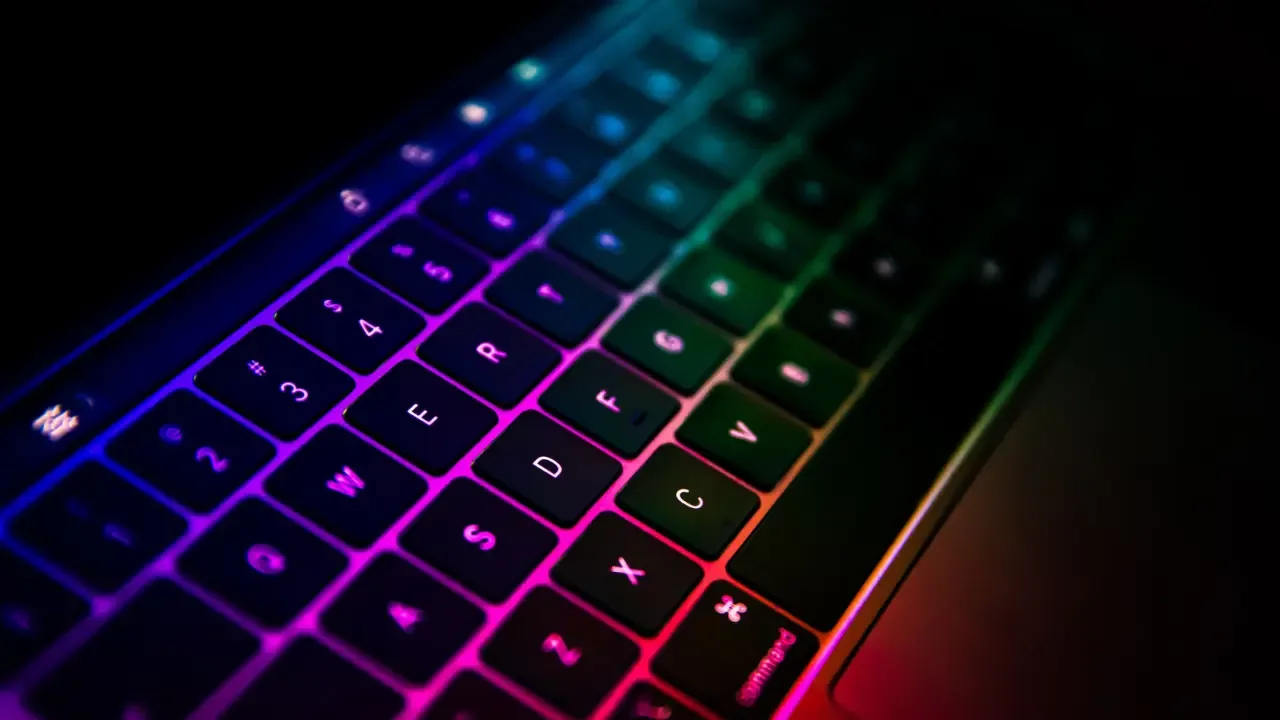
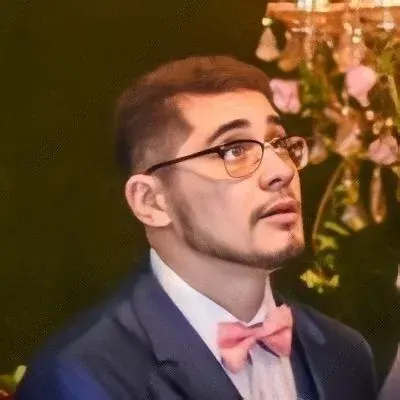
š Title: Easy Ways to Get a Value from a Cell in a Pandas DataFrame
š Introduction: Hey there! š Do you ever find yourself in a situation where you need to get a single value from a cell in a Pandas DataFrame, but you're struggling with the syntax? Don't worry, you're not alone! In this blog post, we'll address this common issue and provide you with easy solutions to help you retrieve that elusive value. Let's dive in! šŖ
š¤·āāļø The Problem: Imagine you have a DataFrame and you've constructed a condition to extract exactly one row that satisfies your criteria. Now, you want to grab a value from a specific column, but when you do it, you end up with a DataFrame containing one row and one column - not exactly what you expected, right? What you really need is just that one value, a float number.
š” The Solution: Luckily, Pandas offers a variety of ways to retrieve the single value you're looking for. Let's explore a few easy methods:
1ļøā£ Using .at[]
:
The .at[]
method allows you to access a single value in a DataFrame by label. In your case, you can try something like this:
val = d2.at[d2.index[0], 'col_name']
Here, we use .index[0]
to get the label of the first (and only) row in d2
. Replace 'col_name'
with the actual column name you want to extract the value from.
2ļøā£ Using .loc[]
with .iloc[]
:
If you prefer using integer-based indexing, you can combine .loc[]
and .iloc[]
to achieve the same result:
val = d2.loc[d2.index[0]].iloc[0]
This code snippet captures the first row using .loc[]
and then retrieves the first (and only) value using .iloc[]
.
3ļøā£ Using .squeeze()
:
If you're confident that your extraction will always result in a single cell, you can simplify the process using .squeeze()
:
val = d2.squeeze()
This method collapses the DataFrame into a Series and extracts the value directly.
š Call-to-Action: Congratulations, you now have easy ways to extract single values from a DataFrame! š If you found this post helpful and want to learn more about working with Pandas, be sure to follow our blog for regular updates. And if you have any questions or other data-related challenges, feel free to reach out in the comments below. Happy coding! š»š
⨠Conclusion:
In this blog post, we tackled the common problem of extracting a single value from a cell in a Pandas DataFrame. We provided easy-to-implement solutions using .at[]
, .loc[]
, .iloc[]
, and .squeeze()
. With these techniques, you'll be able to grab the exact value you need without ending up with a DataFrame when all you wanted was a single float number. Cheers to hassle-free data extraction! š„š