How can I flush the output of the print function?
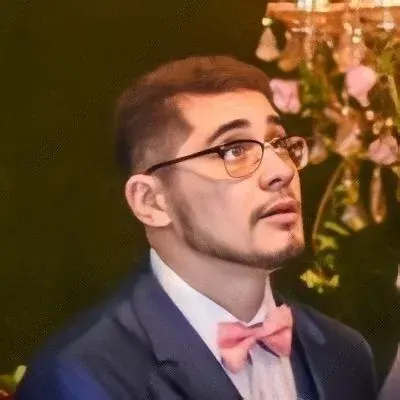
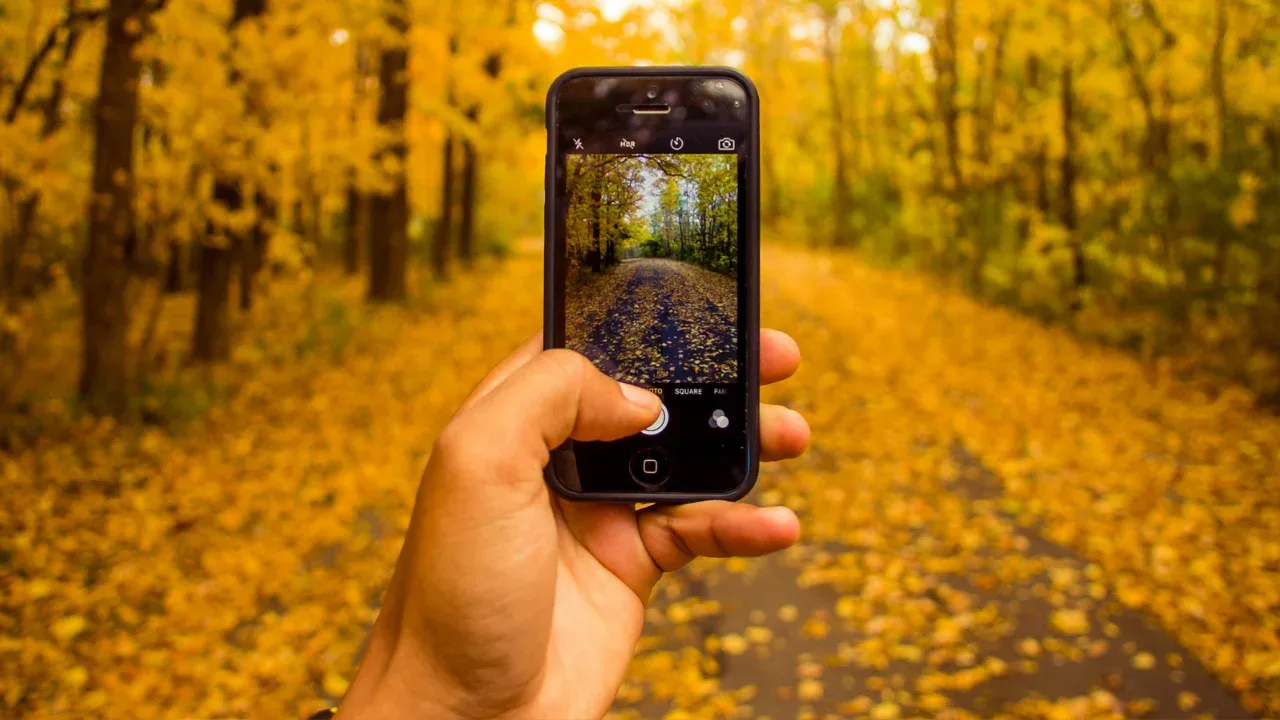
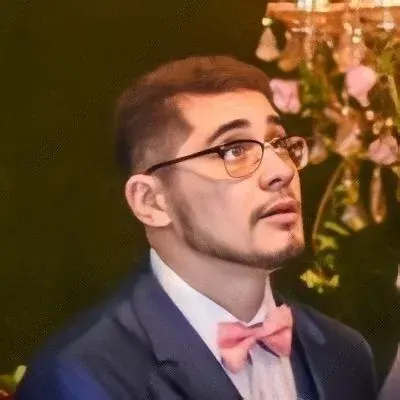
Flush it, baby! How to make Python's print
function show output immediately 🚀
So, you're writing some fancy Python code, using the print
function to display your results. But there's just one little problem: the output doesn't show up immediately on the screen. It's like your code is buffering the output, saving it up for a big reveal later. But what if you want that output to show up on the screen RIGHT NOW? Well, my friend, you've come to the right place. In this guide, we'll show you how to flush the output of the print
function and make it appear immediately.
The buffering dilemma 🧐
To understand the problem, let's first talk about buffering. Buffering is a technique used to improve efficiency in I/O operations. Instead of sending small chunks of output to the screen every time you call print
, Python saves up these chunks and sends them all at once. This can be great for performance, but sometimes we just want our output to be displayed immediately.
The flush
solution 💦
To flush the output and make it appear immediately, we can use the flush
parameter of the print
function. By default, this parameter is set to False
, which means that the output is buffered. But if we set it to True
, Python will flush the output after each print
call, making it show up immediately on the screen. Woohoo! 🎉
Here's an example:
print("Hey there!", flush=True)
In this example, the flush=True
parameter tells Python to flush the output immediately. So, when you run this code, you'll see "Hey there!" displayed on the screen right away. Pretty cool, huh? 😎
Avoiding the "display lag" pitfall ⏳
But be careful, my friend. There's another pitfall you should watch out for: the "display lag" problem. This occurs when your code is printing a lot of output, and it feels like the terminal is taking forever to catch up. Even though the output is being flushed, it takes time for the terminal to display all that information.
To avoid this issue, you can use the time.sleep()
function to introduce a short delay between each print
call. This will give the terminal some time to catch up and display the output gradually.
Here's an example:
import time
for i in range(10):
print(f"Counting: {i}", flush=True)
time.sleep(0.1)
In this example, we're using time.sleep(0.1)
to introduce a 0.1-second delay between each print
call. This makes the output appear gradually, preventing the "display lag" problem.
Take control and flush that output! 🔁
Now that you know the secret to flushing the output of the print
function, it's time to take control of your code. No more waiting for the output to show up on the screen. Just set flush=True
and watch your results appear immediately. It's time to unleash the power of immediate feedback! 💪
Have you ever had a situation where you needed to flush the output of the print
function? How did you solve it? Share your experiences in the comments below and let's help each other out! 🙌