How can I enable CORS on Django REST Framework
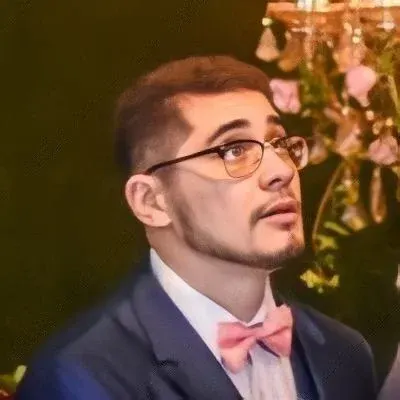
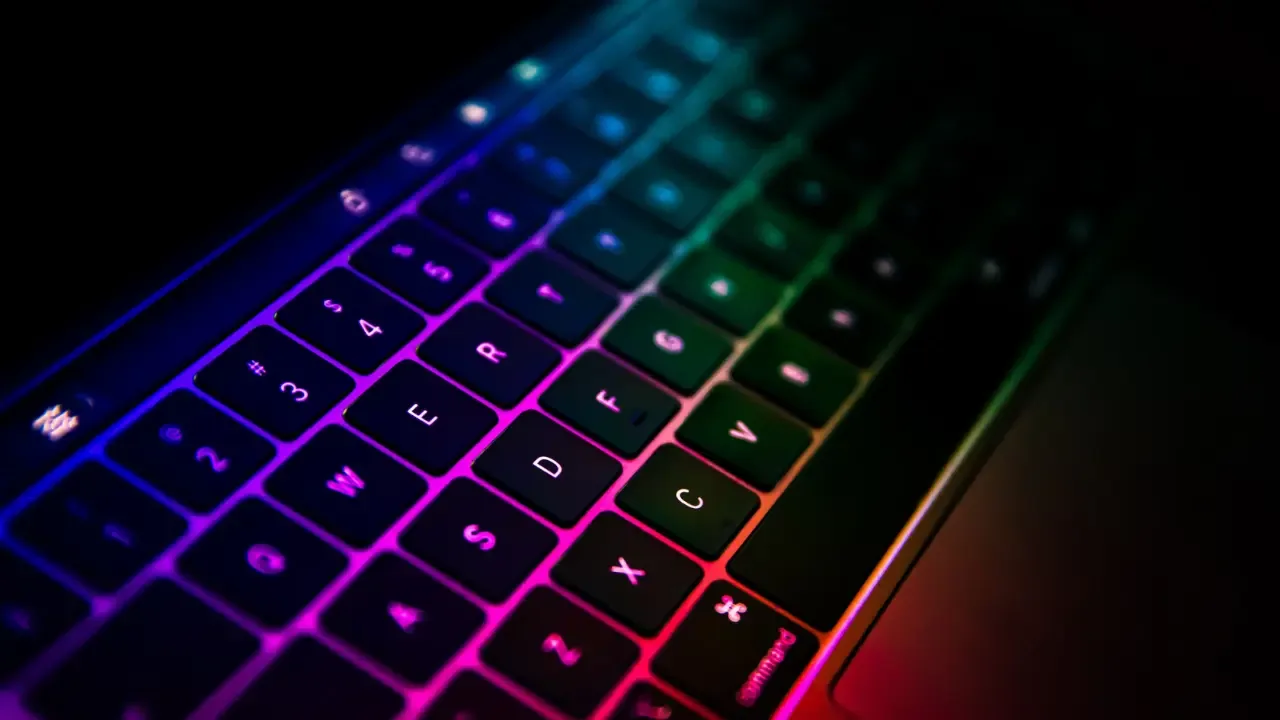
Angular is not Django's Brother.. but they can be Friends! 🤝
So, you're working on your Django project and want to take advantage of the Django REST Framework to build a powerful API. But, uh-oh! You're running into that pesky pesky CORS (Cross-Origin Resource Sharing) issue. Don't worry, we've got your back! In this blog post, we'll guide you through enabling CORS on your Django REST Framework so you can have a peaceful, harmonious integration with your frontend technology of choice - like Angular, React, or Vue.js. 🚀
What is CORS and why does it matter?
CORS, my friend, is a security feature built into web browsers to protect users from malicious attacks. It prevents requests from being made from one domain to another unless they explicitly allow it. This is great for security, but can cause a headache when you're trying to make API requests between different domains or ports.
The Django REST Framework CORS saga
Enabling CORS on Django REST Framework is not that straightforward. The official documentation gives you a hint - it tells you that you can enable CORS through middleware. But, what the reference doesn't give you is an easy step-by-step solution. That's where we come in! 😎
Step 1: Install Django CORS Headers
To begin our journey, we need to install the Django CORS Headers package. Open up your terminal and run the following command:
pip install django-cors-headers
Step 2: Add CORS Middleware to Django Settings
Next, we need to add the CORS middleware to our Django settings. Open up your project's settings.py
file and look for the MIDDLEWARE
list. Add the following line at the top, right under the SecurityMiddleware
:
'MyDjangoApp.middleware.CorsMiddleware',
Step 3: Configure CORS
Now that we have the middleware installed and added, it's time to configure it. In your settings.py
file, add the following configuration:
CORS_ALLOWED_ORIGINS = [
"http://localhost:3000",
"https://myfrontendapp.com",
]
Replace the URLs above with the domain and port of your frontend application. This configuration allows requests from the listed origins to access your API.
Step 4: Celebrate! 🎉
That's it! You've successfully enabled CORS on your Django REST Framework. Now, your API should happily accept requests from your frontend application. 🎉
Common Issues and Troubleshooting
Issue: CORS headers not being added to the response
If you're still running into CORS issues, double-check that you've added the CorsMiddleware
to your MIDDLEWARE
list correctly. Also, ensure that you've restarted your Django server after making the changes.
Issue: CORS errors with cookies and authentication
By default, Django CSRF (Cross-Site Request Forgery) protection requires cookies and won't work with cross-origin AJAX requests. If you need to make authenticated API requests, you will need to configure both CSRF and session settings correctly. You can refer to the Django documentation for more information on how to tackle this issue.
Your Turn, Fellow Django Dev! 💡
By now, you should have CORS enabled on your Django REST Framework and be able to make those sweet API requests from your frontend application. Now it's your turn to shine! Try making a request to your Django API from your beloved Angular, React, or Vue.js application. Feel the power of seamless integration between backend and frontend! 🌟
If you encounter any issues, don't hesitate to leave a comment below. We'd love to hear your thoughts, ideas, or even a cool anecdote about your CORS journey.
Keep calm and code on! Happy coding! 🚀🔥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
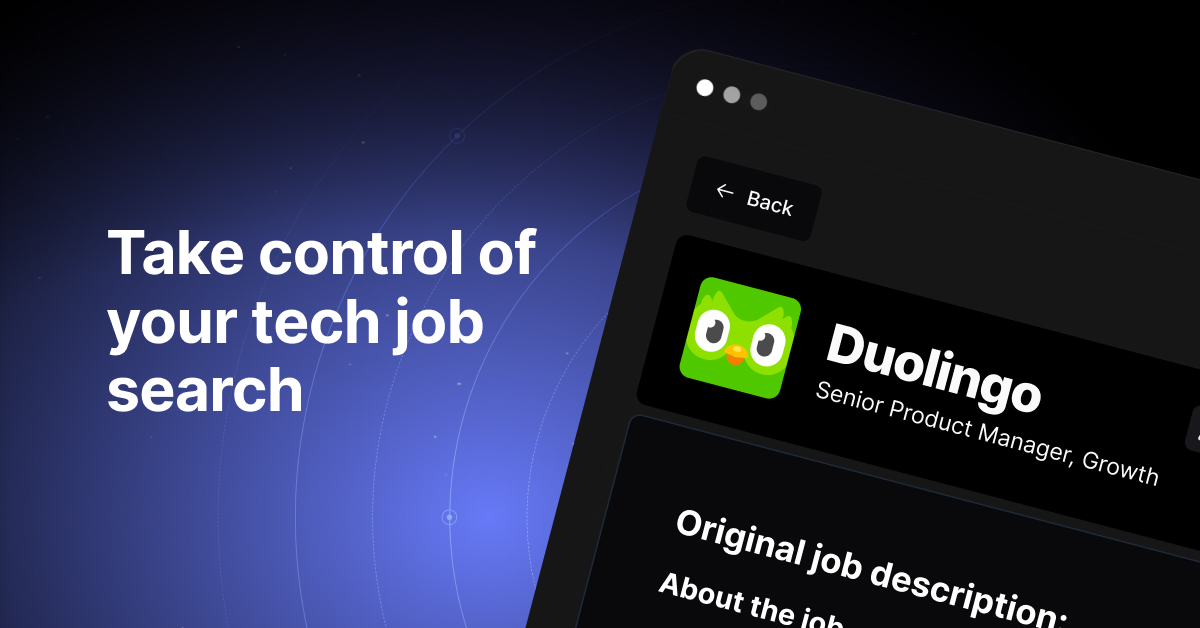