How can I disable logging while running unit tests in Python Django?
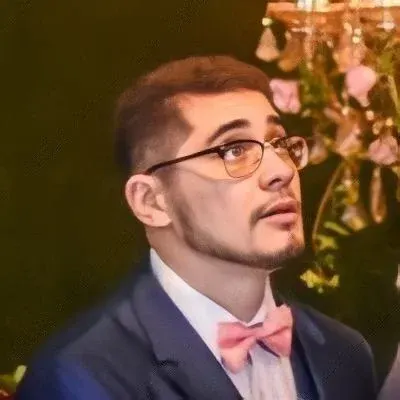

🐍 How to Disable Logging in Python Django Unit Tests? 🛠️
If you're a Python Django developer and running unit tests, you might have encountered the issue of logging cluttering your test result output. But worry not! In this guide, we'll show you easy solutions to disable logging during unit tests in Django. Let's dive in! 🏊♂️
The Problem 🧩
When running unit tests in Django, the application-specific loggers can write unnecessary information to the console. This not only clutters the test output but also affects the readability of the results. The challenge is to find a simple way to turn off logging globally specifically during unit tests.
The Solution 🔧
To disable logging while running unit tests in Python Django, we have a few simple solutions. You can choose the one that suits your needs best:
Solution 1: Using a Custom Test Runner 🏃♂️
One way to disable logging during unit tests is by creating a custom test runner. To do this, follow these steps:
Create a new file, let's call it
my_test_runner.py
, in your Django project directory.In
my_test_runner.py
, import the necessary modules:import unittest import logging
Create a custom test runner class that inherits from
unittest.TextTestRunner
:class NoLoggingTestRunner(unittest.TextTestRunner): def run(self, test): logging.disable(logging.CRITICAL) result = super().run(test) logging.disable(logging.NOTSET) return result
Set the
TEST_RUNNER
in your Django settings.py file to point to your custom test runner:TEST_RUNNER = 'my_project.my_test_runner.NoLoggingTestRunner'
By using a custom test runner, you can easily enable or disable logging as per your requirement.
Solution 2: Using the override_settings
Decorator 🎭
Another way to disable logging during unit tests is by utilizing the override_settings
decorator provided by Django. Here's how you can do it:
Import the necessary module:
from django.test import override_settings
Decorate your test case or test method with
override_settings
:@override_settings(LOGGING_CONFIG=None) def test_my_function_without_logging(self): # Your test code here pass
This sets the
LOGGING_CONFIG
setting toNone
, effectively disabling logging for that specific test case or method.
Conclusion and Call-to-Action 🎉
You've now learned two easy ways to disable logging in Python Django unit tests. No more cluttered test result output! 🥳
Choose the solution that works best for your project and start enjoying clean and readable unit test results. Share this guide with your fellow developers who might be struggling with the same logging issue in Django.
Have you faced any other challenging issues while writing unit tests in Django? Let us know in the comments below! Let's help each other and build better Django applications together! 👊
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
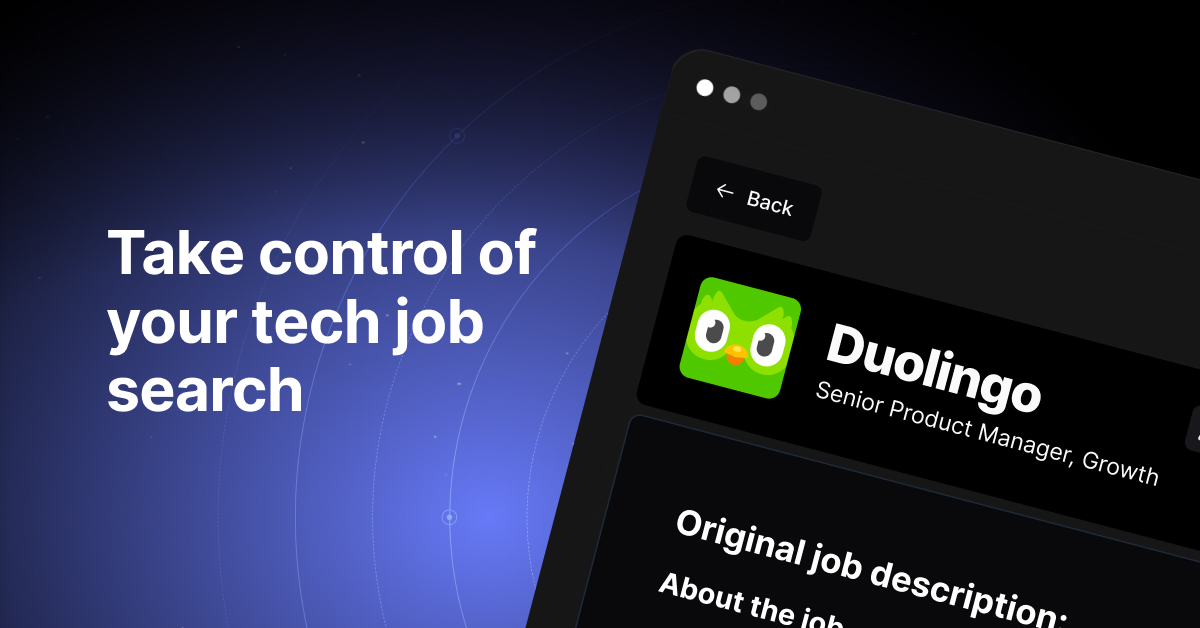