How can I convert JSON to CSV?
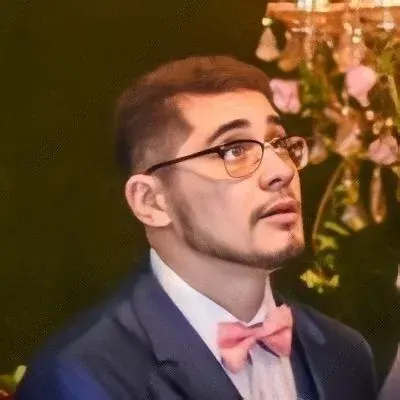
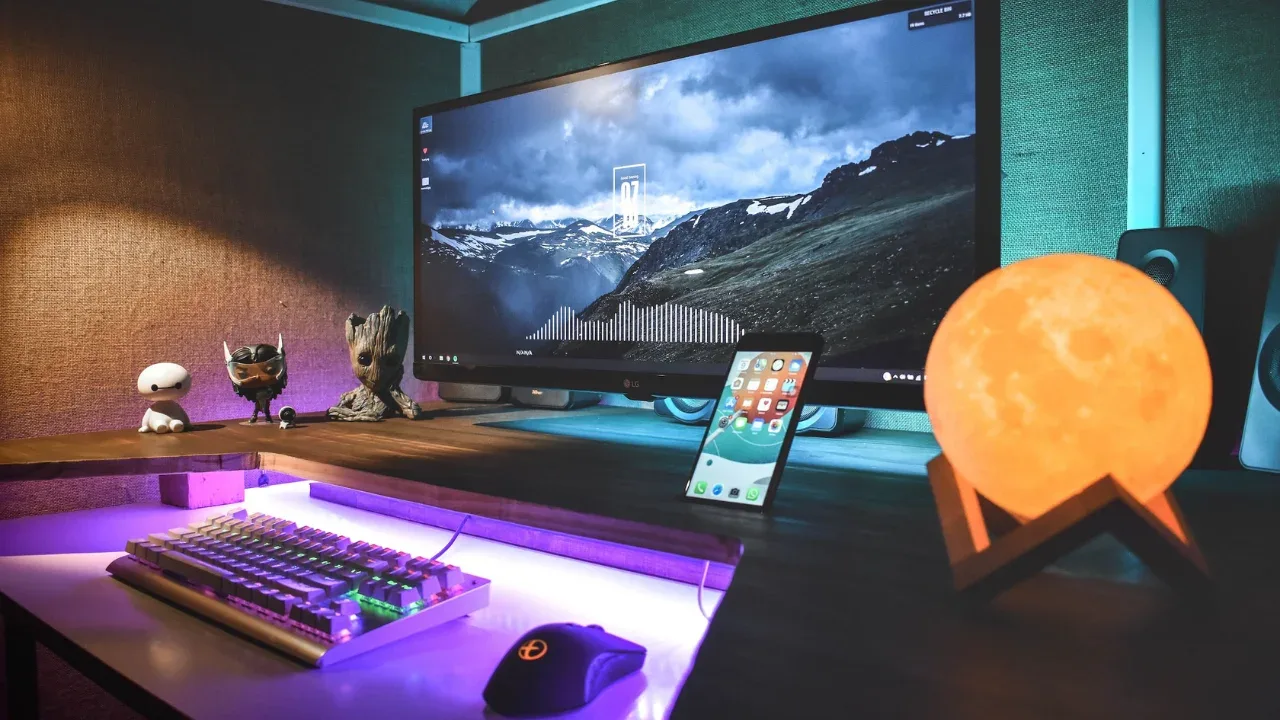
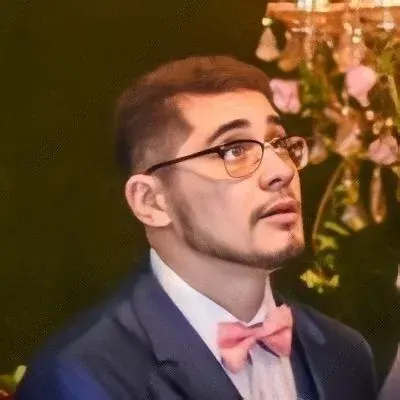
How to Convert JSON to CSV: A Step-by-Step Guide 📈
Are you facing the challenge of converting a JSON file to a CSV file? Don't worry, we've got you covered with an easy solution in Python! 🐍 In this guide, we'll walk you through the process and address common issues you may encounter along the way. Let's dive in! 💪
The Problem 🔍
One of our readers recently asked us, "How can I convert a JSON file to a CSV file using Python?" They shared their initial code attempt, but unfortunately, it didn't work as expected. The Django error they received was 'file' object has no attribute 'writerow'
.
The Solution 💡
To convert JSON to CSV, we need to use the json
and csv
libraries in Python. Here's an updated code snippet that resolves the initial issue and successfully converts the JSON file to CSV:
import json
import csv
with open('data.json') as f:
data = json.load(f)
with open('data.csv', 'w', newline='') as f:
csv_file = csv.writer(f)
csv_file.writerow(data[0].keys()) # Write header row
for item in data:
csv_file.writerow(item.values())
Explanation of the Solution 📝
Let's take a closer look at the updated code and understand what each step does:
We import the
json
andcsv
libraries to utilize their functionality.We use the
with
statement to ensure proper handling of file resources. This way, we don't have to manually close the file afterward.We open the JSON file using
open('data.json')
and load its contents usingjson.load(f)
.We then open the CSV file using
open('data.csv', 'w', newline='')
. The'w'
parameter signifies that we want to open the file in write mode, and thenewline=''
parameter ensures that line breaks are handled correctly across different operating systems.We create a csv
writer
object usingcsv.writer(f)
to write the data into the CSV file.We write the header row to the CSV file using
csv_file.writerow(data[0].keys())
. This line extracts the keys (column names) from the first item in the JSON data and writes them as the first row in the CSV.Finally, we iterate through each item in the JSON data and write its values as rows in the CSV using
csv_file.writerow(item.values())
.
And that's it! 🎉 You should now have a CSV file with the converted JSON data.
Handling Common Issues ⚙️
In case you encounter any issues during the conversion process, here are a couple of common problems and their solutions:
Error: sequence expected
If you see this error, it means that the input JSON file is not a valid iterable. To resolve this issue, make sure that your JSON data is structured as an array of objects (like in the sample JSON provided), rather than a single object.
Missing Header Row
If your resulting CSV file is missing the header row (column names), it's possible that your JSON data doesn't include a consistent set of keys across all objects. You could manually define the keys in this case or update your JSON data to ensure consistency.
Share Your Success! 💬
We hope that this guide helped you successfully convert your JSON file to a CSV file using Python! If you encountered any issues or have more questions, feel free to leave a comment below. We'd be happy to assist you further. Happy coding! 😄💻