How can I build multiple submit buttons django form?
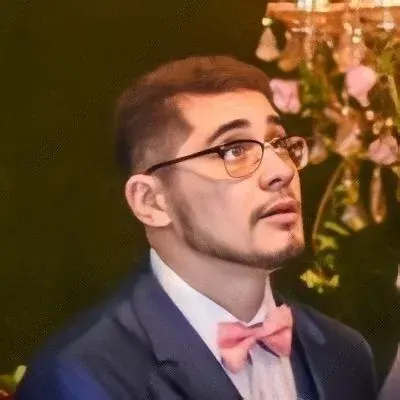
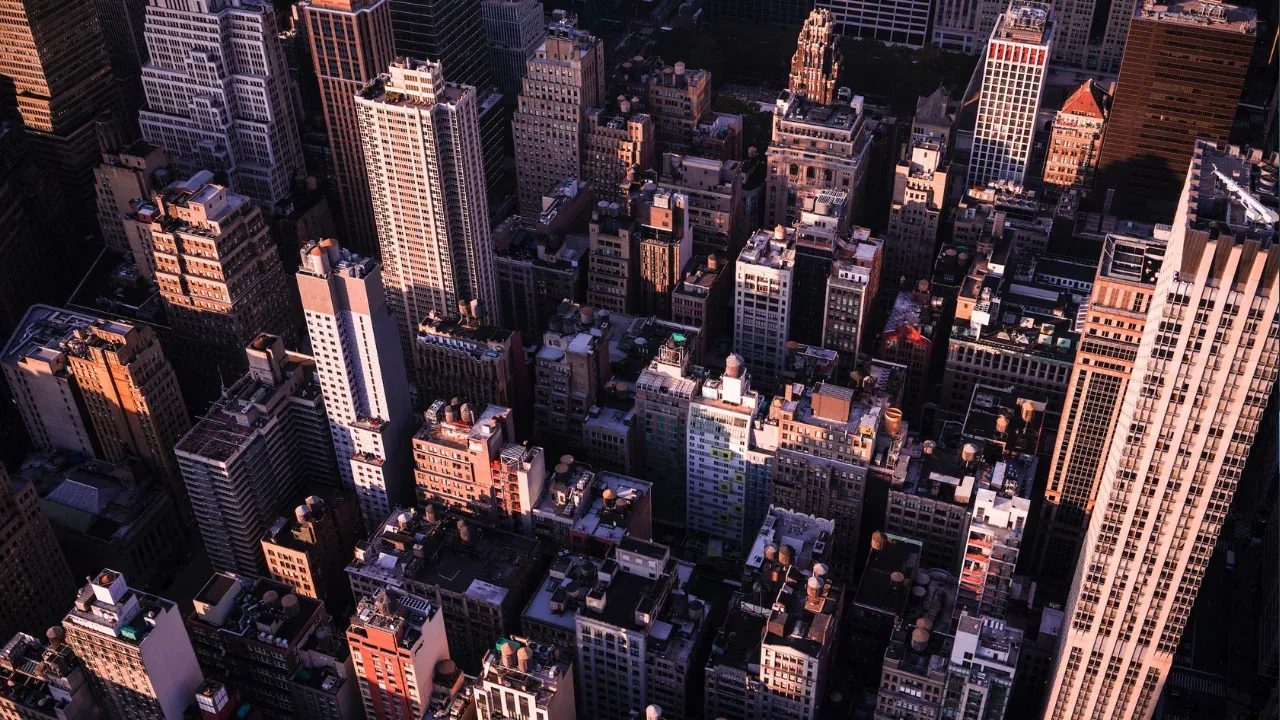
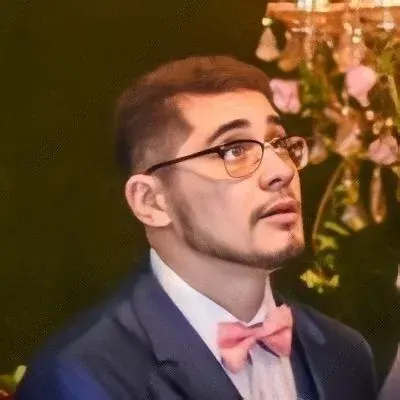
š Title: How to Build Multiple Submit Buttons in Django Forms
š Introduction Have you ever wondered how to add multiple submit buttons to your Django form? š¤ Well, you're in luck! In this blog post, we'll address a common issue faced by many developers and provide easy solutions that will save you time and frustration. So let's dive right in and discover how to build multiple submit buttons in Django forms! š
āļø The Problem Imagine having a form with an email input and two submit buttons - one for subscribing to a newsletter and another for unsubscribing. You might have something like this in your code:
<form action="" method="post">
{{ form_newsletter }}
<input type="submit" name="newsletter_sub" value="Subscribe" />
<input type="submit" name="newsletter_unsub" value="Unsubscribe" />
</form>
Your Django form class, let's call it NewsletterForm
, may look like this:
class NewsletterForm(forms.ModelForm):
class Meta:
model = Newsletter
fields = ('email',)
So the question arises, how can we determine which button was clicked when the form is submitted? š¤
š ļø The Solution
To solve this problem, we can override the clean
method of our form and access the form's data. Here's an example of how to do it:
class NewsletterForm(forms.ModelForm):
# ...
def clean(self):
cleaned_data = super().clean()
if 'newsletter_sub' in self.data:
# Subscribe button was clicked
# Perform additional validation or actions if needed
elif 'newsletter_unsub' in self.data:
# Unsubscribe button was clicked
# Perform additional validation or actions if needed
return cleaned_data
By checking the presence of the submit button names in the self.data
dictionary, we can determine which button was clicked and perform any necessary actions accordingly. šµļøāāļø
š Conclusion
Building multiple submit buttons in Django forms doesn't have to be a headache anymore! š With the simple solution provided in this blog post, you can easily determine which button was clicked when the form is submitted. Remember to override the clean
method of your form class and check the presence of the submit button names in the self.data
dictionary.
So go ahead, implement this solution in your Django projects and simplify your development process! šŖ If you have any questions or suggestions, feel free to comment below. Happy coding! š