How can I access environment variables in Python?
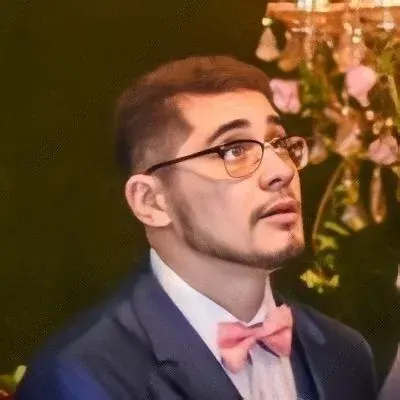
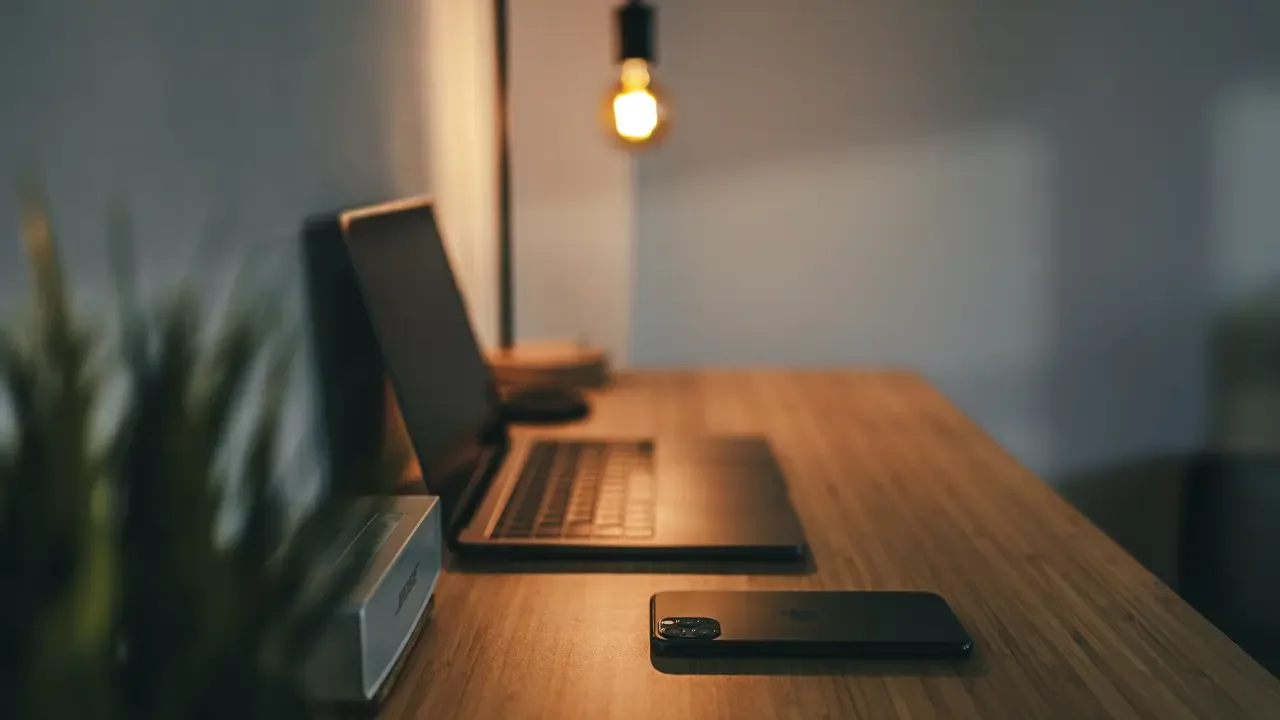
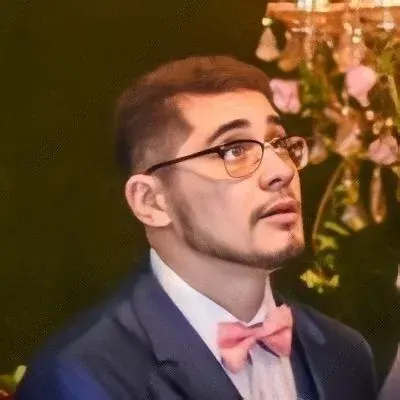
๐ How to Access Environment Variables in Python?
So you want to access environment variables in Python? ๐ค No worries, I've got you covered! ๐
Understanding Environment Variables
Before diving into how to access environment variables in Python, let's quickly understand what environment variables are. ๐ค
Environment variables are dynamic values that can affect the behavior of processes or programs running on a computer. They are usually set by the operating system or user-defined, and they provide a way to configure various aspects of a system.
For example, you might have an environment variable called DATABASE_URL
that stores the URL of your database. By using environment variables, you can keep sensitive information like database credentials separate from your code and easily change them without modifying your codebase. ๐
Accessing Environment Variables in Python
Now, let's get to the good stuff! Here are a few ways you can access environment variables in Python:
Method 1: Using the os
Module
Python's built-in os
module provides a method called environ
which returns a dictionary containing all the environment variables. To access a specific environment variable, simply provide its name as the key. ๐๏ธ
Here's an example:
import os
database_url = os.environ.get('DATABASE_URL')
print(database_url)
Method 2: Using the dotenv
Library
If you prefer a more convenient way to work with environment variables, you can use the dotenv
library. This library allows you to store environment variables in a file named .env
and easily load them into your Python script. โ๏ธ
To use dotenv
, you need to follow these steps:
Install the
dotenv
library:pip install python-dotenv
Create a
.env
file in the same directory as your Python script and add your environment variable there. For example:DATABASE_URL=your_database_url
In your Python script, import the
dotenv
module and load the environment variables:from dotenv import load_dotenv load_dotenv() database_url = os.getenv('DATABASE_URL') print(database_url)
Method 3: Using Third-Party Libraries
If you're working with specific frameworks like Flask or Django, they often have built-in features for handling environment variables. For example, Flask uses the config
object to manage environment variables, and Django uses the settings
module. Check the documentation of your chosen framework for more details. ๐
Wrap Up
Accessing environment variables in Python is crucial for securely managing sensitive information and making your programs more configurable. Whether you choose to use the os
module, dotenv
library, or framework-specific solutions, the key is to keep your code clean, modular, and easily maintainable. ๐งน
Now that you know how to access environment variables in Python, go ahead and give it a try! If you have any questions or need further guidance, leave a comment below. Happy coding! ๐ป๐