Hidden features of Python
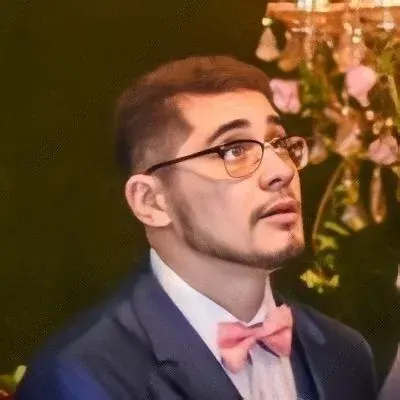
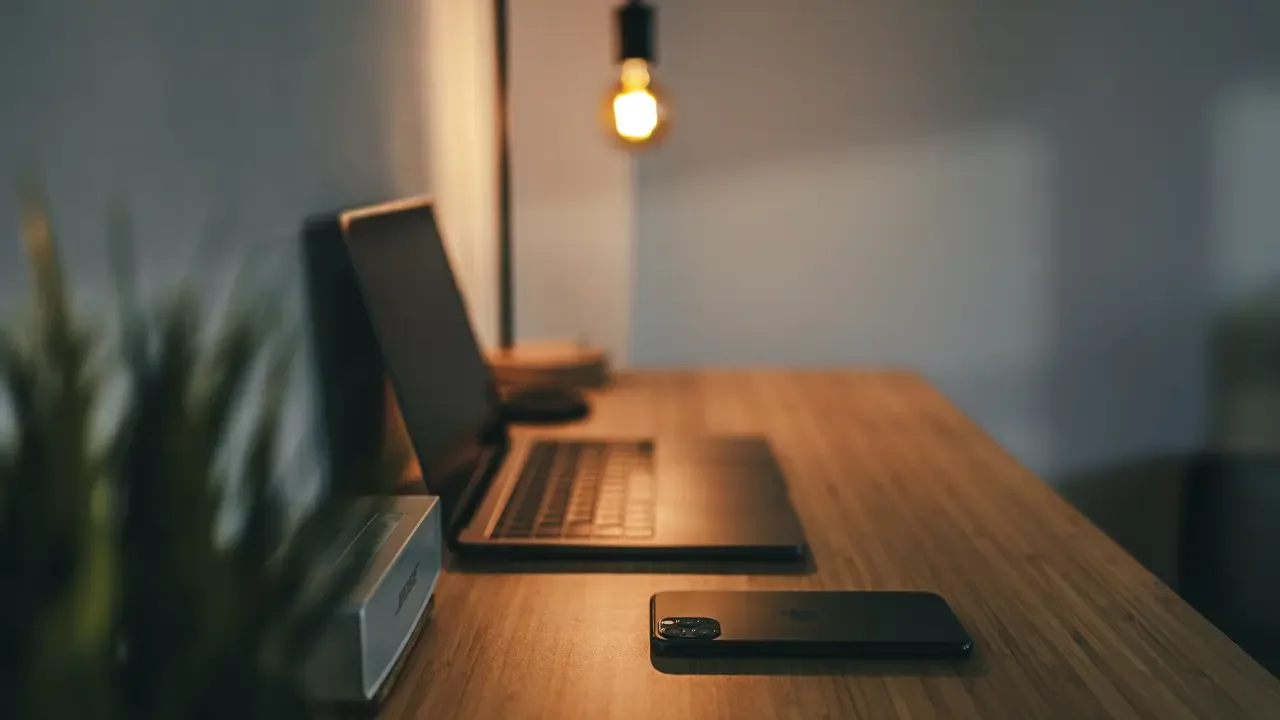
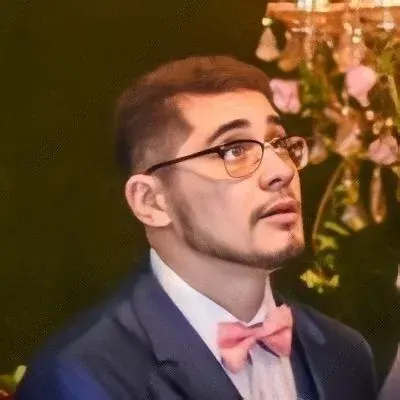
๐ Revealing the Hidden Gems of Python ๐
Python is a versatile and powerful programming language that is known for its simplicity and readability. However, even seasoned Pythonistas may not be aware of its hidden features that can enhance their coding experience. In this blog post, we will dive into some lesser-known but super useful features of Python that can level up your programming game. Let's hop right in! ๐
๐ฏ Argument Unpacking
Have you ever wanted to pass a list or tuple of values as arguments to a function without explicitly unpacking them? Python's argument unpacking feature has got your back! You can use the *
operator to unpack iterable objects, like so:
def multiply(a, b, c):
return a * b * c
values = [2, 3, 4]
result = multiply(*values) # Passes 2, 3, 4 as separate arguments
print(result) # Output: 24
By using argument unpacking, you can flexibly pass multiple values to a function without the need for manual unpacking. ๐
๐งช Docstring Tests
Writing tests for your functions is essential for ensuring their correctness. But did you know that Python allows you to include tests within your docstrings? We call them "doctests." ๐
def square(number):
"""
Squares the given number.
>>> square(4)
16
>>> square(-3)
9
"""
return number ** 2
With docstring tests, you can write concise and executable documentation that serves as both code examples and test cases. To run the doctests, simply use the -m doctest
command-line option or a testing framework like pytest
. ๐งช
๐ Default Argument Gotchas ๐ซ
Python allows function parameters to have default values, which are assigned when the value is not explicitly provided during function calls. However, when using mutable objects like lists or dictionaries as default arguments, beware of the potential gotchas:
def append_to_list(element, my_list=[]):
my_list.append(element)
return my_list
result1 = append_to_list(1)
print(result1) # Output: [1]
result2 = append_to_list(2)
print(result2) # Output: [1, 2]
In the example above, a new list is not created if the default argument my_list
is already present. As a result, each function call modifies the same list. To avoid this issue, it's recommended to use None
as the default value and create a new list inside the function:
def append_to_list(element, my_list=None):
if my_list is None:
my_list = []
my_list.append(element)
return my_list
๐ For/Else
Have you ever wondered about an else
statement after a for
loop in Python? Surprisingly, it's not an error but a hidden gem! The else
block is executed only if the loop completes naturally, without encountering a break
statement:
fruits = ['apple', 'banana', 'grape', 'melon']
for fruit in fruits:
if 'n' not in fruit:
print(fruit)
else:
print("All fruits checked!")
# Output: apple, grape, melon, All fruits checked!
The else
block can be useful in scenarios where you want to perform certain actions when the loop has successfully completed its iterations. ๐
...
To discover even more hidden features of Python, check out the full list here. Feel free to explore and experiment with these features to level up your Python coding skills! ๐ช
Now it's your turn! Let us know in the comments below which hidden feature of Python surprised or delighted you the most. Share your experiences and any cool examples you have come across. Happy coding! ๐ฉโ๐ป๐จโ๐ป
๐ฃ Call to Action:
If you found this blog post helpful, don't forget to share it with your fellow Python enthusiasts. Sharing is caring! ๐๐
And hey, while you're at it, why not follow our Tech Blog for more engaging content on Python and other exciting technologies? Join our coding community and stay updated with the latest trends and tips in the world of tech. ๐๐
See you in the next post! Happy coding! ๐๐