Having Django serve downloadable files
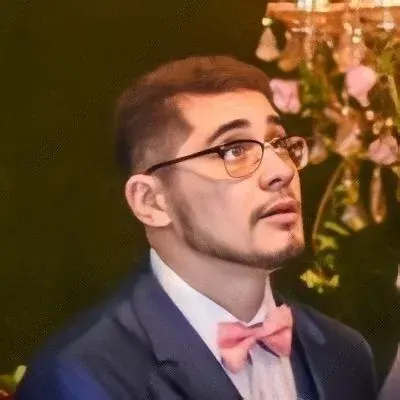
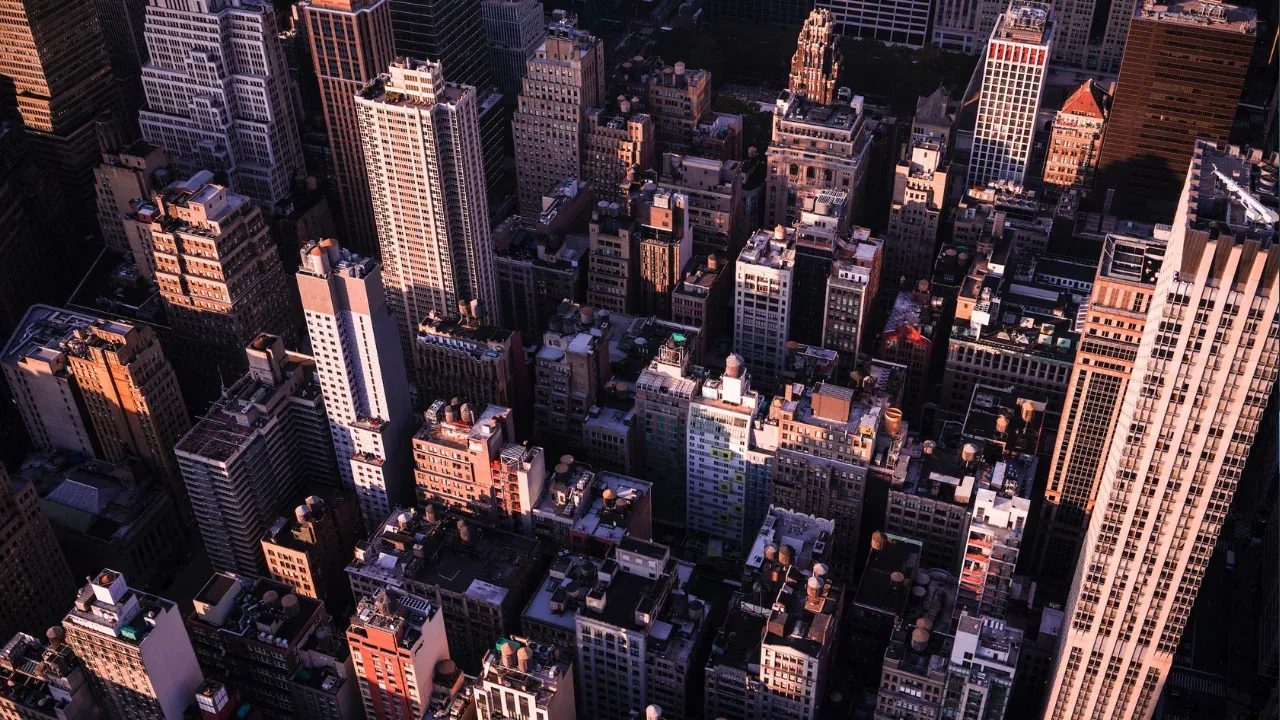
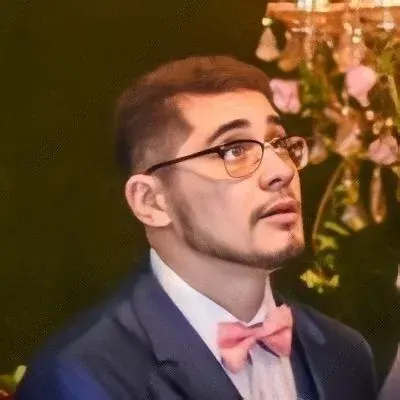
Django 101: Serving Downloadable Files with Ease! 😎
So, you want to serve downloadable files in Django, but you don't want users to directly access the actual file path? No worries! In this blog post, we'll walk you through the common challenges and provide you with easy solutions to serve those files securely. 🚀
The Challenge: Securing Downloadable File Paths 🔒
We understand the importance of keeping your files safe and hidden from prying eyes. To achieve this, we need to ensure that the file's URL doesn't directly expose its actual path. Let's dive into the solutions!
Solution 1: Using Django's Media Files 😃
Django offers a fantastic feature called media files, which allows you to handle user-uploaded files like images, documents, and even videos. To serve downloadable files securely, follow these steps:
Declare a
MEDIA_ROOT
in your Django settings file:MEDIA_ROOT = '/home/user/files/'
Configure your
MEDIA_URL
:MEDIA_URL = '/media/'
In your Django project's main
urls.py
file, add the following line to serve media files:from django.conf import settings from django.conf.urls.static import static urlpatterns += static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
Now, you can use the following URL:
http://example.com/media/somefile.txt
to serve thesomefile.txt
file securely.
With this solution, Django handles the file's path, keeping it hidden from users while still allowing them to download it. 😎
Solution 2: Custom File Download View 📥
Sometimes, you may need more control over how files are served. If you want to obscure the file path further or add additional logic before serving the file, a custom file download view is what you need! Here's how to implement it:
Define a new view in one of your Django app's
views.py
files:from django.http import HttpResponseRedirect, FileResponse import os def download_view(request): filename = request.GET.get('f') file_path = os.path.join('/home/user/files/', filename) response = FileResponse(open(file_path, 'rb')) response['Content-Disposition'] = f'attachment; filename="{filename}"' return response
Add the corresponding URL pattern to your
urls.py
:from django.urls import path from .views import download_view urlpatterns = [ # Your other URL patterns go here path('download/', download_view, name='download'), ]
You can now use the following URL:
http://example.com/download/?f=somefile.txt
to trigger the download of thesomefile.txt
file.
With a custom download view, you have full control over the file's path and can add additional checks or operations before serving it to the user. 🙌
Time to Level Up Your Downloading Game! ⚡️
Congratulations on conquering the art of serving downloadable files in Django! By utilizing Django's media files or implementing a custom download view, you've made your site more secure and user-friendly. Now it's your turn to implement these solutions and level up your downloading game!
If you have any questions or other challenging scenarios, feel free to leave a comment below! Let's share our knowledge and help each other out. 💪💬
Remember, sharing is caring! If you found this blog post helpful, don't hesitate to share it with your fellow Django enthusiasts. Happy coding! 🙌✨