Getting value of enum on string conversion
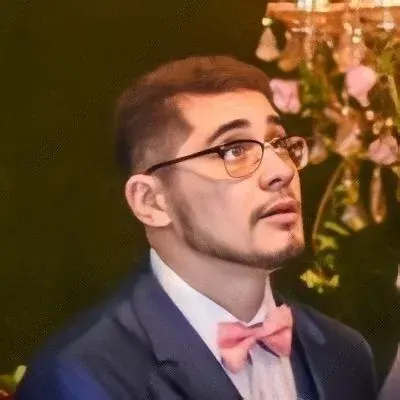
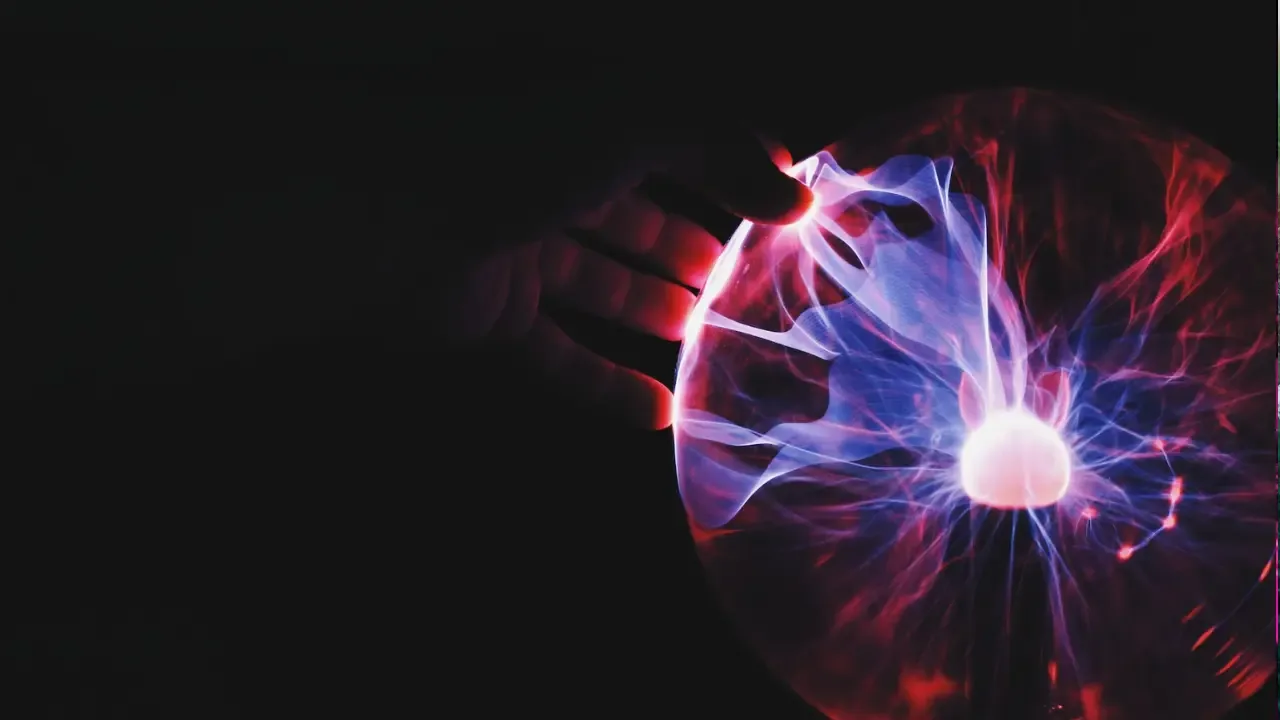
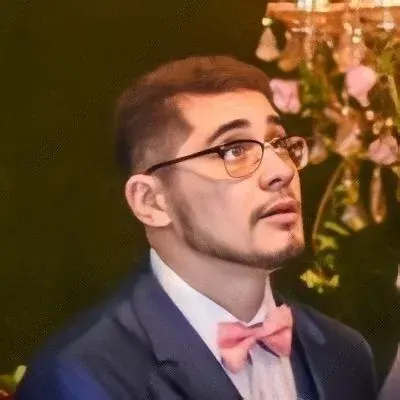
🤖 Getting the Value of an Enum on String Conversion
Enums provide a convenient way to define a set of named values, making your code more readable and semantically correct. However, when it comes to retrieving the value of an enum during string conversion, things can get a bit tricky. But fear not! In this blog post, we'll explore a common issue and provide easy solutions to help you achieve the desired functionality.
The Problem
Let's start by understanding the problem at hand. Consider the following code snippet:
from enum import Enum
class D(Enum):
x = 1
y = 2
print(D.x)
Running this code will output D.x
, rather than the expected value of 1
. The default string representation of an enum is the name of the enum itself concatenated with the name of the value, separated by a dot.
Solution 1: Accessing the Value Directly
One way to get the value of an enum during string conversion is by accessing it directly. Instead of relying on the default string representation, you can retrieve the value using the .value
attribute. Here's how you can modify your code to achieve the desired functionality:
from enum import Enum
class D(Enum):
x = 1
y = 2
print(D.x.value)
Running the updated code will now output 1
, which is the expected value.
Solution 2: Overriding the __str__
Method
Another approach is to override the __str__
method of your enum to provide a custom string representation. By doing so, you can control exactly what gets displayed when an enum is converted to a string. Here's an example:
from enum import Enum
class D(Enum):
x = 1
y = 2
def __str__(self):
return str(self.value)
print(D.x)
With this modification, running the code will output 1
, which is what we wanted.
Solution 3: Using a Helper Function
If you find yourself needing this functionality frequently across your codebase, you can create a helper function to take care of the conversion for you. This approach allows for more flexibility and reusability. Here's an example:
from enum import Enum
class D(Enum):
x = 1
y = 2
def get_enum_value(enum):
return enum.value
print(get_enum_value(D.x))
By utilizing the get_enum_value
function, you can get the desired value of any enum without having to remember to access the .value
attribute every time.
Conclusion and Call-to-Action
Dealing with the string representation of enums can be tricky, but with the solutions provided above, you can easily obtain the value you need. Whether you choose to access the value directly, override the __str__
method, or create a helper function, the choice is yours.
Next time you encounter this issue, remember these easy solutions to save time and frustration. Don't let enums baffle you – get their values with confidence! 💪
Now, we want to hear from you! Have you ever faced difficulties while getting enum values? How did you solve them? Share your experiences in the comments below and let's learn from each other! 😊