Getting the SQL from a Django QuerySet
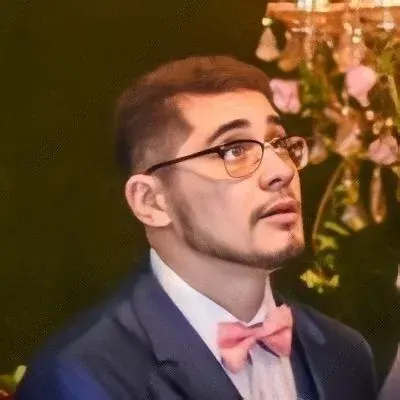
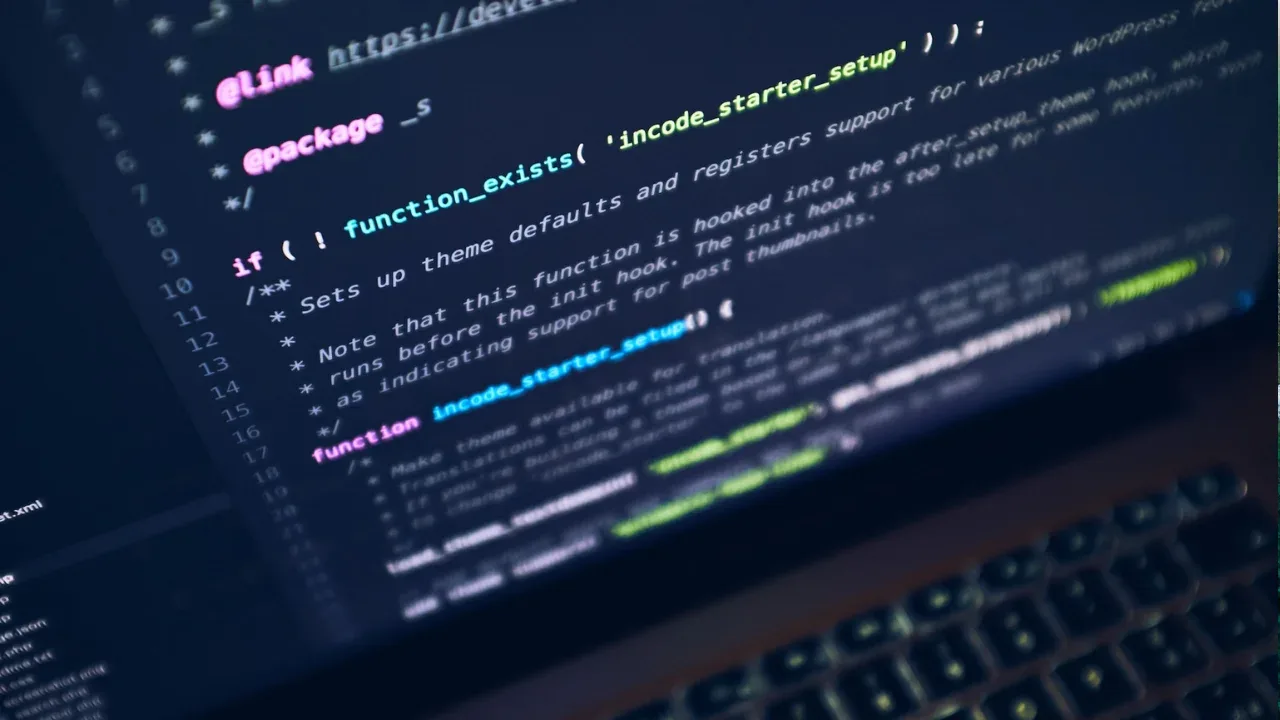
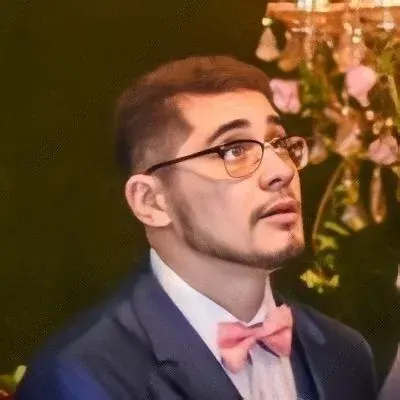
Getting the SQL from a Django QuerySet 👨💻💡
Are you trying to debug some strange behavior in your Django application but can't figure out what queries are being executed on the database? Don't worry, we've got you covered! In this blog post, we'll show you an easy way to extract the SQL statements from a QuerySet object in Django.
The Problem 🤔
Sometimes, when you encounter unexpected behavior in your Django application, it's crucial to understand the underlying SQL queries being sent to the database. It allows you to analyze and optimize the performance of your application, troubleshoot data-related issues, or simply gain insights into what's happening behind the scenes.
The Solution ✅
Django provides a powerful feature that allows you to obtain the SQL statements from a QuerySet object. You can achieve this by using the query
attribute of the QuerySet object.
Here's an example:
# Let's assume you have a QuerySet object called 'my_queryset'
sql_query = my_queryset.query.__str__()
print(sql_query)
By accessing the query
attribute and converting it to a string, you can obtain the SQL representation of the QuerySet object. This SQL statement shows the exact query Django will execute on the database.
Example 👀
Let's illustrate this with a practical example. Suppose you have a Django model named Person
, and you want to fetch all instances where the age is greater than 30:
class Person(models.Model):
name = models.CharField(max_length=100)
age = models.IntegerField()
To get the SQL query from the QuerySet object, you can do the following:
my_queryset = Person.objects.filter(age__gt=30)
sql_query = my_queryset.query.__str__()
print(sql_query)
The output will be the SQL statement corresponding to the filtering operation:
SELECT "myapp_person"."id", "myapp_person"."name", "myapp_person"."age"
FROM "myapp_person"
WHERE "myapp_person"."age" > 30
Now you have the SQL statement and can use it to dig deeper into your application's behavior or share it with your team to investigate any potential database-related issues.
Conclusion 🎉
Obtaining the SQL from a Django QuerySet object is a handy technique that can greatly assist in debugging and understanding the behavior of your application. By following the steps outlined in this blog post, you can easily retrieve the corresponding SQL queries and gain valuable insights into your application's database interaction.
We hope this guide helps you in your Django development journey! If you found this post helpful or have any additional tips or tricks to share, let us know in the comments below. Happy coding! 😄👍