Getting the class name of an instance
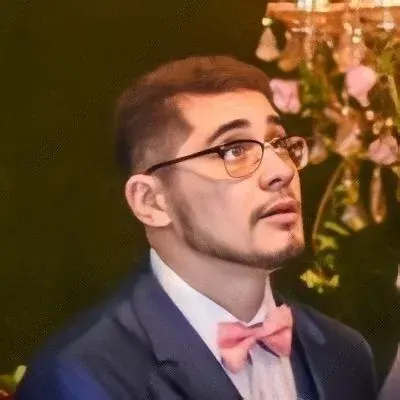
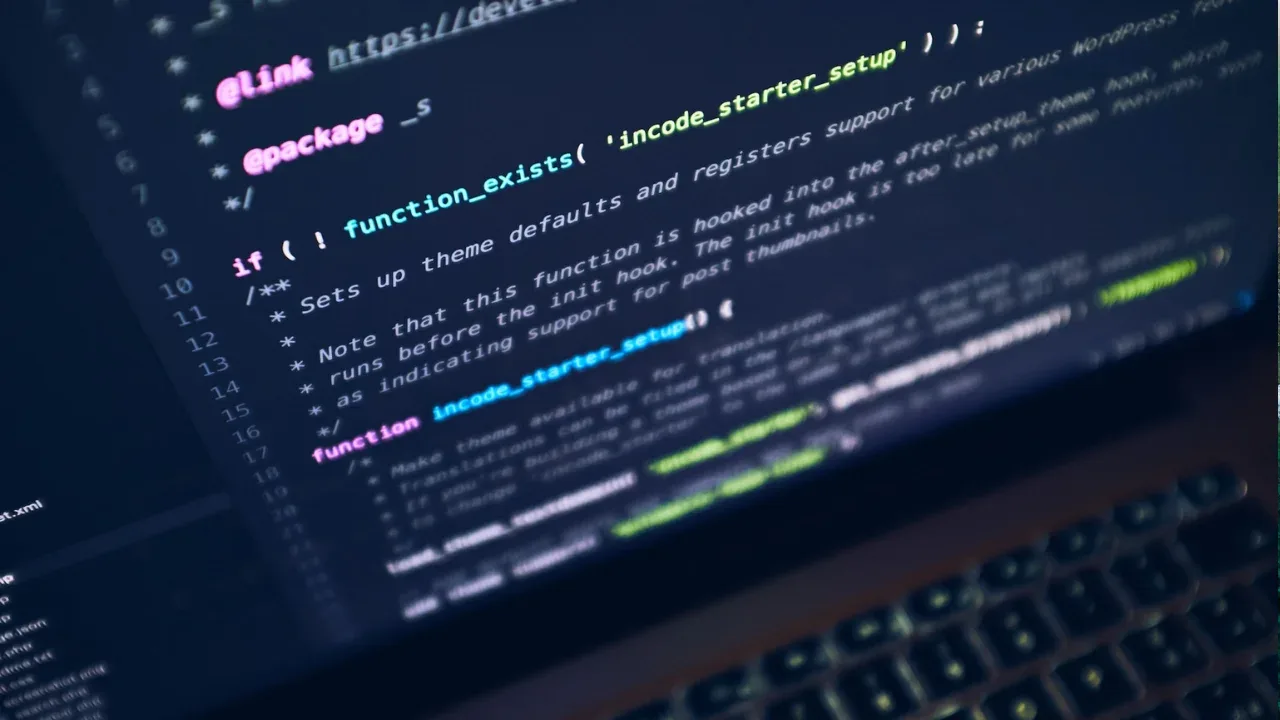
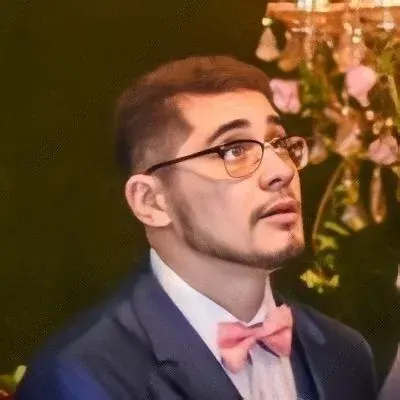
Getting the Class Name of an Instance in Python: A Simple Guide 😎
So you want to find out the name of the class used to create an instance of an object in Python? 🐍 Don't worry, we've got you covered! In this blog post, we will explore common issues and provide easy solutions to help you accomplish this task. Let's dive in! 💪
The Inspect Module or the __class__
Attribute? 🤔
Before we start, you may be wondering whether to use the inspect
module or parse the __class__
attribute. Both options are valid, so let's take a closer look at each one:
Option 1: Using the Inspect Module 🕵️♀️
The inspect
module in Python provides several functions that allow you to retrieve information about live objects, such as classes and their instances. To get the class name from an instance, you can use the inspect.getmodule()
and inspect.getmembers()
functions.
Here's an example to illustrate how to use the inspect
module:
import inspect
class MyClass:
pass
my_instance = MyClass()
class_name = inspect.getmodule(my_instance).__name__
print(class_name) # Output: __main__
In this example, we create a class called MyClass
and then instantiate an object my_instance
from that class. By using inspect.getmodule()
, we can retrieve the module from which the instance was created. Finally, we print the module name to obtain the class name.
Option 2: Parsing the __class__
Attribute 📚
In Python, every instance of a class has a special attribute called __class__
, which refers to the class used to create that instance. By accessing this attribute, you can easily obtain the class name.
Take a look at the following example:
class MyClass:
pass
my_instance = MyClass()
class_name = my_instance.__class__.__name__
print(class_name) # Output: MyClass
Here, we create the MyClass
class and instantiate my_instance
. By accessing the __class__
attribute and then the __name__
attribute, we can directly retrieve the class name.
Easy Solutions to a Common Problem ✔️
Now that we have explored both options, you might be wondering which one is the best choice. The truth is, it depends on your specific use case. Here are some things to consider when choosing between the inspect
module and parsing the __class__
attribute:
If you need additional information about the class or want to perform more complex operations on it, the
inspect
module may be the better option. It provides more flexibility and powerful functionality.On the other hand, if you only need the class name and prefer a simpler approach, using the
__class__
attribute is more straightforward and concise.
Ultimately, it's up to you to decide which solution fits your needs best. Don't hesitate to experiment and choose the approach that feels most comfortable for your codebase!
Call-To-Action: Let's Engage! 🚀
We hope this guide has helped you understand how to get the class name of an instance in Python. Now it's your turn to put it into practice!
Have you ever encountered any challenges related to this topic?
Do you prefer using the
inspect
module or parsing the__class__
attribute?Is there anything else you would like to learn or discuss about Python?
Leave a comment below and join the conversation! Let's share our knowledge and help each other become better Pythonistas! 🎉