Getting a map() to return a list in Python 3.x
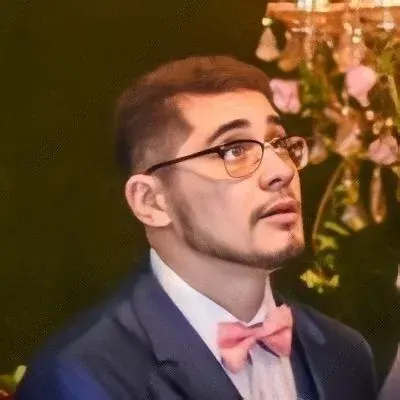
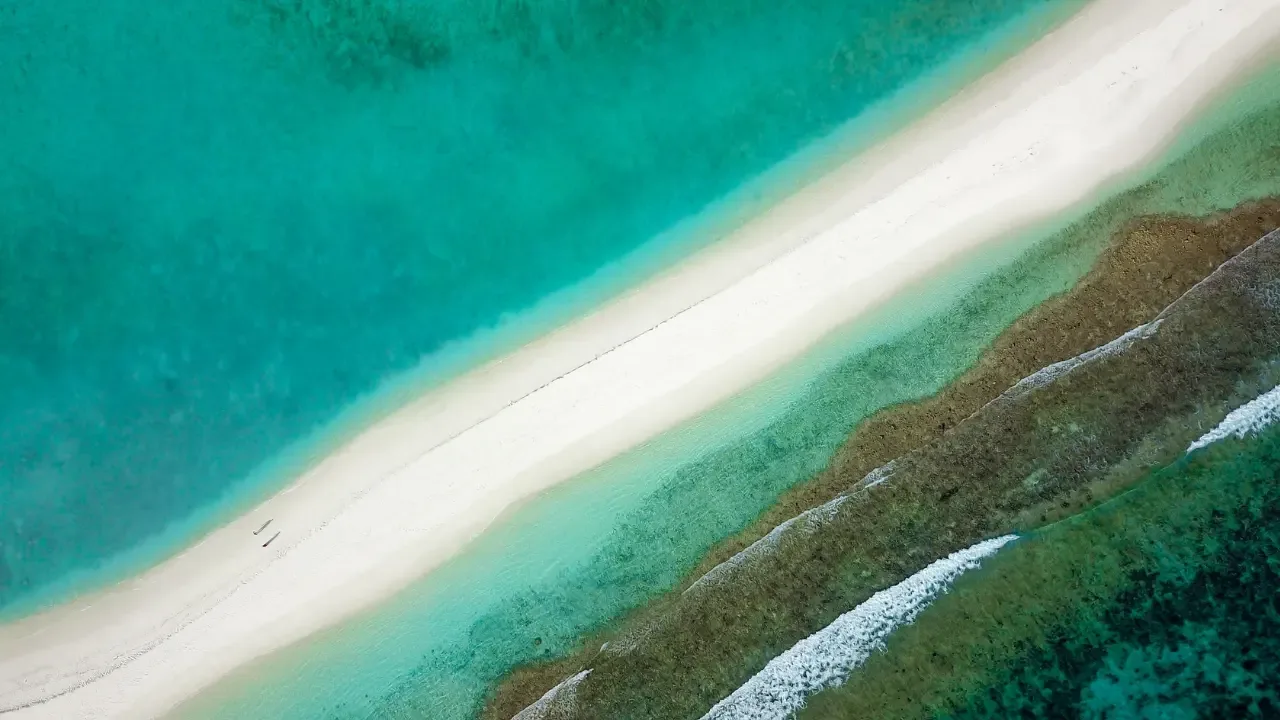
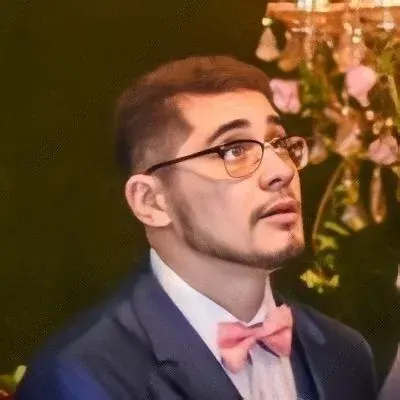
Getting a map() to return a list in Python 3.x πΊοΈπ
Hey there Pythonistas! π In this blog post, we'll be tackling a common issue when using the map()
function in Python 3.x. You might have noticed that instead of returning a list, it gives you a map
object. Don't worry, we've got you covered! π
The Problem π
Let's take a look at the code snippet that raised this question:
A: Python 2.6:
>>> map(chr, [66, 53, 0, 94])
['B', '5', '\x00', '^']
In Python 2.6, the map()
function directly returns a list with the expected output. However, in Python 3.1 and above, things have changed:
B: Python 3.1:
>>> map(chr, [66, 53, 0, 94])
<map object at 0x00AF5570>
Instead of receiving the list ['B', '5', '\x00', '^']
, we get a <map object>
representation. π©
The Solution(s) π
Solution 1: Using the list()
function β
The most straightforward solution is to convert the map
object into a list using the list()
function:
>>> list(map(chr, [66, 53, 0, 94]))
['B', '5', '\x00', '^']
By wrapping the map()
function with the list()
function, we obtain our desired list output! π
Solution 2: Using a list comprehension β β
Another elegant way of achieving the same result is by utilizing list comprehensions:
>>> [chr(item) for item in [66, 53, 0, 94]]
['B', '5', '\x00', '^']
This approach allows you to explicitly iterate over each item in the input list and apply the chr()
function to convert them individually.
Is there a better way? π€
Absolutely! If your goal is to convert a list of integers to their corresponding hexadecimal representations, you can leverage the hex()
function:
>>> [hex(item) for item in [66, 53, 0, 94]]
['0x42', '0x35', '0x0', '0x5e']
The hex()
function converts an integer to its hexadecimal representation, returning a string prefixed with '0x'
.
Conclusion and Call-to-Action π
We hope this guide helped you understand how to get a map()
function to return a list in Python 3.x! Remember, you can use either the list()
function or list comprehensions to achieve your desired output. And if you wish to convert integers to hexadecimal values, the hex()
function has got your back. πͺ
If you have any questions or want to share your own tips and tricks, leave a comment below! Let's keep the Python community thriving together! ππβ¨