Get the data received in a Flask request
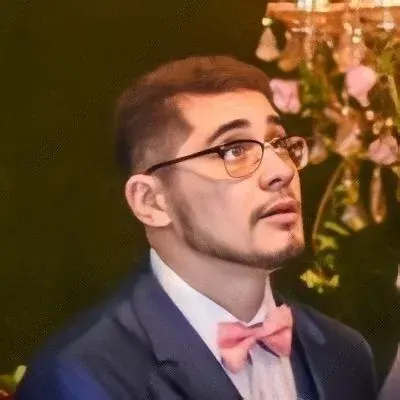
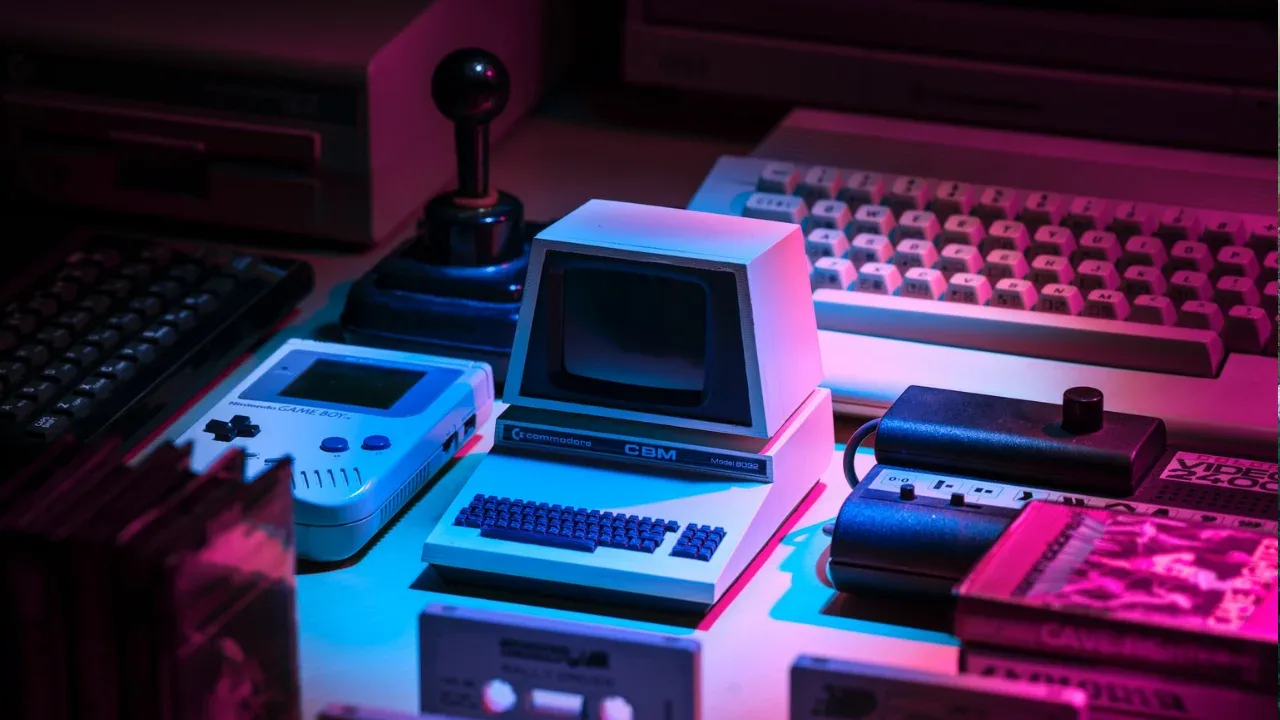
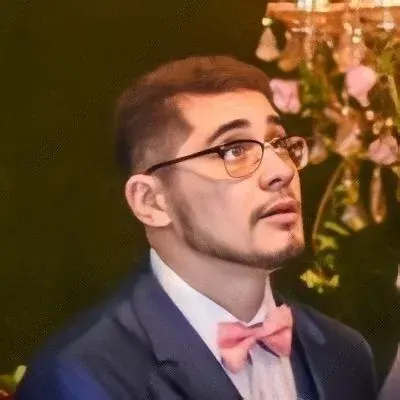
How to Get the Data Received in a Flask Request
š So, you want to be able to get the data sent to your Flask app, huh? You've landed in the right place! šÆ In this blog post, we'll address the common issue of accessing the request data in Flask and provide you with some easy solutions. So, buckle up and let's dive right in! š
š” The first thing you need to understand is that Flask provides a handy request
object to access incoming request data. However, accessing the data directly through request.data
may sometimes return an empty string, leaving you scratching your head in confusion. š¤
So, how do you access the request data properly? Here are three simple ways to get the data you need:
1. request.form
- For Form Data
š If you're dealing with form data, Flask has got your back! You can access the submitted form data using the request.form
object. It automatically parses the data for you, making it super easy to work with.
Here's an example:
from flask import request
@app.route('/', methods=['POST'])
def parse_form_data():
data = request.form
# Do something with the data
In this example, the request.form
object gives you a dictionary-like structure where you can access the individual form fields using their names as keys. š
2. request.get_json()
- For JSON Data
š¤ JSON is a popular data format, especially when working with APIs. If you're expecting JSON data in your Flask request, fret not! Flask provides a built-in method called get_json()
to extract the JSON data effortlessly.
Take a look at this example:
from flask import request
@app.route('/', methods=['POST'])
def parse_json_data():
data = request.get_json()
# Work with the JSON data
In this snippet, request.get_json()
automatically parses the incoming JSON data and returns it as a Python dictionary. You can then manipulate the data as required š ļø.
3. request.data
- For Raw Data
š Sometimes, you might need to access the raw data of the request, regardless of the Content-Type header. To get your hands on the raw data, you can use the request.data
attribute. But remember, this will give you the data as a bytestring, and you'll need to handle it accordingly.
Here's an example:
from flask import request
@app.route('/', methods=['POST'])
def parse_raw_data():
data = request.data.decode('utf-8')
# Handle the raw data
In this snippet, we're using the decode()
method to convert the bytestring into a UTF-8 string. You may need to use a different encoding depending on your specific use case.
šŖ Armed with these three approaches, you can now confidently handle different types of data in your Flask requests! But wait, there's more! š
š BONUS TIP: In addition to accessing the data, Flask's request
object offers other helpful attributes like request.headers
to access request headers, and request.method
to get the request method (e.g., GET, POST). Exploring these attributes can provide valuable insights into your requests and enhance your Flask app's functionality. š
š If you want to dig deeper into this topic or have other Flask-related questions, I recommend checking out the Stack Overflow thread titled Get raw POST body in Python Flask regardless of Content-Type header. It provides relevant insights into handling raw POST data.
⨠Now that you've learned different ways to access request data in Flask, it's time to implement them in your own project! š Remember, practice makes perfect, so go ahead and give it a try! And if you have any questions or suggestions, I'd love to hear from you in the comments section below. Let's keep the conversation going! š