Get statistics for each group (such as count, mean, etc) using pandas GroupBy?
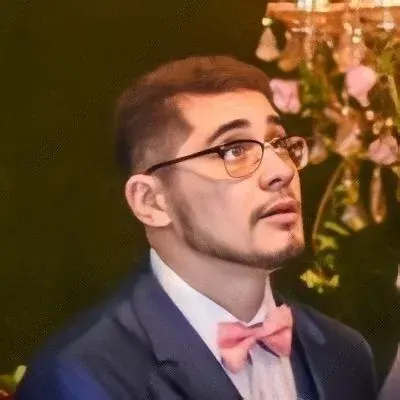
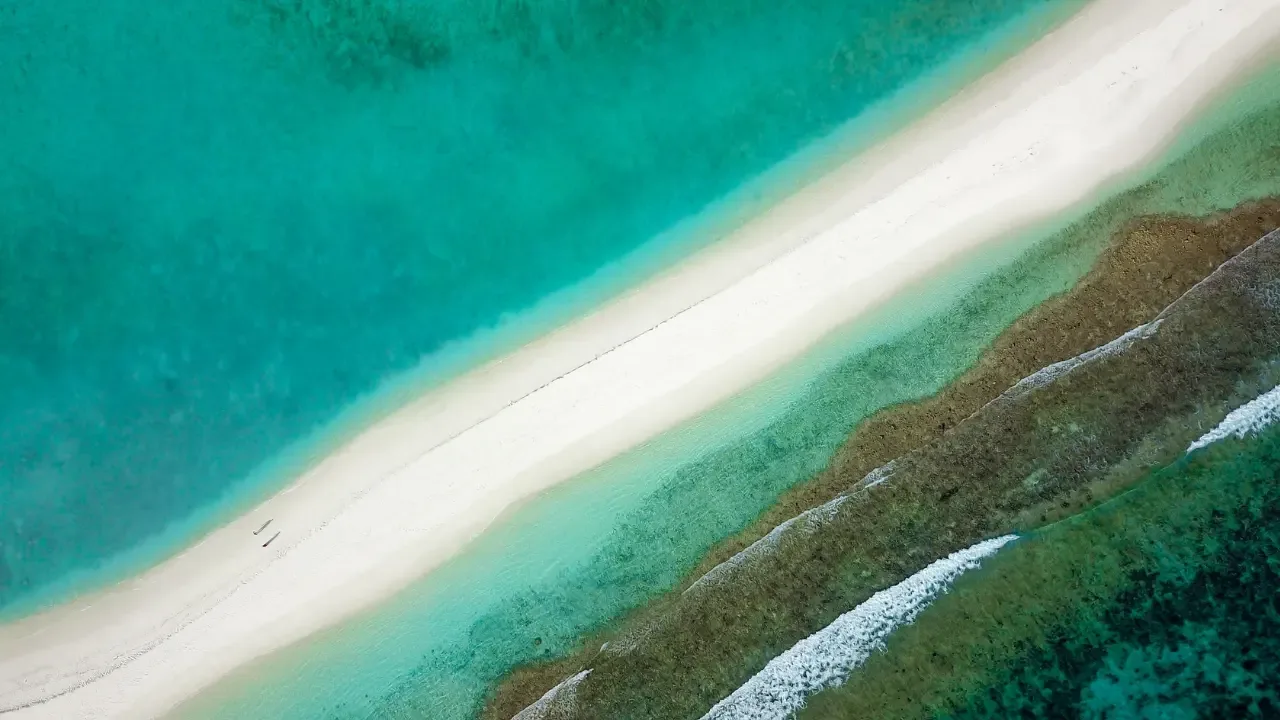
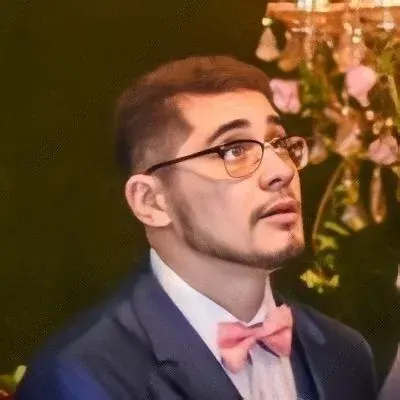
Get Group-Wise Statistics for a Dataframe using Pandas GroupBy π
Are you looking to get statistics for each group in your dataframe using the powerful GroupBy
function in Pandas? Do you want to calculate metrics like count, mean, and more, while also determining the number of rows in each group? You're in the right place! In this guide, I will show you how to accomplish this task effortlessly. Let's dive in! πͺ
The Challenge: Missing Group-Wise Row Count π§©
Imagine you have a dataframe called df
that contains several columns, including col1
, col2
, col3
, and col4
. You want to group your data by the columns col1
and col2
and calculate the mean for each group. However, you also desire an additional column that displays the count of rows for each group. The mean alone is not enough; you want to see how many values were used to compute these means for each group.
The Solution: Adding Row Count to Group-Wise Statistics π―
To achieve your desired result, you can modify your existing code by incorporating the count()
function from the Pandas library. Here's an updated version of your code:
grouped_df = df.groupby(['col1','col2']).agg(['mean', 'count'])
In the modified code snippet, we employ the agg()
function along with the mean
and count
operations. This allows us to calculate both the mean and the count for each group in a single line of code! π
The resulting grouped_df
dataframe will contain two columns for each of the grouped columns: one with the means and one with the counts. You can easily access these columns using grouped_df['col_name']
notation.
Example: Putting it all Together π
To illustrate the solution, let's consider the following example:
import pandas as pd
# Create sample dataframe
data = {'col1': ['A', 'A', 'B', 'B', 'B', 'C'],
'col2': [1, 1, 2, 2, 2, 3],
'col3': [10, 20, 30, 40, 50, 60],
'col4': [0.1, 0.2, 0.3, 0.4, 0.5, 0.6]}
df = pd.DataFrame(data)
# Group by col1 and col2, calculate mean and count
grouped_df = df.groupby(['col1','col2']).agg(['mean', 'count'])
print(grouped_df)
Output:
col3 col4
mean count mean count
col1 col2
A 1 15 2 0.15 2
B 2 40 3 0.40 3
C 3 60 1 0.60 1
In this example, we grouped the dataframe df
by col1
and col2
, and then calculated the mean and count for all the other columns. As you can see from the output, the resulting grouped_df
dataframe displays the mean and count for col3
and col4
. It provides the essential information we need for each group!
Your Turn: Try it Out! π
Now that you know how to obtain group-wise statistics using Pandas GroupBy, why not apply this technique to your own datasets? Experiment with different groupings and columns to explore the insights hidden within your data. Don't forget to include the row count using the agg()
function with the count
operation!
Feel free to share your experience, ask questions, or provide feedback in the comments below. I would love to hear about your adventures with Pandas! π
Keep coding and keep exploring! Happy data manipulation with Pandas! πΌπ»
Note: Don't forget to install the latest version of Pandas if you haven't already done so: pip install pandas
.
References
Pandas GroupBy Documentation: https://pandas.pydata.org/pandas-docs/stable/reference/api/pandas.DataFrame.groupby.html
Pandas agg() Documentation: https://pandas.pydata.org/pandas-docs/stable/reference/api/pandas.core.groupby.DataFrameGroupBy.agg.html
Image Source: https://www.pexels.com/photo/person-holding-data-table-3560431/