Get list from pandas dataframe column or row?
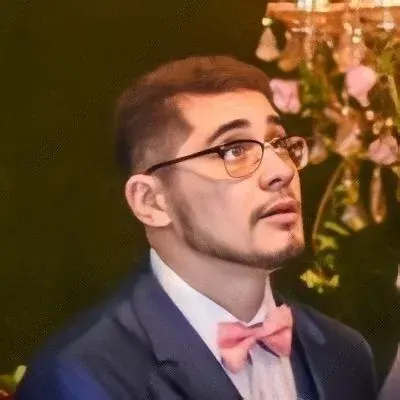
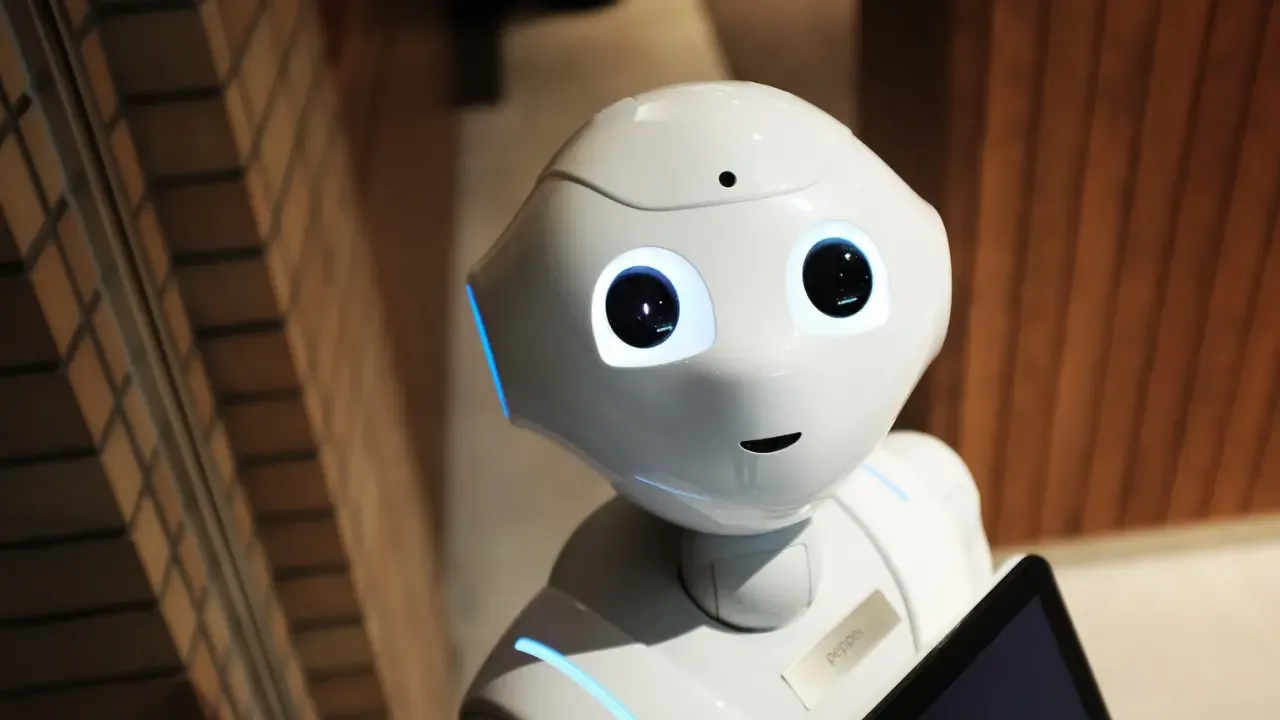
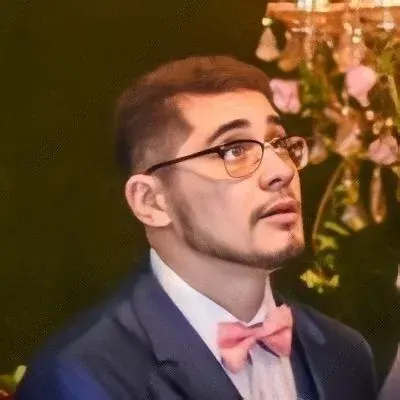
Get List from Pandas DataFrame Column or Row
Are you struggling to extract a list from a column or row in a Pandas DataFrame? ๐ Don't worry, we've got you covered! In this guide, we'll explore common issues and provide easy solutions that will allow you to retrieve the contents of a column or row as a list. Let's dive in! ๐ช
The Problem
Let's start by understanding the problem scenario. You have a DataFrame called df
imported from an Excel document with the following structure:
cluster load_date budget actual fixed_price
A 1/1/2014 1000 4000 Y
A 2/1/2014 12000 10000 Y
A 3/1/2014 36000 2000 Y
B 4/1/2014 15000 10000 N
B 4/1/2014 12000 11500 N
B 4/1/2014 90000 11000 N
C 7/1/2014 22000 18000 N
C 8/1/2014 30000 28960 N
C 9/1/2014 53000 51200 N
Your goal is to extract the contents of the 'cluster'
column as a list so that you can use it in a for-loop to create an Excel worksheet for every cluster. Additionally, you want to know if it is possible to extract the contents of a whole column or row to a list.
The Solutions
Solution 1: Extract a Column as a List
To extract a column as a list in Pandas, you can simply use the indexing syntax with the column name. In this case, you can use df['cluster']
to retrieve the 'cluster'
column as a list. Here's an example:
cluster_list = df['cluster'].tolist()
Now, you can use the cluster_list
in your for-loop to create worksheets for each cluster.
Solution 2: Extract a Row as a List
If you want to extract the contents of a specific row as a list, you can use the index-based .iloc
accessor. Here's an example of extracting the first row as a list:
row_list = df.iloc[0].tolist()
In this case, df.iloc[0]
selects the first row, and tolist()
converts it to a list.
Wrapping Up
Congratulations! Now you know how to extract a list from a column or row in a Pandas DataFrame. ๐ We covered two simple solutions:
Extracting a column as a list using
df['column_name'].tolist()
.Extracting a row as a list using
df.iloc[row_index].tolist()
.
Feel free to experiment with these solutions and adapt them to your specific needs ๐งช. If you have any questions or face any issues, let us know in the comments below. Happy coding! ๐ป
*[Excel]: Microsoft Excel