Get difference between two lists with Unique Entries
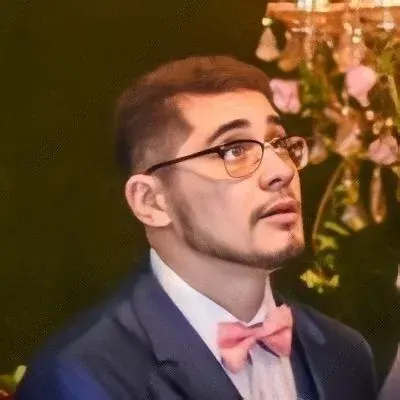
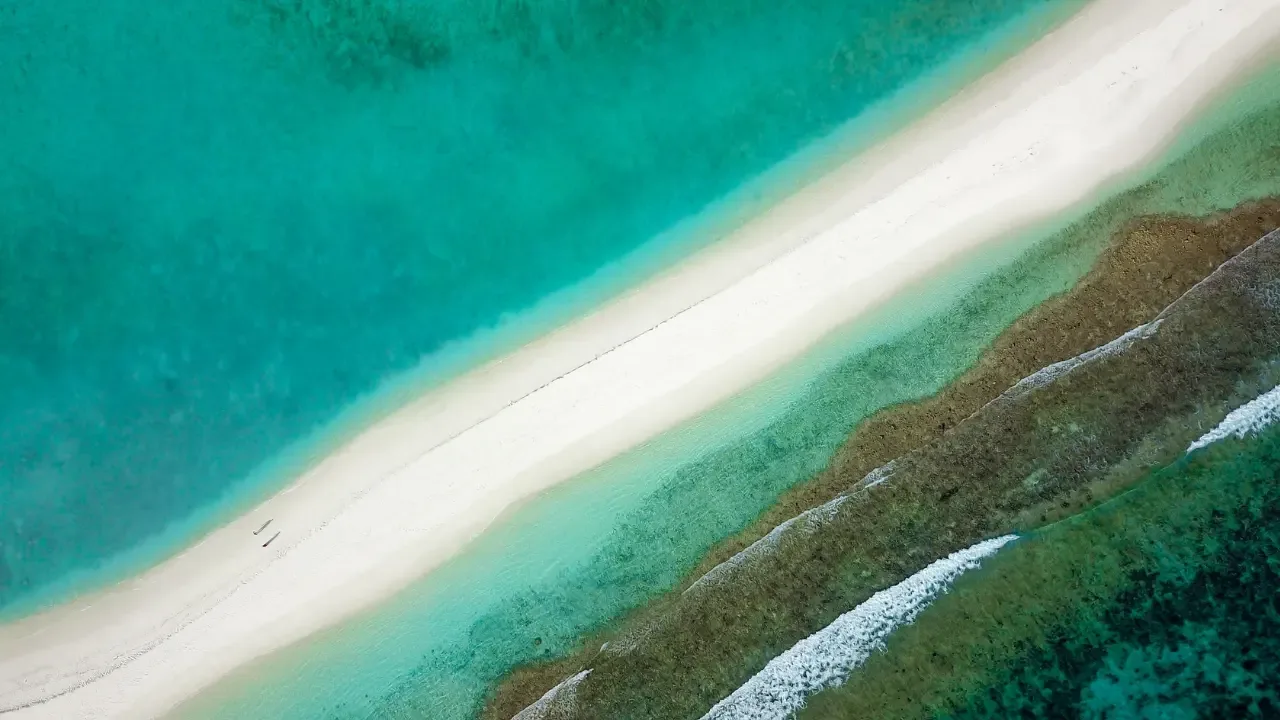
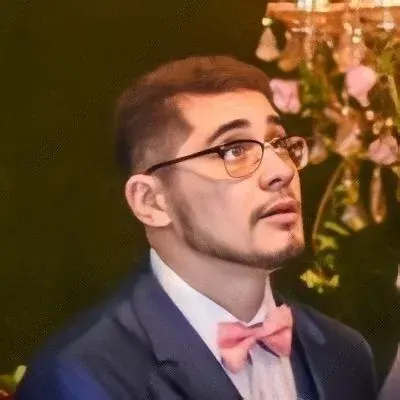
How to Get the Difference Between Two Lists with Unique Entries in Python 😎
Are you looking for a fast and efficient way to find the unique entries in one list that are not present in another list? Look no further! In this blog post, we'll explore an easy solution to this problem using Python.
The Problem 🤔
Let's say you have two lists in Python:
temp1 = ['One', 'Two', 'Three', 'Four']
temp2 = ['One', 'Two']
You want to create a third list, temp3
, that contains all the elements from temp1
which are not present in temp2
. In this case, the desired result would be:
temp3 = ['Three', 'Four']
You might be wondering if there's a way to achieve this without using cycles or explicit checking. Well, buckle up, because there is!
The Solution 🚀
Python offers a simple solution using the set
data structure and the subtraction operation. Here's how you can do it:
temp3 = list(set(temp1) - set(temp2))
Let's break down how this works:
We convert both
temp1
andtemp2
to sets using theset()
function. A set is an unordered collection of unique elements.We perform the subtraction operation
-
between the two sets. This operation returns a new set containing the elements that are in the first set but not in the second set.Finally, we convert the resulting set back to a list using the
list()
function to obtain the desiredtemp3
.
That's it! With just one line of code, you can easily get the unique entries from temp1
that do not appear in temp2
.
Example 🌟
To further clarify the solution, let's see an example:
temp1 = ['One', 'Two', 'Three', 'Four']
temp2 = ['One', 'Two']
temp3 = list(set(temp1) - set(temp2))
print(temp3) # Output: ['Three', 'Four']
As you can see, the elements 'Three'
and 'Four'
in temp1
are not present in temp2
and are successfully captured in temp3
.
Conclusion & Call-to-Action 📝
Finding the difference between two lists with unique entries in Python can be as simple as using sets and the subtraction operation. By following the solution provided in this blog post, you can efficiently obtain the desired results without cumbersome cycles or explicit checking.
So why wait? Give it a try and let us know how it goes! Do you have any other Python problems you'd like us to tackle? Feel free to reach out and share your thoughts in the comments below. Happy coding! 💻🐍