Get column index from column name in python pandas
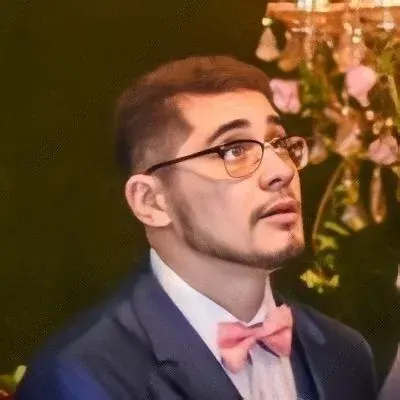
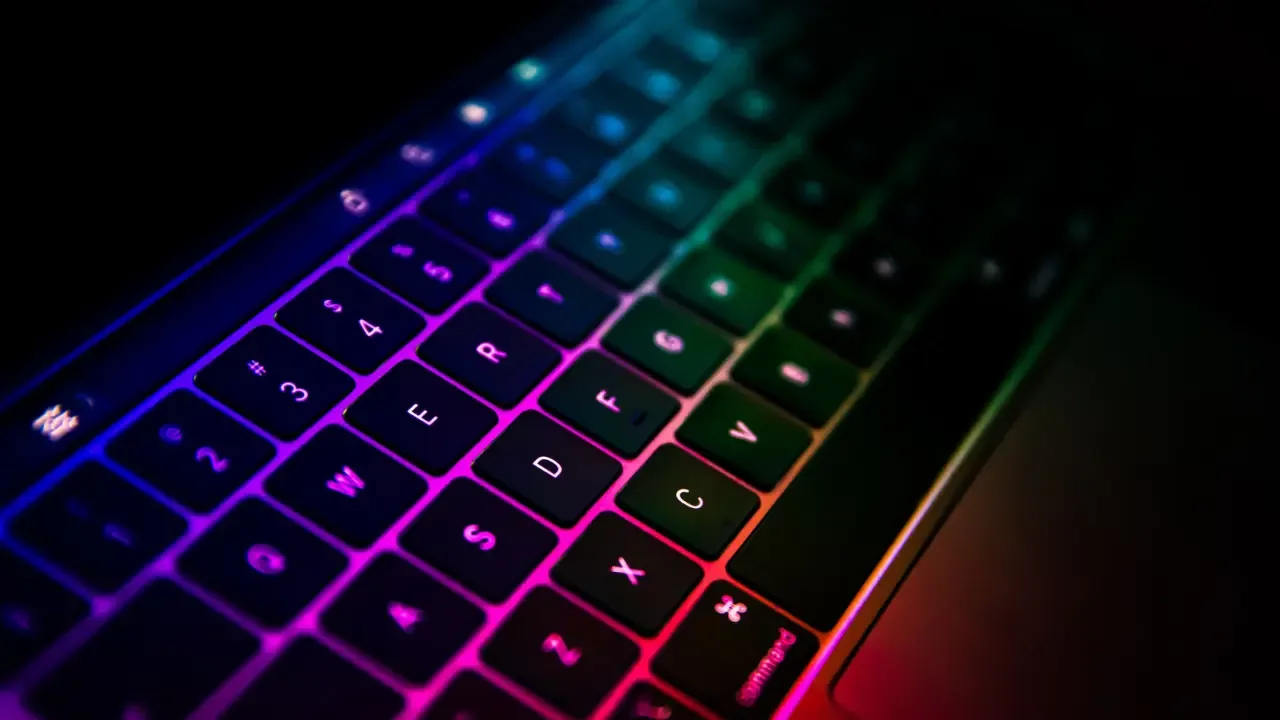
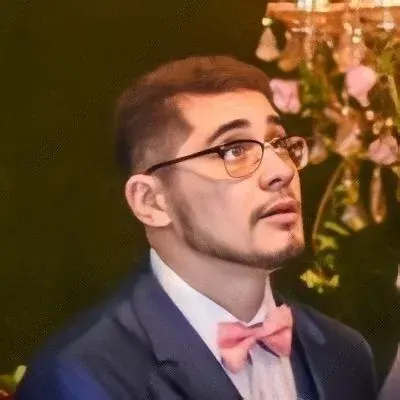
How to Get Column Index from Column Name in Python Pandas: A Beginner's Guide 💻🐼
Have you ever found yourself working with a large dataset in Python's Pandas library and needing to retrieve the index of a column based on its name? It can be quite a daunting task, especially if you're new to Python or data manipulation. But fear not! In this article, we'll explore different ways to solve this problem and provide you with easy-to-follow solutions. So let's dive right in! 🏊♂️
Before we proceed, let's set the context. In R, if you want to retrieve a column index based on its name, you can simply use the which()
function along with names()
:
idx <- which(names(my_data) == my_column_name)
But what about Python Pandas? Is there a similar functionality available? The short answer is YES! 🎉 Let's explore a few different methods that will help you achieve the same result.
Method 1: Using the get_loc()
Function 🕵️♂️
Python Pandas provides us with a very handy function called get_loc()
that allows us to retrieve the index of a column by its name. Here's how you can use it:
import pandas as pd
# Assuming you have a DataFrame named 'df'
column_name = 'my_column_name'
column_index = df.columns.get_loc(column_name)
# Voilà! You've got the index of your desired column
print(f"The index of {column_name} is {column_index}")
By using the get_loc()
function, you can easily get the index of my_column_name
. Remember to replace 'my_column_name'
with the actual name of the column you want to find.
Method 2: Converting Column Names to a List and Using index()
🔢
Another approach to finding the index of a column is by converting the column names into a list and then using the index()
method to retrieve the index of the desired column. Check out the following code snippet:
import pandas as pd
# Assuming you have a DataFrame named 'df'
column_name = 'my_column_name'
column_index = list(df.columns).index(column_name)
# Success! You've got the index of your desired column
print(f"The index of {column_name} is {column_index}")
Now, you can easily find the index of my_column_name
using the index()
method. Just replace 'my_column_name'
with your column's actual name.
Method 3: Using a Dictionary Comprehension 📚
Here's another nifty way to retrieve the index of a column using a dictionary comprehension. Take a look at the following code snippet:
import pandas as pd
# Assuming you have a DataFrame named 'df'
column_name = 'my_column_name'
column_index = {column: idx for idx, column in enumerate(df.columns)}[column_name]
# Brilliant! You've got the index of your desired column
print(f"The index of {column_name} is {column_index}")
By using this dictionary comprehension technique, you can easily map each column name to its corresponding index and retrieve the desired index efficiently.
Now that you know different ways to get the column index from the column name in Python Pandas, you can confidently manipulate your data without any struggle. But remember, practice makes perfect! So go ahead and try out these methods on your own datasets. 📊
Tell us, which method was your favorite? Did you find this article helpful? We'd love to hear your thoughts and see your code examples! Feel free to leave a comment below and keep the discussion going. Let's learn and grow together! 🌟
Happy coding! 💻✨