Get a list from Pandas DataFrame column headers
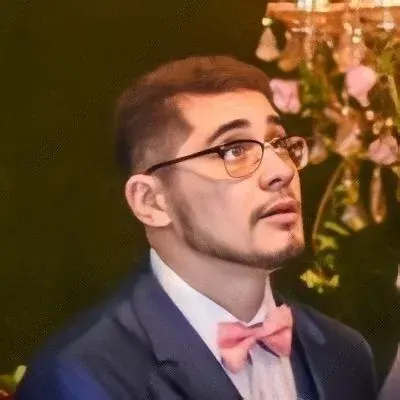
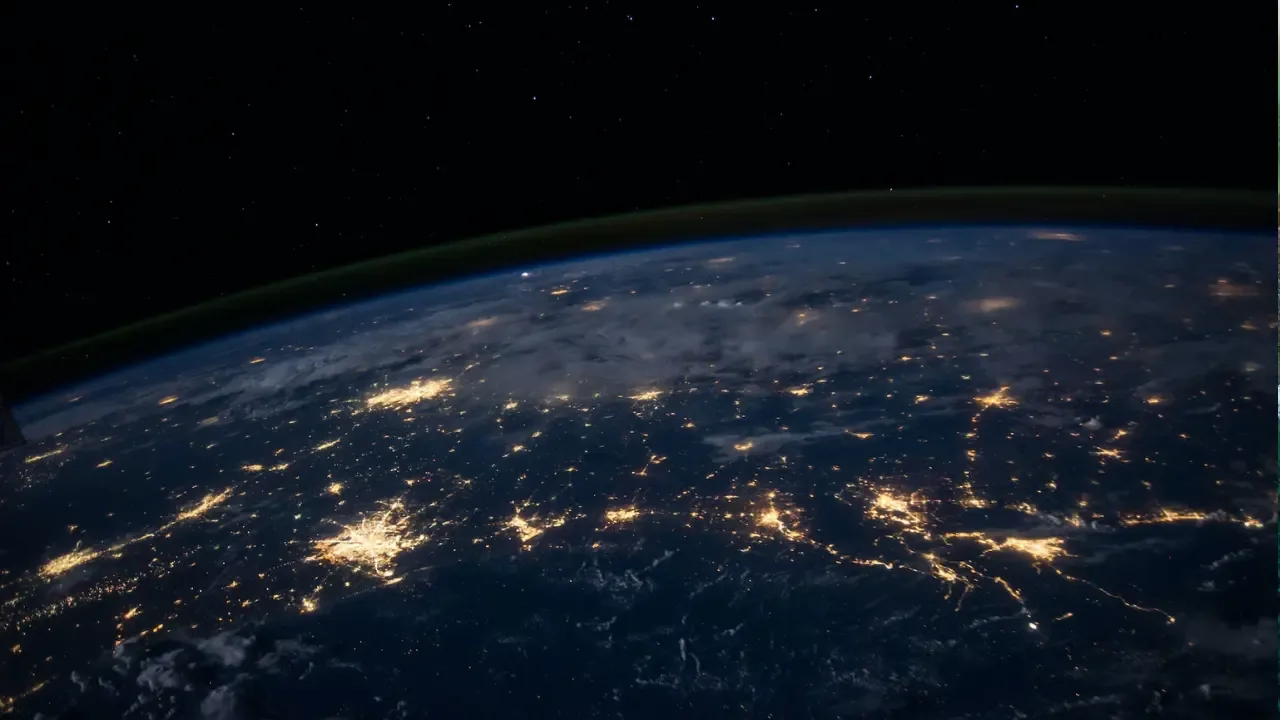
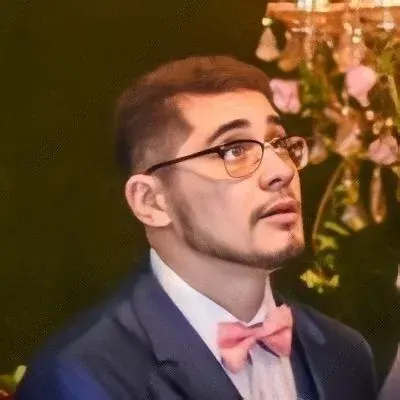
How to Get a List of Column Headers from a Pandas DataFrame 📊
So, you've got a Pandas DataFrame and you need to extract a list of its column headers? No worries, we've got you covered! In this guide, we'll go over a simple and straightforward solution to this problem. Let's dive in! 💪
The Scenario 📋
Imagine you have a Pandas DataFrame, but you don't know how many columns it contains or what their names are. Here's an example DataFrame to make things clearer:
import pandas as pd
df = pd.DataFrame({
'y': [1, 2, 8, 3, 6, 4, 8, 9, 6, 10],
'gdp': [2, 3, 7, 4, 7, 8, 2, 9, 6, 10],
'cap': [5, 9, 2, 7, 7, 3, 8, 10, 4, 7]
})
# DataFrame:
# y gdp cap
# 0 1 2 5
# 1 2 3 9
# 2 8 7 2
# 3 3 4 7
# 4 6 7 7
# 5 4 8 3
# 6 8 2 8
# 7 9 9 10
# 8 6 6 4
# 9 10 10 7
Your goal is to obtain a list containing the column headers from this DataFrame, which should look like this:
['y', 'gdp', 'cap']
The Solution 💡
To accomplish this, you can leverage a simple pandas method called columns
. This method returns an Index object with the column labels, which can be conveniently converted to a list. Here's how you can achieve it:
column_headers = df.columns.tolist()
And that's it! column_headers
will now contain the list of column headers from your DataFrame.
Putting It All Together 🤝
Let's wrap up everything we've learned in a nice little code snippet:
import pandas as pd
df = pd.DataFrame({...}) # Your DataFrame here
column_headers = df.columns.tolist()
print(column_headers)
When you run this code, the output will be the list of column headers.
In Conclusion 🎉
Obtaining a list of column headers from a Pandas DataFrame is a breeze! By using the columns
method and converting the resulting Index object to a list, we can effortlessly achieve our goal.
Now that you have this knowledge in your toolkit, you'll be able to tackle similar challenges with ease. Feel free to explore more functionalities offered by Pandas to level up your data analysis skills! 🚀
If you found this guide helpful, don't forget to share it with your fellow data enthusiasts! 📤✨