Format numbers in django templates
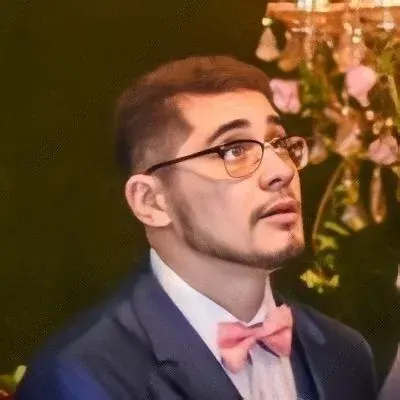
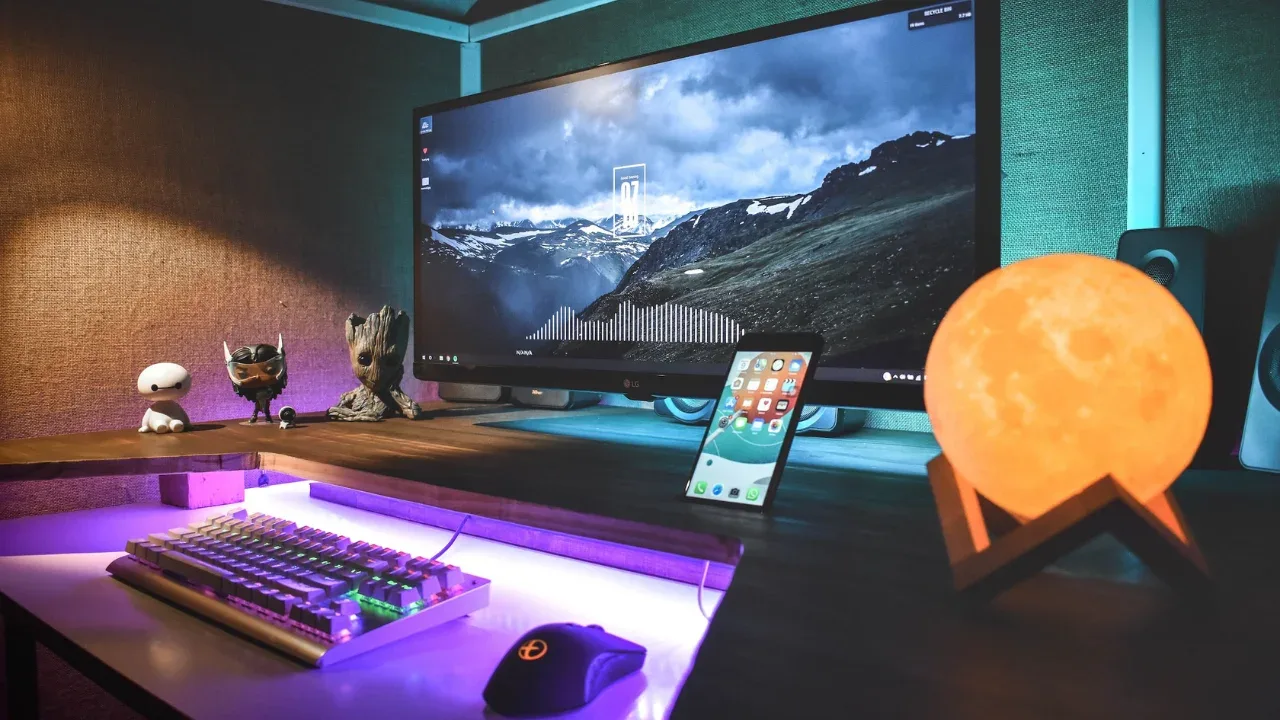
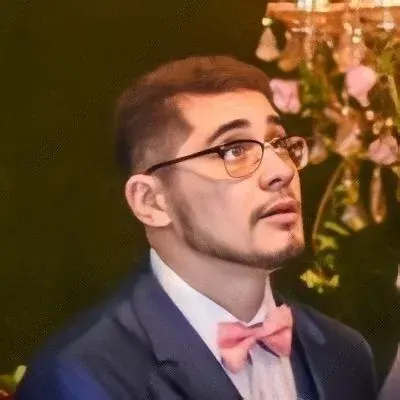
📝🤔 transforming numbers using filters in Django templates...
Oh hi there! 👋 Are you struggling to format numbers in your Django templates? I totally get it! It seems like a simple task, but can be quite confusing trying to figure out which filter to use. But fret not, my friend! In this post, I'll guide you through the process of formatting numbers like a pro. Let's dive in! 🏊♂️
So, you want to format those numbers? Let's start with some examples to understand the desired outcome:
1 => 1
12 => 12
123 => 123
1234 => 1,234
12345 => 12,345
Makes sense? Excellent. Now, let me show you two different approaches you can take to achieve this.
Option 1: Using Django's intcomma
filter
Django comes with a built-in filter called intcomma
that adds commas to numbers. It's perfect for our use case! Here's how you can utilize it:
<!-- your_template.html -->
{% load humanize %}
<p>The formatted number is: {{ your_number|intcomma }}</p>
All you need to do is load the humanize
template tag library, and then you can apply the intcomma
filter to your number. Easy-peasy, right? 😉
Option 2: Using Python's locale
module
If you prefer a more generic Python solution, you can handle the formatting directly in your model using the locale
module. Here's how:
import locale
from django.db import models
class YourModel(models.Model):
# your other fields
@property
def formatted_number(self):
locale.setlocale(locale.LC_ALL, '') # Use the system's default locale
return locale.format_string("%d", self.your_number, grouping=True)
In this approach, we import the locale
module and use the setlocale
function to set the system's default locale. Then, in the property formatted_number
, we use the format_string
method to format the number with commas.
To display the formatted number in your template, simply access the formatted_number
property of your model instance:
<!-- your_template.html -->
<p>The formatted number is: {{ your_model_instance.formatted_number }}</p>
That's it! Two simple ways to format your numbers in Django templates. Choose the option that works best for you and start using it in your project today. 🎉
But hey, don't leave just yet! I love hearing from my readers. Have you encountered any other number formatting challenges in Django? Or maybe you found a unique solution? Feel free to share your experiences and insights in the comments below. Let's grow together! 🌱💬
Happy coding! Keep those numbers formatted and your templates looking snazzy! ✨👨💻
📝 P.S. If you're enjoying this blog post and want to receive more valuable content in the future, don't forget to subscribe to my newsletter! It's a great way to stay updated on the latest tech tips and tricks. Plus, you'll become part of an awesome community of developers like yourself. Join us today! 💌💻