Finding the index of an item in a list
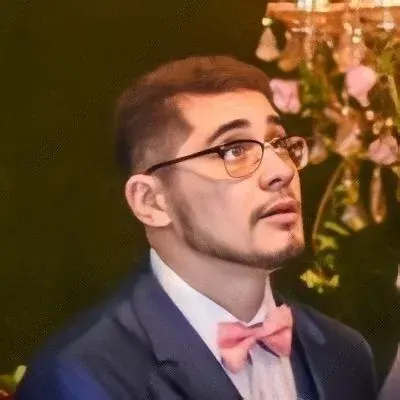
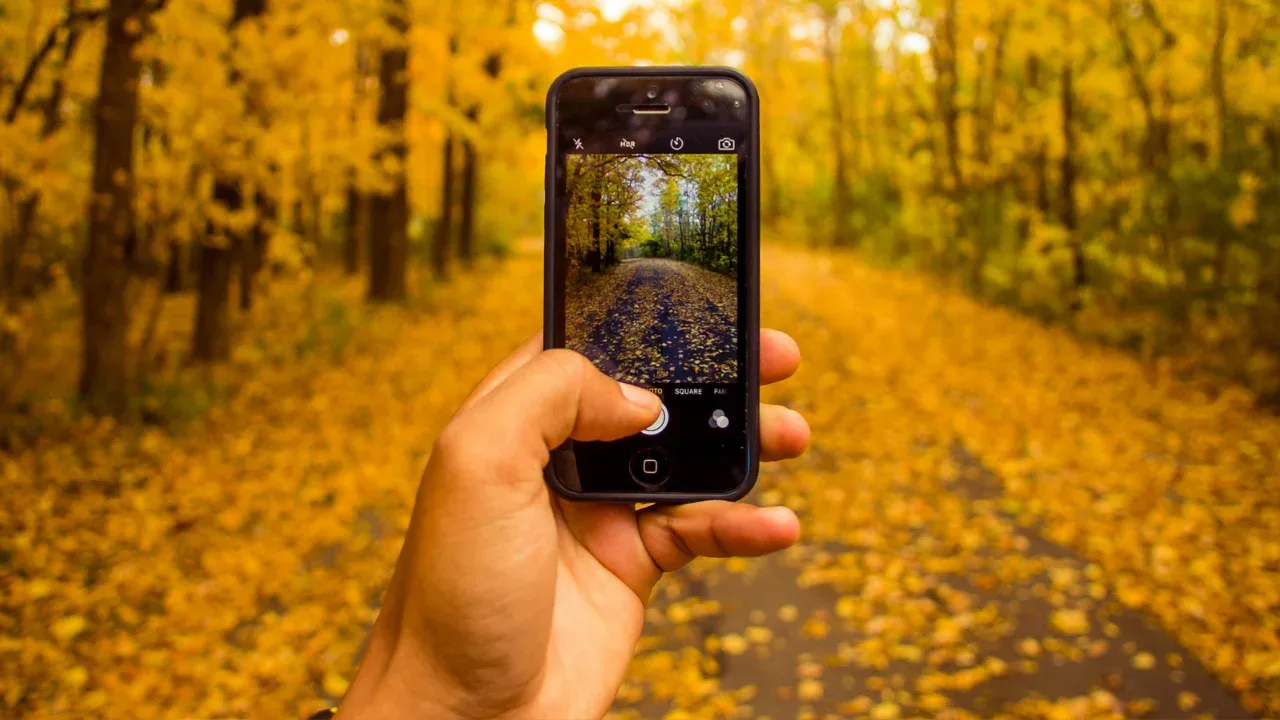
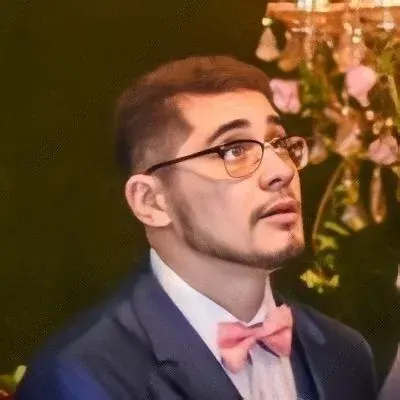
Finding the Index of an Item in a List: A Complete Guide
ššā
Have you ever found yourself in a situation where you have a list and you need to find the index of a specific item within that list? It can be a common problem, but don't worry, we've got you covered! In this blog post, we will explore this issue, provide easy and practical solutions, and ensure you leave with a clear understanding. Let's dive in! šŖ
The Problem at Hand
Let's start with a simple scenario: you have a list and you want to find the index of a specific item within that list. Consider the following example:
my_list = ["foo", "bar", "baz"]
Our mission is to find the index of the item "bar". What's the best approach? Well, let's explore some solutions!
Solution 1: Using the index
Method
Python provides a built-in method called index
which simplifies finding the index of an item in a list. Take a look at this code snippet:
my_list = ["foo", "bar", "baz"]
item = "bar"
index = my_list.index(item)
print(index)
This will output 1
, which is the index of the item "bar" in the list "my_list". Easy, right? š
However, it's worth mentioning that if the item does not exist in the list, the index
method will raise a ValueError
exception. So, keep an eye out for that!
Solution 2: Handling the Exception
To avoid potential exceptions, it's a good practice to handle them gracefully. One way to achieve this is by using a try
...except
block. Let's take a look at an example:
my_list = ["foo", "bar", "baz"]
item = "qux" # Not in the list
try:
index = my_list.index(item)
print(index)
except ValueError:
print("Item not found in the list.")
In this case, since "qux" does not exist in the list, the code will print "Item not found in the list." This way, you can handle the exception and ensure your code continues running without interruptions. š
Solution 3: Handling Multiple Occurrences
What if you have duplicates in your list and want to find the index of the first occurrence or all occurrences? Don't worry, we've got you covered!
Finding the Index of the First Occurrence
To find the index of the first occurrence of an item, you can simply use the index
method we discussed earlier. Here's an example:
my_list = ["foo", "bar", "baz", "bar"]
item = "bar"
index = my_list.index(item)
print(index)
The output will be 1
, which is the index of the first occurrence of "bar".
Finding the Index of All Occurrences
If you want to find the index of all occurrences of an item in a list, you can use a list comprehension along with the enumerate
function. Let me show you an example:
my_list = ["foo", "bar", "baz", "bar"]
item = "bar"
indexes = [index for index, value in enumerate(my_list) if value == item]
print(indexes)
This will output [1, 3]
, which are the indexes of both occurrences of "bar" in the list.
Conclusion and Call-to-Action
Finding the index of an item in a list is a seemingly simple task, but it can come with its own set of challenges. In this blog post, we explored different solutions to tackle this problem and learned about handling exceptions, finding the index of the first occurrence, and even finding the index of all occurrences.
Now that you have a solid understanding of how to find the index of an item in a list, it's time to put it into practice! šØš”
Think of scenarios where this knowledge can be applied and try it out in your own projects. And remember, sharing is caring! If you found this blog post helpful, don't hesitate to share it with your fellow developers.
Happy coding! šš„
Feel free to leave a comment below, sharing your thoughts or asking any questions you might have! šš