Find the unique values in a column and then sort them
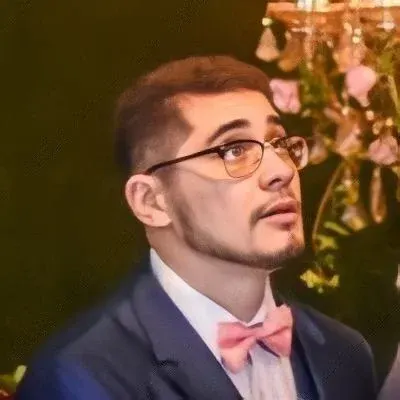
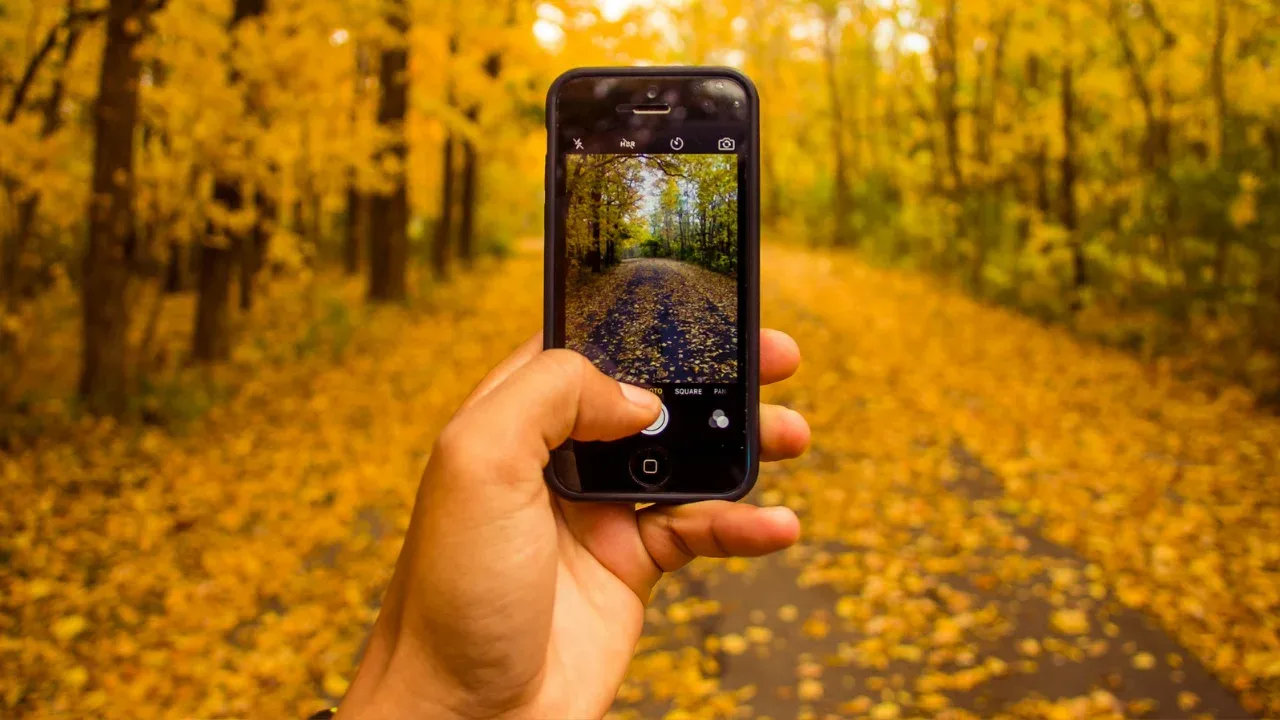
🎉 Finding Unique Values and Sorting Them in a Pandas DataFrame 🎉
So you have a pandas dataframe and you want to find the unique values in one of its columns and then sort them in ascending order. Sounds like a simple task, right? But wait, you tried it and got an unexpected None
as the output. Don't worry, we've got you covered! In this blog post, we'll walk you through common issues that can arise when trying to find unique values and sort them in pandas, and we'll provide easy solutions to ensure you get the correct results. Let's dive in! 💪
The Problem 😱
Let's start by taking a look at the code you provided:
import pandas as pd
df = pd.DataFrame({'A':[1,1,3,2,6,2,8]})
a = df['A'].unique()
print(a.sort())
You expected to get the unique values in column 'A', sorted in ascending order. However, you ended up with a frustrating None
as the output. What went wrong? Let's analyze it step by step. 🕵️♀️
Understanding the Issue 🤔
The problem lies in the usage of the .sort()
method. The sort()
method doesn't return a sorted array; instead, it sorts the array in place and returns None
. So when you do a.sort()
, it sorts the array a
and the return value will be None
, which is why you see None
printed on the screen. To fix this, we'll need to use a different approach.
Solution 1: Using the sorted()
Function 📝
One simple solution is to use the built-in sorted()
function to sort the unique values. Here's the modified code:
import pandas as pd
df = pd.DataFrame({'A':[1,1,3,2,6,2,8]})
a = sorted(df['A'].unique())
print(a)
By using sorted()
, the unique values in column 'A' will be sorted and assigned to the variable a
. Then, by printing a
, you will see the sorted unique values as expected. 🎉
Solution 2: Sorting the Dataframe Directly with .sort_values()
🔄
Another approach is to sort the dataframe itself using the .sort_values()
method. This way, you can get the sorted unique values directly from the dataframe without using the unique()
function. Here's how you can do it:
import pandas as pd
df = pd.DataFrame({'A':[1,1,3,2,6,2,8]})
df_sorted = df['A'].sort_values().unique()
print(df_sorted)
By chaining the .sort_values()
method to the column 'A', you can sort the dataframe and then use .unique()
to get the unique values. Finally, print df_sorted
to see the sorted unique values. 🥳
Conclusion and Call-to-Action 🏁
Finding unique values and sorting them in pandas can be a bit tricky, especially if you're not familiar with the specific methods involved. But fear not! Now you know how to overcome the issue you faced and get the desired results.
Want to explore more about pandas or any other exciting tech topics? Be sure to check out our blog for more helpful guides and engaging content. Also, share this post with your fellow tech enthusiasts and let us know your thoughts in the comments below. Happy coding! ✨🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
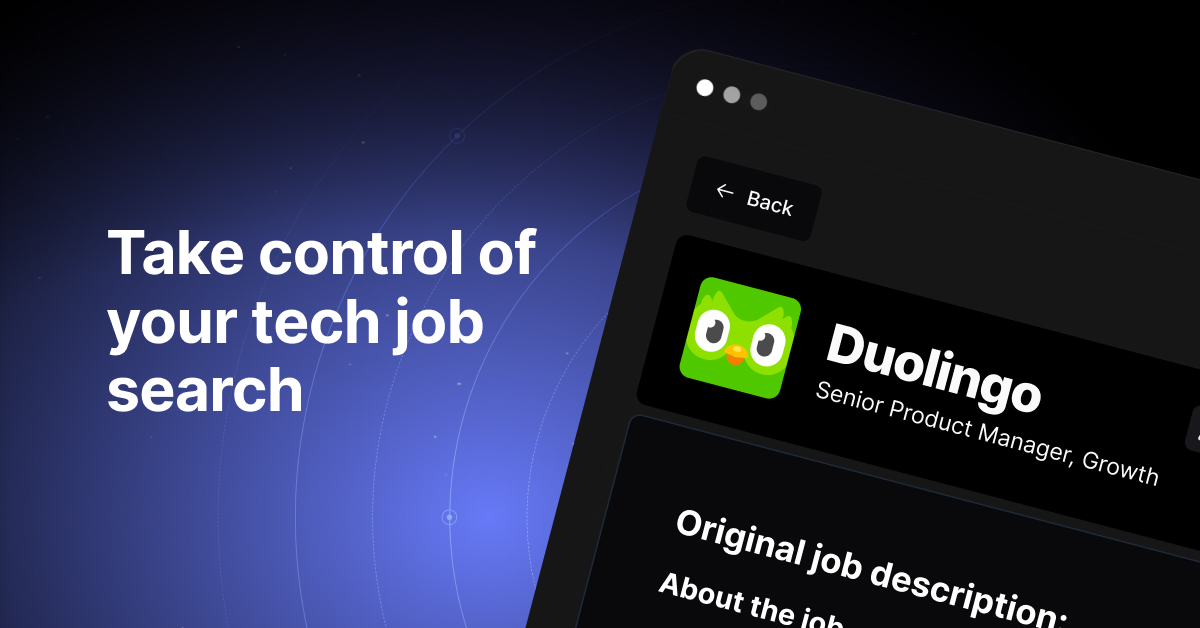