Find the current directory and file"s directory
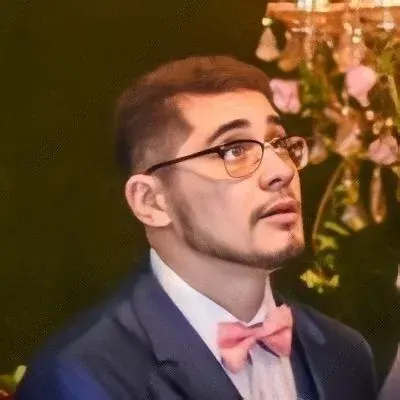
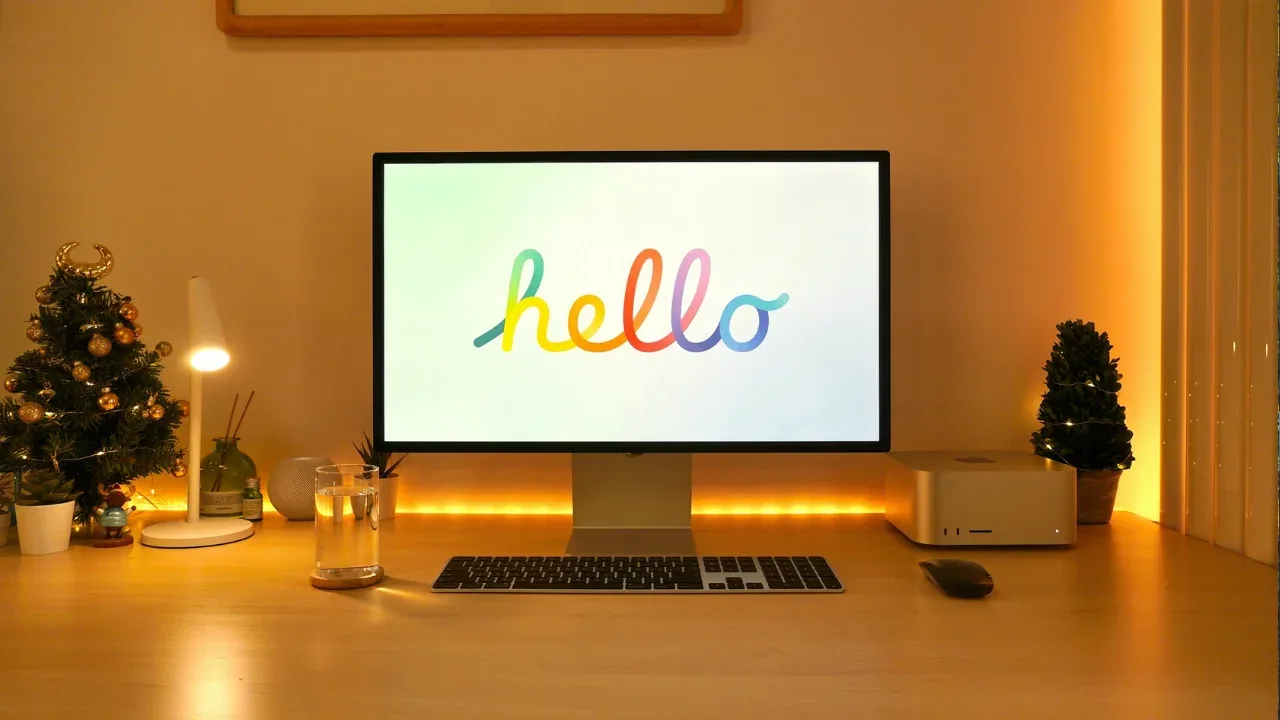
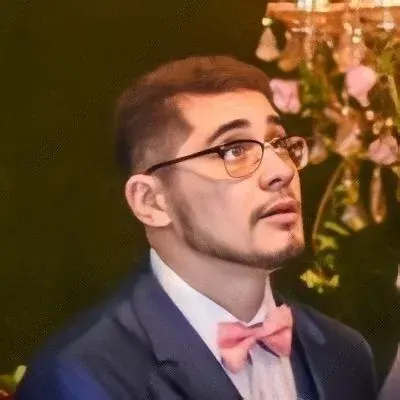
Finding the Current Directory and File's Directory: A Guide for Python Developers
Hey there, fellow Python developers! 🐍 Have you ever found yourself wondering about the current directory and file's directory when running a Python script? I know I have! 🤔
In this blog post, we'll tackle this common issue and provide easy solutions to help you find the answers you're looking for. So let's dive right in and get to the bottom of it! 💻
Understanding the Problem
When running a Python script, it's often helpful to know the current directory (where we were in the shell when we ran the script) and the directory where the Python file we're executing is located. This information can be crucial for tasks like reading/writing files, importing modules, or working with relative file paths.
Solution 1: The os
Module
One way to obtain the current directory in a Python script is by using the os
module. This module provides a wide range of functions for interacting with the operating system, including retrieving directory-related information.
To obtain the current directory, you can use the getcwd()
function from the os
module. It returns a string representing the current working directory.
import os
current_directory = os.getcwd()
print("Current Directory:", current_directory)
Running this code will display the current directory in the console. 📂
Solution 2: The __file__
Attribute
To determine the directory where the Python file you're executing is located, you can make use of the __file__
attribute, which contains the script's file path.
import os
script_path = os.path.dirname(os.path.realpath(__file__))
print("File Directory:", script_path)
Executing this code will print the directory of the current script. 📄
Dealing with Common Issues
Issue 1: Differentiating between absolute and relative paths
When using the os
module's functions, it's important to understand the distinction between absolute and relative paths.
Absolute paths begin with the root directory (e.g.,
/usr/local/bin
on Unix-like systems orC:\Program Files
on Windows).Relative paths are specified relative to the current directory.
Make sure you're using the appropriate path based on your requirements. 🛤️
Issue 2: Working with scripts in different directories
If your Python script is located in a different directory than the one you're executing it from, Solution 2 may yield unexpected results. In such cases, it's advisable to use Solution 1 to find the current directory.
Call-to-Action: Engage with our Community!
Now that you have a better understanding of how to find the current directory and the directory of the executing Python file, why not share your thoughts with our community? We'd love to hear your experiences, tips, and tricks! 💬
Join us on Twitter using the hashtag #PythonDirectoryGuide and let's continue the conversation. Together, we can make Python development even more fun and productive! 🚀
Happy coding! 😄