Find row where values for column is maximal in a pandas DataFrame
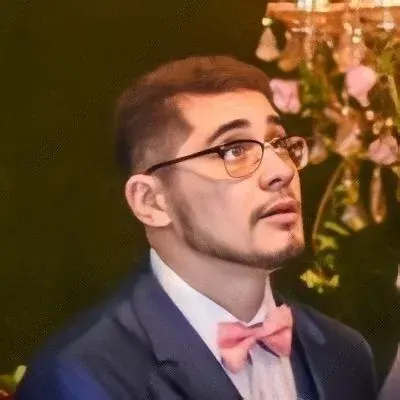
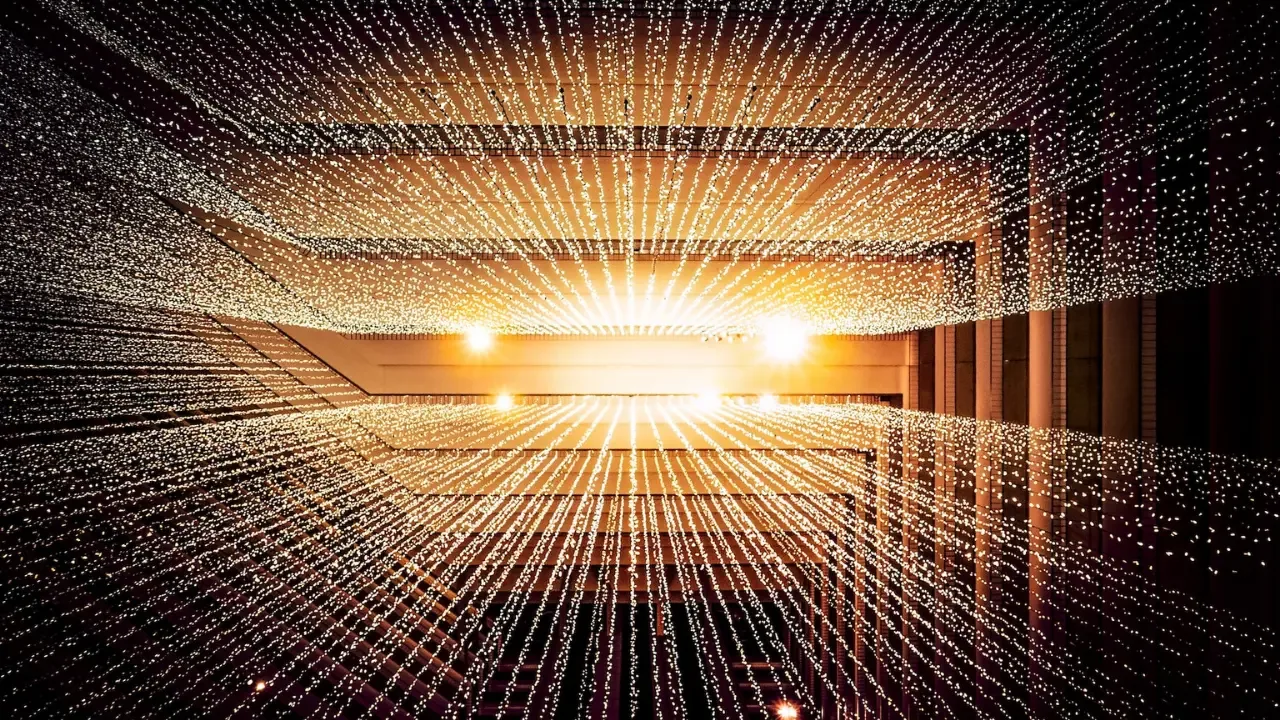
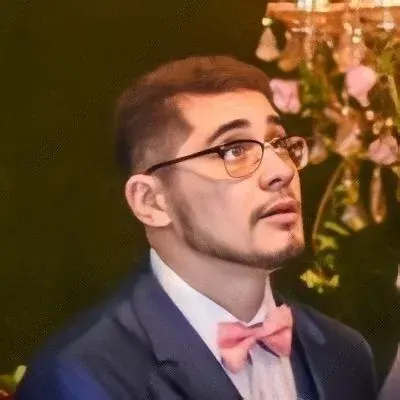
Finding the Row with the Maximal Value in a Pandas DataFrame
If you are working with a Pandas DataFrame in Python, you may come across a situation where you need to find the row that contains the maximum value in a specific column. This can be a common task when analyzing data, but it can sometimes be tricky to figure out the best approach.
To set the context, let's consider the following scenario:
import pandas as pd
# Creating a sample DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David'],
'Age': [25, 30, 35, 40],
'Score': [95.5, 80.2, 87.8, 92.3]}
df = pd.DataFrame(data)
In this example, we have a DataFrame with three columns: 'Name', 'Age', and 'Score'. Now, let's say we want to find the row that has the maximum value in the 'Score' column.
Solution 1: Using idxmax()
One way to solve this problem is by using the idxmax()
function. This function returns the index of the maximum value in a given Series or DataFrame column.
To find the row with the maximum value in the 'Score' column, we can use the following code:
max_index = df['Score'].idxmax()
max_row = df.loc[max_index]
Here, max_index
will store the index of the row that contains the maximum value in the 'Score' column. By using df.loc[max_index]
, we can retrieve the entire row corresponding to this index.
Let's print the result to see the output:
print(max_row)
Output:
Name Alice
Age 25
Score 95.5
Name: 0, dtype: object
As you can see, the code correctly identifies that the row with the maximum score belongs to Alice. Now you can apply the same approach to any other column in your DataFrame.
Solution 2: Using Boolean Indexing
Another method to find the row with the maximum value in a column is by using Boolean indexing. This technique allows you to filter the DataFrame based on a specific condition.
To find the row with the maximum value in the 'Score' column using Boolean indexing, you can follow these steps:
Create a boolean mask by comparing each value in the 'Score' column with the maximum value.
Use this mask to filter the DataFrame and retrieve the desired row.
mask = df['Score'] == df['Score'].max()
max_row = df[mask]
Here, mask
is a Boolean Series where each value is True
if the corresponding value in the 'Score' column is equal to the maximum value, and False
otherwise. By using this mask with df[mask]
, we can select the rows that satisfy the condition.
Printing the result will give you the same output as before.
Conclusion
Finding the row that contains the maximum value in a specific column of a Pandas DataFrame is a common task when working with data. In this blog post, we explored two simple yet effective solutions to accomplish this: using idxmax()
and Boolean indexing.
Now that you have learned these techniques, you can easily apply them to your own data analysis tasks. So go ahead and give them a try!
Feel free to share any other methods or ask any further questions in the comments below. Happy coding! 👩💻🚀
Do you have a specific DataFrame problem you need help with? Let us know in the comments, and we'll be happy to assist you!
Image Source: Unsplash.com